C# 有效地刪除字串中的所有空格
Minahil Noor
2024年2月16日
Csharp
Csharp String
-
C# 使用
Regex.Replace()
方法有效地刪除字串中的所有空格 -
C# 使用
String.Replace()
方法有效地從字串中刪除所有空格 -
C# 使用
Where
方法有效地刪除字串中的所有空格
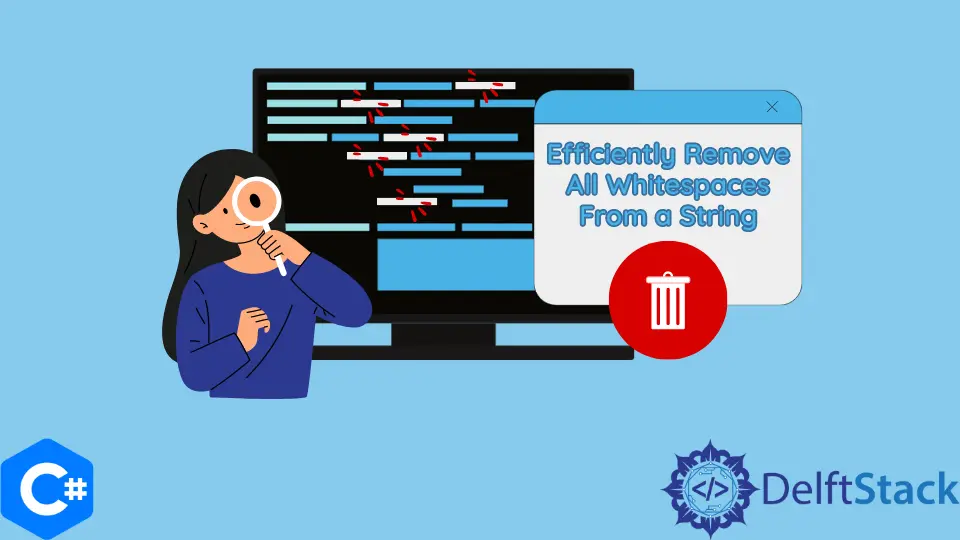
在 C# 中,有多種方法可以有效地刪除字串中的所有空格。在本文中,我們將討論各種方法來刪除字串中的所有空格。
C# 使用 Regex.Replace()
方法有效地刪除字串中的所有空格
正規表示式是 C# 中最有效的模式匹配功能。每個操作都有特定的模式。通過使用正規表示式,我們還可以從字串中刪除所有空格。我們將使用 Regex.Replace()
方法有效地刪除所有空格。它屬於 C# 中的 Regex
類。
使用此方法的正確語法如下:
Regex.Replace(String, @"\s", "")
這裡的 ""
代表一個空的字串。空格字元的正規表示式模式為\s
。Regex.Replace()
方法在給定的字串中找到一個空格字元,並將其替換為空的字串。
示例程式碼:
using System;
using System.Text.RegularExpressions;
namespace Example {
class RemoveAllWhitespaces {
static void Main(string[] args) {
string OldString = "This is a String.";
Console.WriteLine("The old string is: " + OldString);
string NewString = Regex.Replace(OldString, @"\s", "");
Console.WriteLine("The new string is: " + NewString);
}
}
}
輸出:
The old string is: This is a String.
The new string is: ThisisaString.
C# 使用 String.Replace()
方法有效地從字串中刪除所有空格
這是從給定的字串中刪除空格的最簡單方法。方法 Replace()
將給定的字串或 character
替換為所需的字串或字元。
使用此方法的正確語法如下:
String.Replace(" ", String.Empty);
這裡的 String.Empty
代表一個空的字串。該方法在字串中查詢空格,並將其替換為空的字串。
示例程式碼:
using System;
namespace Example {
class RemoveWhitespaces {
static void Main(string[] args) {
string String1 = "This is a String.";
String1 = String1.Replace(" ", String.Empty);
Console.WriteLine(String1);
}
}
}
輸出:
The old string is: This is a String.
The new string is: ThisisaString.
注意
此方法只能刪除單個空格字元
" "
,而不能刪除其他空白,例如製表符(\t
)或換行符(\n
)。C# 使用 Where
方法有效地刪除字串中的所有空格
方法 Where
是一個 LINQ
類方法。它用於執行各種有用的操作。我們在這裡使用它來刪除字串中的所有空格。
使用此方法刪除所有空格的正確語法如下:
String.Concat(OldString.Where(c => !Char.IsWhiteSpace(c)));
在這裡,我們使用 Where
在 IsWhiteSpace()
方法的幫助下找到字串的所有非空白。我們逐字元檢查了字串字元,然後用 String.Concat
方法來連線所有這些字元以再次形成字串。
示例程式碼:
using System;
using System.Linq;
namespace Example {
class RemoveAllWhitespaces {
static void Main(string[] args) {
string OldString = "This is a String.";
Console.WriteLine("The old string is: " + OldString);
string NewString = String.Concat(OldString.Where(c => !Char.IsWhiteSpace(c)));
Console.WriteLine("The new string is: " + NewString);
}
}
}
輸出:
The old string is: This is a String.
The new string is: ThisisaString.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe