How to Remove Item From List in C#
-
C# Program to Remove Item From
List
UsingRemove()
Method -
C# Program to Remove Item From
List
UsingRemoveAt()
Method -
C# Program to Remove Item From
List
UsingRemoveRange()
Method
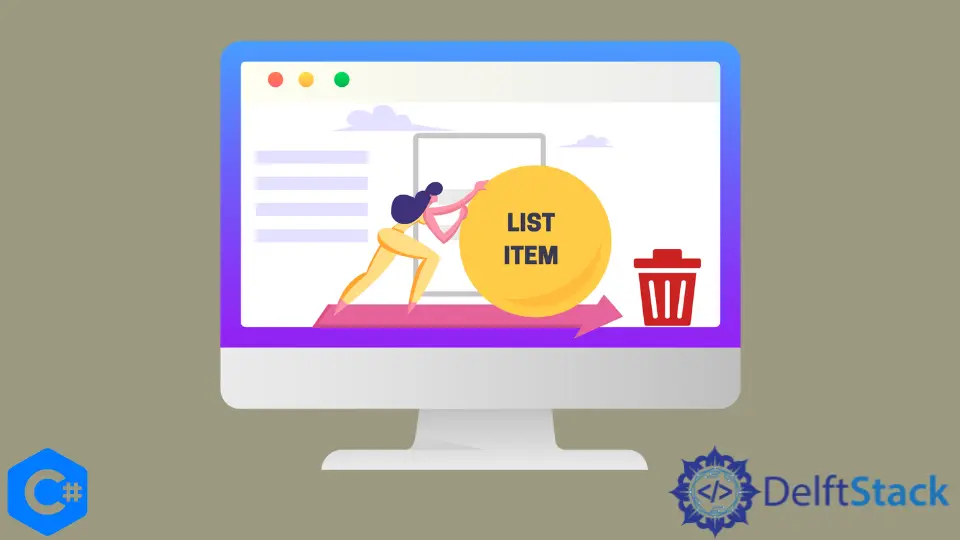
In C# we can perform several operations on the List
data structure. The items can be added, removed, replaced, etc. To remove an item from List
in C# we use Remove()
, RemoveAt()
and RemoveRange()
methods.
These methods remove the item from List
based on either its index or value. In the next few examples, you’ll learn how to implement these.
C# Program to Remove Item From List
Using Remove()
Method
This Remove()
method removes items based on its name on the List
. The correct syntax to use this method is as follows:
ListName.Remove("NameOfItemInList");
Example Codes:
using System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of Remove() method
Flowers.Remove("Lili");
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
Output:
List Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Hibiscus
Daisy
C# Program to Remove Item From List
Using RemoveAt()
Method
RemoveAt()
method removes the item from List
based on the index number of that item. We already know that indexes in C# start with 0. So, be careful while selecting the index number. The correct syntax to use this method is as follows:
ListName.RemoveAt(Index);
Example Codes:
using System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of RemoveAt() method
Flowers.RemoveAt(3);
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
Output:
List Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Lili
Daisy
C# Program to Remove Item From List
Using RemoveRange()
Method
In C#, we can also remove multiple items at the same time. For this purpose RemoveRange()
method is used. We pass the range of items to be removed as a parameter to the method. The correct syntax to use this method is as follows:
ListName.RemoveRange(int index, int count);
index
is the starting index of the elements to be removed, and count
is the number of elements to be removed.
Example Codes:
using System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of RemoveRange() method
Flowers.RemoveRange(3, 2);
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
Output:
List Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Lili