How to Get the First Object From List<Object> Using LINQ
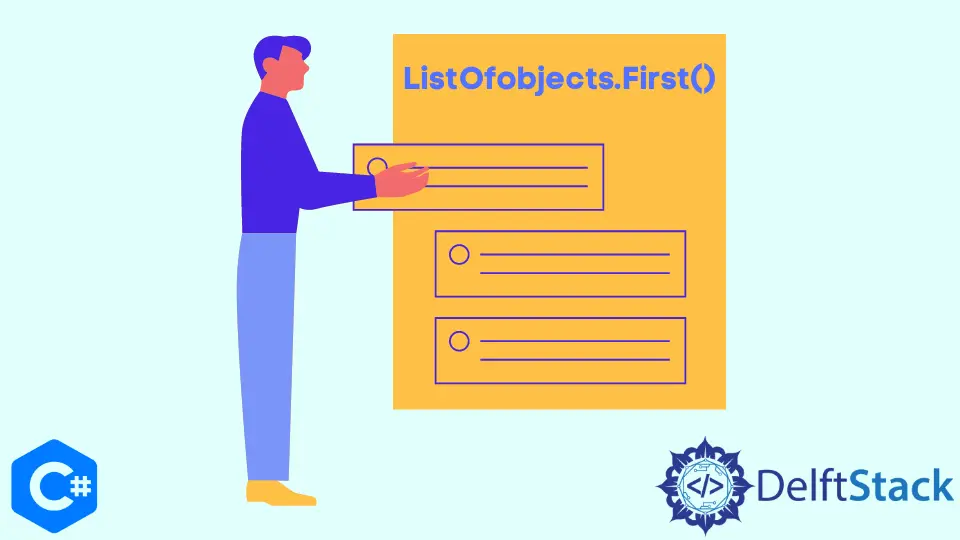
The topic we will cover in this tutorial is how to use LINQ in C# to get the first object from a list of objects. We’ll use the First()
method of LINQ to do this.
Use the First()
Method to Get the First Object From List<Object>
Using LINQ
The First()
function in LINQ returns the first element from the sequence of items in the list or collection or returns the first element in the line of items in the list, depending on the criteria supplied.
An error will be thrown by the LINQ First()
function if the list or collection does not contain any entries based on the provided constraints.
Let’s look at the First()
function implementation with the help of an example below.
-
Firstly, we have to import the following libraries.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
-
In the
Main()
, we’ll create a list of elements namedListofObjects
and populate it with random data.string[] ListOfobjects = { "Muhammad Zeeshan", "Nabeela G", "Bubby Shah", "Saada G" };
-
Now, we’ll create a string variable called
ResulttedValue
, and by applying theFirst()
method on the list, we’ll get the first element of the list.string ResulttedValue = ListOfobjects.First();
-
Lastly, we’ll print the resulting value on the console, as shown below.
Console.WriteLine("The First Object from the List of Objects is : {0}", ResulttedValue); Console.ReadLine();
Complete Source Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ExamplebyShani {
class Program {
static void Main(string[] args) {
string[] ListOfobjects = { "Muhammad Zeeshan", "Nabeela G", "Bubby Shah", "Saada G" };
string ResulttedValue = ListOfobjects.First();
Console.WriteLine("The First Object from the List of Objects is : {0}", ResulttedValue);
Console.ReadLine();
}
}
}
Output:
The First Object from the List of Objects is : Muhammad Zeeshan
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn