The AddRange Function for List in C#
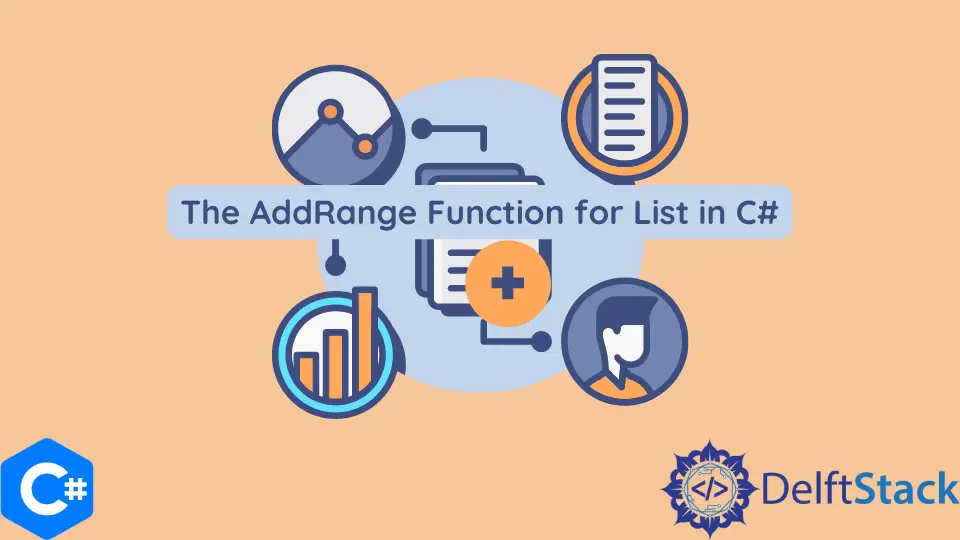
This article will provide a demonstration of the AddRange()
function and its operations that are done in the C# programming language.
Significance of the AddRange()
Function in C#
The AddRange
method in lists allows for adding a whole collection of components at once. When adding items from a particular collection to the end of a list, the AddRange
function of the C# programming language is used.
It can take the place of the tiresome foreach
loop, which continually uses the Add on List method. It is possible to send AddRange
not just an array or another List but also any other IEnumerable
collection.
InsertRange()
performs actions similar to those described above, but it also requires a starting point as an argument. First, we look at the AddRange()
instance method available in the List
base class library for the C# programming language.
InsertRange()
is the function that implements the AddRange()
function. AddRange()
, a wrapper method around InsertRange()
, has a destination position of 0 as its target location.
The AddRange
method differs from arrays in a few key respects. While lists may have their size dynamically increased, arrays cannot be expanded this way.
The System.Collections.Generic
namespace contains the AddRange(T)
function for the List
class. This function needs to receive the parameter collection
, which is considered a mandatory one.
System.Collections.Generic.IEnumerableT>
is the value type for the collection
argument. The collection whose items ought to be tacked on to the end of ListT>
is represented by IEnumerableT>
.
The order in which the collection components are stored is preserved by the ListT>
. The following description demonstrates the function’s prototype.
public void AddRange(System.Collections.Generic.IEnumerable<T> collection);
Implement the AddRange()
Function in C#
Now that we have everything out of the way let’s look at some instances to grasp them better. This is the most basic example possible to illustrate how the AddRange()
function accomplishes its goals.
Firstly, we create a list of integers and name their variable l
.
List<int> l = new List<int>();
After generating a list of integers, the next step is to add some items to the list we had just generated.
l.Add(1);
l.Add(2);
l.Add(3);
l.Add(4);
The next step is to build an array of the same type as the list, which is an array of the integer type with a size 4, and then to fill the array.
int[] arr = new int[4];
arr[0] = 5;
arr[1] = 6;
arr[2] = 7;
arr[3] = 8;
After that, we use the AddRange()
method on our list l
, and as an argument, we supply the arr
that we built earlier in the process.
l.AddRange(arr);
The list that we have will now be shown.
foreach (int val in l) {
Console.WriteLine(val);
}
We observed that each array member was also included in the list.
Source Code
using System;
using System.Collections.Generic;
public class AddRangeFunction {
public static void Main() {
List<int> l = new List<int>();
l.Add(1);
l.Add(2);
l.Add(3);
l.Add(4);
int[] arr = new int[4];
arr[0] = 5;
arr[1] = 6;
arr[2] = 7;
arr[3] = 8;
l.AddRange(arr);
foreach (int val in l) {
Console.WriteLine(val);
}
}
}
Output:
1
2
3
4
5
6
7
8
Here is another example.
using System;
using System.Collections.Generic;
public class AddRangeFunction {
public static void Main() {
List<int> l = new List<int>();
int[] arr = new int[4];
arr[0] = 5;
arr[1] = 6;
arr[2] = 7;
arr[3] = 8;
l.AddRange(arr);
foreach (int val in l) {
Console.WriteLine(val);
}
}
}
Output:
5
6
7
8
In this case, we followed the same procedure we did in the previous one. In this particular instance, though, there was nothing on our list.
The items on our list were not checked off. We have included the array in the list that was previously empty.
This example illustrates that adding items to an empty list is possible. Looking at the output, you will notice that the array’s values have been added to the list.
Suppose the new Count (the current Count plus the collection size) is more than the capacity. In that case, the capacity of ListT>
is increased by automatically reallocating the internal array to accommodate the additional items.
This occurs when the new Count is greater than the capacity. Before adding any new items, the previous elements are first copied over to the newly created array.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn