How to Convert an IEnumerable to a List in C#
-
Understanding
IEnumerable
andList
in C# -
Why Convert
IEnumerable
to aList
in C# -
Convert
IEnumerable
to aList
in C# Using theToList()
Method -
Convert
IEnumerable
to aList
in C# Using aList
Constructor -
Convert
IEnumerable
to aList
in C# Using the LINQ Query Syntax -
Convert
IEnumerable
to aList
in C# Using aforeach
Loop -
Convert
IEnumerable
to aList
in C# Using Extension Methods - Conclusion
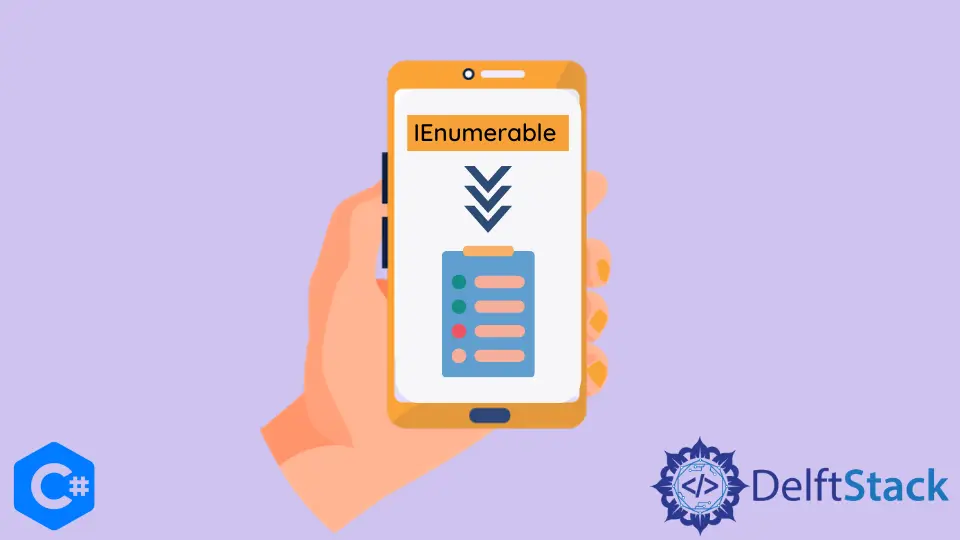
In C#, dealing with collections is a fundamental part of programming. One common task is converting an IEnumerable
to a List
.
Both of these data structures are prevalent in C# and have their unique characteristics and use cases. Understanding how to convert from one to the other is essential for efficient coding.
In this article, we will explore the various methods and scenarios for converting an IEnumerable
to a List
in C#.
Understanding IEnumerable
and List
in C#
Before we dive into conversion techniques, let’s briefly review what IEnumerable
and List
are.
IEnumerable
in C#
IEnumerable
is an interface in C# that represents a forward-only cursor of data. It’s the most basic type for working with collections and allows you to iterate over a collection’s elements.
IEnumerable
is suitable for lazy evaluation, which means that elements are retrieved one by one as you iterate over the collection, making it efficient for working with large datasets.
Here’s a simple example of an IEnumerable
:
IEnumerable<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
List
in C#
List
, on the other hand, is a generic collection type that implements the IEnumerable
interface. It’s part of the System.Collections.Generic
namespace and provides dynamic resizing, random access to elements, and various methods for manipulating the collection.
List
is great for scenarios where you need to perform frequent add, remove, and search operations on the data.
Here’s an example of a List
:
List<string> fruits = new List<string> { "Apple", "Banana", "Cherry" };
Why Convert IEnumerable
to a List
in C#
There are situations in which you might want to convert an IEnumerable
to a List
. Some of the common reasons include:
-
Eager Evaluation:
IEnumerable
uses lazy evaluation, which can be inefficient when you need to access elements multiple times or in random order. Converting to aList
allows for eager evaluation and faster access. -
Data Manipulation:
List
offers various methods for modifying, sorting, or filtering data. If you need to perform these operations, converting to aList
makes sense. -
API Compatibility: Some methods and libraries in C# expect a
List
as input. If you have anIEnumerable
and need to work with such APIs, conversion is necessary.
Now that we understand why we might want to convert an IEnumerable
to a List
, let’s explore the various methods to achieve this.
Convert IEnumerable
to a List
in C# Using the ToList()
Method
The most straightforward and widely used method for converting an IEnumerable
to a List
is by using the ToList()
method provided by the System.Linq
namespace. It’s available for any collection that implements the IEnumerable
interface, making it a versatile choice for converting various types of collections into a List
.
The ToList()
method is concise, readable, and efficient, making it the preferred option for most C# developers.
Here’s the basic syntax of using the ToList()
method:
IEnumerable<T> enumerable = // your IEnumerable<T> collection
List<T> list = enumerable.ToList();
In the above code, enumerable
represents your source IEnumerable
collection, and list
will hold the resulting List
after the conversion.
The ToList()
method works by iterating through the elements of the source IEnumerable
collection and creating a new List
containing the same elements. This operation doesn’t modify the original IEnumerable
but produces a separate List
that provides random access to its elements.
The resulting List
is essentially a copy of the source collection in a List
format, making it useful for scenarios where you need the benefits of random access and the versatility of a List
.
Let’s illustrate the usage of the ToList()
method with a practical example:
IEnumerable<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
List<int> numberList = numbers.ToList();
In this example, numbers
is an IEnumerable
containing integers, and numberList
is a List
that will store the converted elements. After applying the ToList()
method, numberList
will contain the same integers as the original numbers
collection.
List<int> numberList = new List<int> { 1, 2, 3, 4, 5 };
Now, you can efficiently access, manipulate, and perform various list operations on the numberList
, which was created from the original IEnumerable
.
Complete code:
using System;
using System.Collections.Generic;
using System.Linq;
namespace IEnumerableToListExample
{
class Program
{
static void Main(string[] args)
{
IEnumerable<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
List<int> numberList = numbers.ToList();
Console.WriteLine("Elements in the List:");
foreach (int num in numberList)
{
Console.WriteLine(num);
}
}
}
}
When you run this program, it will output the following:
Elements in the List:
1
2
3
4
5
This demonstrates how the ToList()
method efficiently converts an IEnumerable
collection to a List
in C#, allowing you to work with the converted list for various operations.
Convert IEnumerable
to a List
in C# Using a List
Constructor
There are multiple approaches to convert an IEnumerable
collection into a List
. The ToList()
method, as previously discussed, is one common method.
Another useful technique is using the List
constructor that accepts an IEnumerable
as an argument. This constructor is a straightforward way to create a new List
and populate it with elements from the source IEnumerable
.
This approach offers added flexibility and control during the conversion process, making it a valuable tool in certain scenarios.
Here’s how it’s done:
IEnumerable<T> enumerable = // your IEnumerable<T> collection
List<T> list = new List<T>(enumerable);
In this code snippet, enumerable
represents the source IEnumerable
collection, and list
is the List
that will hold the converted elements. This approach can be particularly helpful when you want to be explicit about the conversion process or when you need to perform additional setup or transformations on the elements before they are included in the List
.
Let’s illustrate the usage of the List
constructor with an example:
IEnumerable<string> names = new List<string> { "Alice", "Bob", "Charlie" };
List<string> nameList = new List<string>(names);
In this example, the names
collection is an IEnumerable
containing a list of names, and nameList
is a List
that will store the converted names. After using the List
constructor, nameList
will contain the same names as the original names
collection.
List<string> nameList = new List<string> { "Alice", "Bob", "Charlie" };
Complete code:
using System;
using System.Collections.Generic;
namespace IEnumerableToListConstructorExample
{
class Program
{
static void Main(string[] args)
{
IEnumerable<string> names = new List<string> { "Alice", "Bob", "Charlie" };
List<string> nameList = new List<string>(names);
Console.WriteLine("Elements in the List:");
foreach (string name in nameList)
{
Console.WriteLine(name);
}
}
}
}
When you run this program, it will output the following:
Elements in the List:
Alice
Bob
Charlie
The List
constructor provides a clean and straightforward way to convert an IEnumerable
into a List
without the need for explicit iteration or any additional code. This approach is particularly beneficial when you prefer a more direct and explicit conversion method, and it offers added control when custom logic or transformation is required during the conversion.
Convert IEnumerable
to a List
in C# Using the LINQ Query Syntax
When you need to convert an IEnumerable
into a List
while applying filtering, sorting, or other operations during the process, LINQ provides a flexible and expressive solution.
Using LINQ’s query syntax to achieve this conversion allows you to mold your data as it’s being transformed, giving you fine-grained control over the outcome.
To convert an IEnumerable
into a List
using LINQ query syntax, follow these steps:
IEnumerable<T> enumerable = // your IEnumerable<T> collection
List<T> list = (from item in enumerable select item).ToList();
In this code snippet, enumerable
represents your source IEnumerable
collection, and list
will contain the converted elements. The LINQ query iterates through the IEnumerable
, selecting each item, and eventually produces a new List
.
This method is particularly advantageous when you need to transform the data while performing the conversion.
Here’s an example that showcases the power of LINQ in sorting elements before converting an IEnumerable
to a List
:
IEnumerable<int> unsortedNumbers = new List<int> { 3, 1, 4, 2, 5 };
List<int> sortedNumberList = (from num in unsortedNumbers orderby num select num).ToList();
In this example, unsortedNumbers
is an IEnumerable
of integers in random order. The LINQ query, from num in unsortedNumbers orderby num
, sorts the numbers in ascending order.
After applying the ToList()
method, sortedNumberList
will contain the numbers in sorted order.
The resulting sortedNumberList
would look like this:
List<int> sortedNumberList = new List<int> { 1, 2, 3, 4, 5 };
Complete code:
using System;
using System.Collections.Generic;
using System.Linq;
namespace IEnumerableToListWithLinqExample
{
class Program
{
static void Main(string[] args)
{
IEnumerable<int> unsortedNumbers = new List<int> { 3, 1, 4, 2, 5 };
List<int> sortedNumberList = (from num in unsortedNumbers orderby num select num).ToList();
Console.WriteLine("Elements in the List:");
foreach (int num in sortedNumberList)
{
Console.WriteLine(num);
}
}
}
}
When you run this program, it will output the following:
Elements in the List:
1
2
3
4
5
This demonstrates how LINQ query syntax can be used not only for straightforward conversion but also for performing complex operations on the data during the conversion process.
Convert IEnumerable
to a List
in C# Using a foreach
Loop
When converting an IEnumerable
to a List
, a more hands-on approach involves using a foreach
loop to iterate through the elements of the IEnumerable
and add them one by one to a new List
. While this method is more manual and less concise compared to using built-in functions like ToList()
or LINQ, it offers complete control over the conversion process.
This level of control becomes advantageous when custom logic, filtering, or specific operations need to be applied during the conversion.
Here’s how you can employ a foreach
loop to convert an IEnumerable
to a List
:
IEnumerable<T> enumerable = // your IEnumerable<T> collection
List<T> list = new List<T>();
foreach (var item in enumerable)
{
list.Add(item);
}
In the above code snippet, a new List
is instantiated, and the elements from the IEnumerable
are individually added to the list
within the foreach
loop.
This method grants control over the process, enabling custom operations or filtering while elements are transferred from the IEnumerable
to the List
.
Let’s consider an example that illustrates how a foreach
loop can be utilized to filter elements during the conversion:
IEnumerable<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
List<int> evenNumberList = new List<int>();
foreach (var num in numbers)
{
if (num % 2 == 0)
{
evenNumberList.Add(num);
}
}
In this example, numbers
is an IEnumerable
of integers, and a new evenNumberList
is created to store only the even numbers.
During the iteration using the foreach
loop, each element is evaluated, and if it satisfies the condition (being an even number), it is added to the evenNumberList
.
The resulting evenNumberList
will contain only the even numbers from the original IEnumerable
.
Complete code:
using System;
using System.Collections.Generic;
namespace IEnumerableToListWithForeachExample
{
class Program
{
static void Main(string[] args)
{
IEnumerable<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
List<int> evenNumberList = new List<int>();
foreach (var num in numbers)
{
if (num % 2 == 0)
{
evenNumberList.Add(num);
}
}
Console.WriteLine("Even Numbers in the List:");
foreach (int num in evenNumberList)
{
Console.WriteLine(num);
}
}
}
}
When you run this program, it will output the following:
Even Numbers in the List:
2
4
This demonstrates how to use a foreach
loop to convert an IEnumerable
into a List
while allowing custom logic, filtering, or other specific operations during the conversion process.
Convert IEnumerable
to a List
in C# Using Extension Methods
In C#, extension methods are a powerful feature that allows you to add new functionality to existing types without modifying their source code. You can create an extension method for IEnumerable
to encapsulate the conversion process from IEnumerable
to List
.
This approach can improve code readability and reusability by abstracting the conversion logic into a well-defined and reusable method.
To create an extension method for IEnumerable
to convert it into a List
, you need to define a static class that contains the extension method. Here’s how it’s done:
public static class EnumerableExtensions
{
public static List<T> ToList<T>(this IEnumerable<T> enumerable)
{
return new List<T>(enumerable);
}
}
In this code, we’ve defined a static class named EnumerableExtensions
, which contains the extension method ToList
. This extension method takes an IEnumerable<T>
as its source and returns a List
containing the same elements.
The this
keyword in the method parameter indicates that it’s an extension method for IEnumerable<T>
. This allows you to call the method directly on any instance of IEnumerable
.
Once you’ve defined the extension method, you can use it throughout your codebase to convert IEnumerable
collections into List
instances with ease.
Here’s an example of how to use the ToList
extension method:
IEnumerable<string> colors = new List<string> { "Red", "Green", "Blue" };
List<string> colorList = colors.ToList();
In this example, the colors
collection is an IEnumerable
containing color names. By calling the ToList
extension method on the colors
collection, you can effortlessly convert it into a List<string>
.
This encapsulates the conversion logic and makes it more readable and reusable in your code.
Complete code:
using System;
using System.Collections.Generic;
public static class EnumerableExtensions
{
public static List<T> ToList<T>(this IEnumerable<T> enumerable)
{
return new List<T>(enumerable);
}
}
namespace IEnumerableToListWithExtensionMethodsExample
{
class Program
{
static void Main(string[] args)
{
IEnumerable<string> colors = new List<string> { "Red", "Green", "Blue" };
List<string> colorList = colors.ToList();
Console.WriteLine("Elements in the List:");
foreach (string color in colorList)
{
Console.WriteLine(color);
}
}
}
}
When you run this program, it will output the following:
Elements in the List:
Red
Green
Blue
This demonstrates how to use extension methods to encapsulate the conversion process from IEnumerable
to List
, improving code readability and reusability.
Conclusion
Converting an IEnumerable
to a List
is a common operation in C# programming. Each method for conversion has its advantages and is suited to different scenarios.
The choice of method depends on factors such as code readability, performance, and the need for additional data transformation.
In summary, the methods we discussed for converting an IEnumerable
to a List
in C# are:
- Using the
ToList()
method for a simple and efficient conversion. - Using a
List
constructor when you need additional setup or transformation. - Using LINQ query syntax for flexibility in data manipulation.
- Using a
foreach
loop for fine-grained control and custom logic. - Creating extension methods to encapsulate the conversion process.
Understanding when and how to use each of these methods will help you write efficient and maintainable code when working with collections in C#.