How to Convert IEnumerable to List in C#
-
Use
ToList()
to Convert Data FromIEnumerable
IntoList
in C# -
Use the
List
Constructor to ConvertIEnumerable
toList
in C# -
Use Manual Iteration to Convert
IEnumerable
toList
in C# -
Use
AsEnumerable()
to Convert Data FromList
IntoIEnumerable
in C# - Conclusion
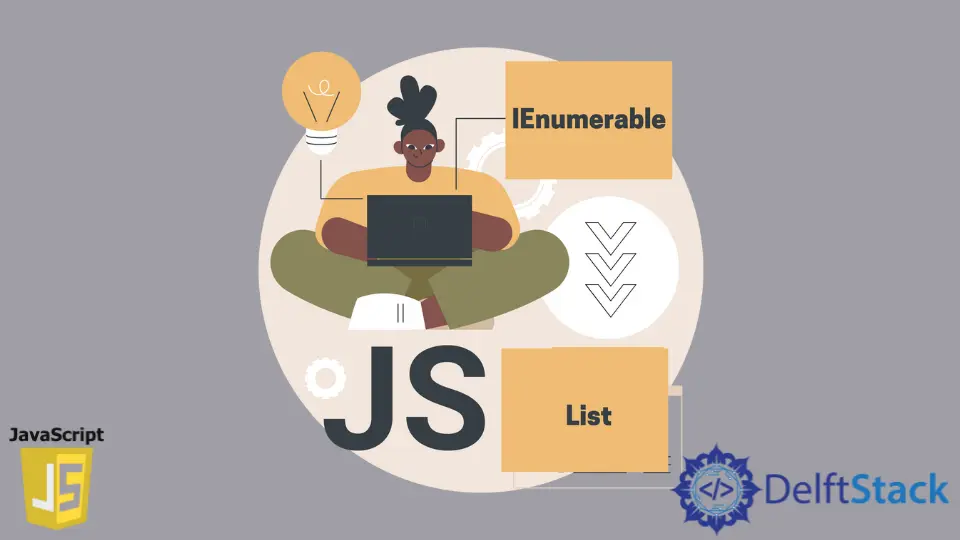
When working with collections in C#, it’s common to encounter scenarios where you need to convert an IEnumerable
into a List
. The IEnumerable
interface provides a generic way to iterate over a collection, but sometimes you need the additional features and methods provided by the List
class.
In this article, we will explore various methods to convert IEnumerable
to list in C#, each with its advantages and use cases.
Use ToList()
to Convert Data From IEnumerable
Into List
in C#
The most straightforward and concise method to convert an IEnumerable
to a List
is by using the ToList()
extension method. This method is available as part of the LINQ (Language Integrated Query) extension methods.
IEnumerable
is an interface contained in the System.Collections.Generic
namespace. Like every other interface, it exposes a method.
This case exposes the enumerator
method, which supports iteration or loop through generic and non-generic lists, including LINQ queries and Arrays. IEnumerable
contains only the GetEnumerator
method, which returns an IEnumerator
object.
public interface IEnumerable<out T> : System.Collections.IEnumerable
The values returned by the IEnumerable
interface are read-only. This implies that manipulation is limited to these data.
The ToList()
method in C# is an alternate method to manipulate these data. Elements in a list in C# can be added, removed, ordered, and re-arranged.
There are so many operations that can be performed on a list compared to the IEnumerable
values.
The C# List
class represents a collection of strongly typed objects that can be accessed by index. The ToList()
function or method is in the System.Linq
namespace (Language-Integrate Query).
The ToList
operator in LINQ takes the element from the given source and returns a new list. The input would be converted to a type list.
The ToList()
method returns a list of string instances. The ToList()
function can be invoked on an array reference or IEnumerable
values and vice versa.
List elements can be accessed or manipulated as follows:
// an array of 4 strings
string[] animals = { "Cow", "Camel", "Elephant" };
// creating a new instance of a list
List<string> animalsList = new List<string>();
// Use AddRange to add elements
animalsList.AddRange(animals);
// declaring a list and passing array elements into the list
List<string> animalsList = new List<string>(animals);
// Adding an element to the collection
animalsList.Add("Goat");
Console.WriteLine(animalsList[2]); // Output Elephant, Accessing elements of a list
// Collection of new animals
string[] newAnimals = { "Sheep", "Bull", "Camel" };
// Insert array at position 3
animalsList.InsertRange(3, newAnimals);
// delete 2 elements starting with the element at position 3
animalsList.RemoveRange(3, 2);
The namespace must be imported to use the ToList
function as follows:
using System.Collections.Generic;
using System.Linq;
Below is the syntax of the ToList()
method:
List<string> result = countries.ToList();
Below is an example code to convert IEnumerable
to a list in C#:
using System;
using System.Collections.Generic;
using System.Linq;
namespace TestDomain {
class Program {
public static void Main(String[] args) {
IEnumerable<int> testValues = from value in Enumerable.Range(1, 10) select value;
List<int> result = testValues.ToList();
foreach (int a in result) {
Console.WriteLine(a);
}
}
}
}
Output:
1
2
3
4
5
6
7
8
9
10
This code defines a program within the "TestDomain"
namespace. In the "Main"
method, it initializes an IEnumerable<int>
named testValues
using a LINQ query with Enumerable.Range(1, 10)
, which generates a sequence of integers from 1 to 10.
Subsequently, it converts this IEnumerable
to a List<int>
using the ToList()
method. Finally, a foreach
loop iterates through the elements in the resulting List<int>
named result
, and each element is printed to the console using Console.WriteLine
.
In this specific case, the output will display integers from 1 to 10, as generated by the LINQ query, showcasing the conversion from IEnumerable
to List
and subsequent iteration through the list.
Use the List
Constructor to Convert IEnumerable
to List
in C#
Another approach to convert from IEnumerable
to List
is by using the List
constructor that accepts an IEnumerable
as its parameter.
The List
class in C# provides a versatile constructor that allows you to initialize a new list with elements from an existing IEnumerable
collection. This constructor serves as a powerful tool for efficiently creating and populating a list in a single operation, minimizing the need for explicit iteration or additional LINQ methods.
Example Code:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
IEnumerable<int> enumerableData = GetSomeData();
// Convert IEnumerable to List using the List constructor
List<int> listData = new List<int>(enumerableData);
// Display the result
Console.WriteLine("Converted List:");
foreach (var item in listData) {
Console.WriteLine(item);
}
}
static IEnumerable<int> GetSomeData() {
// Some sample data
return new List<int> { 1, 2, 3, 4, 5 };
}
}
Output:
Converted List:
1
2
3
4
5
The List
constructor that accepts an IEnumerable
is a straightforward way to create a new List
instance and populate it with the elements from the provided IEnumerable
. This method is similar to using ToList()
and is suitable when you want a concise syntax without relying on LINQ.
Use Manual Iteration to Convert IEnumerable
to List
in C#
In some cases, you might need to perform a manual iteration over the IEnumerable
and add each element to the List
individually. This method provides more control over the conversion process.
Manual iteration involves explicit traversal of each element in the source IEnumerable
, adding them one by one to the destination List
. This method provides developers with fine-grained control over the conversion process and is particularly useful in scenarios where custom logic or additional checks are required during the transformation.
Example Code:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
IEnumerable<int> enumerableData = GetSomeData();
// Convert IEnumerable to List using manual iteration
List<int> listData = new List<int>();
foreach (var item in enumerableData) {
listData.Add(item);
}
// Display the result
Console.WriteLine("Converted List:");
foreach (var item in listData) {
Console.WriteLine(item);
}
}
static IEnumerable<int> GetSomeData() {
// Some sample data
return new List<int> { 1, 2, 3, 4, 5 };
}
}
Output:
Converted List:
1
2
3
4
5
This method involves manually iterating through each element of the IEnumerable
and adding it to the List
. While it provides more control, it may be less concise and efficient than using the built-in methods provided by LINQ.
Use AsEnumerable()
to Convert Data From List
Into IEnumerable
in C#
The AsEnumerable()
extension method is a powerful tool in the LINQ arsenal that facilitates the transition of a collection to a more abstract IEnumerable
. This method is particularly useful when you want to expose the functionality of LINQ operators without losing the original type information.
Below is an example code to convert IEnumerable
to a list and back to IEnumerable
.
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ListToArrayApp {
class Program {
static void Main(string[] args) {
List<int> list = new List<int>();
IEnumerable enumerable = Enumerable.Range(1, 5);
foreach (int item in enumerable) {
list.Add(item);
}
Console.WriteLine("Output as List");
foreach (var item in list) {
Console.WriteLine(item);
}
Console.WriteLine("Output as Enumerable");
// using the AsEnumerable to convert list back to Enumerable
IEnumerable resultAsEnumerable = list.AsEnumerable();
foreach (var item in resultAsEnumerable) {
Console.WriteLine(item);
}
}
}
}
Output:
Output as List
1
2
3
4
5
Output as Enumerable
1
2
3
4
5
This code within the "ListToArrayApp"
namespace demonstrates the conversion of data between a List<int>
and an IEnumerable
. Initially, a List<int>
named list
is created.
Using LINQ’s Enumerable.Range(1, 5)
, an IEnumerable
named enumerable
is generated, containing integers from 1 to 5. Through a foreach
loop, each element of the IEnumerable
is added to the List
.
The first output section displays the elements of the List
using a foreach
loop. Subsequently, the code uses the AsEnumerable()
method to convert the List
back into an IEnumerable
, creating a variable named resultAsEnumerable
.
The second output section then prints the elements of this resulting IEnumerable
. This illustrates the versatility of AsEnumerable()
in transitioning between different collection types, providing flexibility in the manipulation and utilization of data structures.
Conclusion
In this comprehensive guide, we explored various methods for converting IEnumerable
to List
in C#. The article covered four distinct approaches, each serving specific use cases:
ToList()
Method: A concise and efficient method using theToList()
extension method from LINQ. This approach provides simplicity and is suitable for most scenarios.List
Constructor: Utilizing theList
constructor that accepts anIEnumerable
as its parameter. This method is straightforward and useful when a concise syntax without LINQ is preferred.- Manual Iteration: A method involving explicit iteration through each element of
IEnumerable
and adding them one by one to a newList
. This approach offers fine-grained control for scenarios requiring custom logic during conversion. AsEnumerable()
Extension Method: Leveraging theAsEnumerable()
method to transition between different collection types, preserving deferred execution and retaining type information. This method is particularly valuable for seamless integration with LINQ.
The article concluded by emphasizing the importance of choosing the most suitable method based on factors such as readability, performance considerations, and the specific features required for the given scenario. Armed with a deep understanding of these conversion methods, C# developers can confidently navigate and optimize their collection manipulation tasks.
Related Article - Csharp List
- How to Convert an IEnumerable to a List in C#
- How to Remove Item From List in C#
- How to Join Two Lists Together in C#
- How to Get the First Object From List<Object> Using LINQ
- How to Find Duplicates in a List in C#
- The AddRange Function for List in C#