C#에서 IEnumerable을 목록으로 변환
-
ToList()
를 사용하여 IEnumerable에서C#
의 목록으로 데이터 변환 -
ToList()
를 사용하여C#
의 배열에서 목록으로 데이터 변환 -
C#
에서ToArray()
를 사용하여 목록에서 배열로 데이터 변환 -
AsEnumerable()
을 사용하여C#
의 목록에서 IEnumerable로 데이터 변환
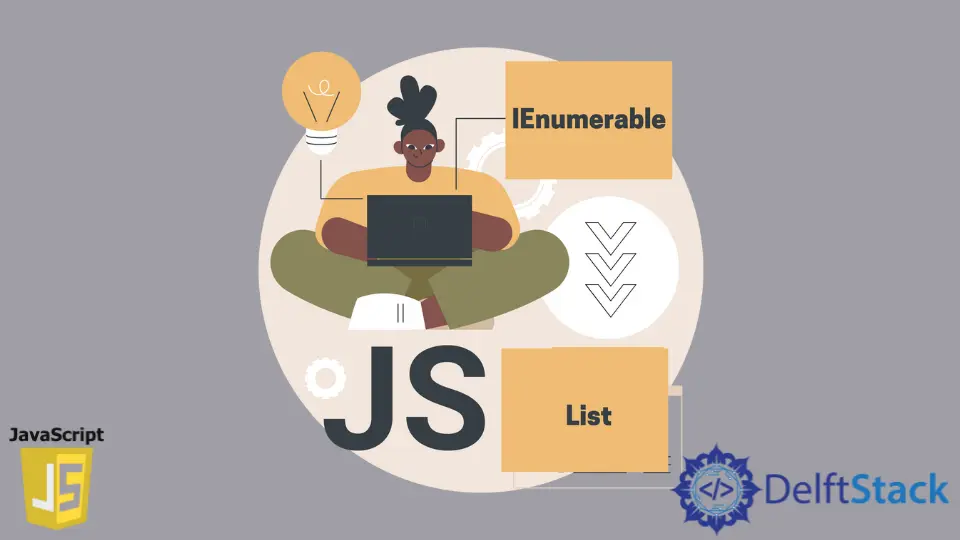
이 문서에서는 IEnumerable에서 C#의 목록으로 데이터를 변환하는 방법을 설명합니다.
ToList()
를 사용하여 IEnumerable에서 C#
의 목록으로 데이터 변환
IEnumerable은 System.Collections.Generic
네임스페이스에 포함된 인터페이스입니다. 다른 모든 인터페이스와 마찬가지로 메서드를 노출합니다.
이 경우는 LINQ 쿼리 및 배열을 포함하여 제네릭 및 비제네릭 목록을 통해 반복 또는 루프를 지원하는 enumerator
메서드를 노출합니다.
IEnumerable에는 IEnumerator 개체를 반환하는 GetEnumerator
메서드만 포함되어 있습니다.
public interface IEnumerable<out T> : System.Collections.IEnumerable
IEnumerable 인터페이스에서 반환된 값은 읽기 전용입니다. 이는 조작이 이러한 데이터로 제한됨을 의미합니다.
C#의 ToList()
메서드는 이러한 데이터를 조작하는 대체 메서드입니다. C#에서 목록의 요소를 추가, 제거, 정렬 및 재정렬할 수 있습니다.
IEnumerable 값에 비해 목록에서 수행할 수 있는 작업이 너무 많습니다.
C# List
클래스는 인덱스로 액세스할 수 있는 강력한 형식의 개체 컬렉션을 나타냅니다. ToList()
함수 또는 메서드는 System.Linq
네임스페이스(Language-Integrate Query)에 있습니다.
LINQ의 ToList
연산자는 주어진 소스에서 요소를 가져와서 새 목록을 반환합니다. 입력은 유형 목록으로 변환됩니다.
ToList()
메서드는 문자열 인스턴스 목록을 반환합니다. ToList()
함수는 배열 참조 또는 IEnumerable 값에서 호출할 수 있으며 그 반대의 경우도 마찬가지입니다.
이 참조를 통해 추가 논의가 가능합니다.
목록 요소는 다음과 같이 액세스하거나 조작할 수 있습니다.
// an array of 4 strings
string[] animals = { "Cow", "Camel", "Elephant" };
// creating a new instance of a list
List<string> animalsList = new List<string>();
// use AddRange to add elements
animalsList.AddRange(animals);
// declaring a list and passing array elements into the list
List<string> animalsList = new List<string>(animals);
// Adding an element to the collection
animalsList.Add("Goat");
Console.WriteLine(animalsList[2]); // Output Elephant, Accessing elements of a list
// Collection of new animals
string[] newAnimals = { "Sheep", "Bull", "Camel" };
// Insert array at position 3
animalsList.InsertRange(3, newAnimals);
// delete 2 elements at starting with element at position 3
animalsList.RemoveRange(3, 2);
다음과 같이 ToList
기능을 사용하려면 네임스페이스를 가져와야 합니다.
using System.Collections.Generic;
using System.Linq;
다음은 ToList()
메서드의 구문입니다.
List<string> result = countries.ToList();
다음은 C#에서 IEnumerable을 목록으로 변환하는 예제 코드입니다.
using System;
using System.Collections.Generic;
using System.Linq;
namespace TestDomain {
class Program {
public static void Main(String[] args) {
IEnumerable<int> testValues = from value in Enumerable.Range(1, 10) select value;
List<int> result = testValues.ToList();
foreach (int a in result) {
Console.WriteLine(a);
}
}
}
}
출력:
1
2
3
4
5
6
7
8
9
10
ToList()
를 사용하여 C#
의 배열에서 목록으로 데이터 변환
다음은 C#에서 배열을 목록으로 변환하는 예제 코드입니다.
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ArrayToListApp {
class Program {
static void Main(string[] args) {
// create an array of African countries of type string containing the collection of data
string[] countries = { "Nigeria", "Ghana", "Egypt", "Liberia",
"The Gambia", "Morocco", "Senegal" };
// countries.ToList() convert the data collection into the list.
List<string> result = countries.ToList();
// foreach loop is used to print the countries
foreach (string s in result) {
Console.WriteLine(s);
}
}
}
}
출력:
Nigeria
Ghana
Egypt
Liberia
The Gambia
Morocco
Senegal
C#
에서 ToArray()
를 사용하여 목록에서 배열로 데이터 변환
다음은 C#에서 목록에서 배열로 변환하는 예제 코드입니다.
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ListToArrayApp {
class Program {
static void Main(string[] args) {
// create an array of African countries of type string containing the collection of data
IEnumerable<int> testValues = from value in Enumerable.Range(1, 10) select value;
// countries.ToList() convert the data collection into the list.
List<int> result = testValues.ToList();
int[] array = result.ToArray(); // convert string to array.
// foreach loop is used to print the countries
foreach (int i in array) {
Console.WriteLine(i);
}
}
}
}
출력:
1
2
3
4
5
6
7
8
9
10
AsEnumerable()
을 사용하여 C#
의 목록에서 IEnumerable로 데이터 변환
다음은 IEnumerable을 목록으로 변환하고 다시 IEnumerable로 변환하는 예제 코드입니다.
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ListToArrayApp {
class Program {
static void Main(string[] args) {
List<int> list = new List<int>();
IEnumerable enumerable = Enumerable.Range(1, 5);
foreach (int item in enumerable) {
list.Add(item);
}
Console.WriteLine("Output as List");
foreach (var item in list) {
Console.WriteLine(item);
}
Console.WriteLine("Output as Enumerable");
// using the AsEnumerable to convert list back to Enumerable
IEnumerable resultAsEnumerable = list.AsEnumerable();
foreach (var item in resultAsEnumerable) {
Console.WriteLine(item);
}
}
}
}
출력:
Output as List
1
2
3
4
5
Output as Enumerable
1
2
3
4
5
관련 문장 - Csharp List
- IEnumerable을 C#의 목록으로 변환하는 방법
- C# 목록에서 항목 제거
- C# 두 목록을 함께 결합
- C#에 나열할 고유 항목
- C#에서 값을 사용하여 목록 초기화
- C#에서 내림차순으로 목록 정렬