C#에서 내림차순으로 목록 정렬
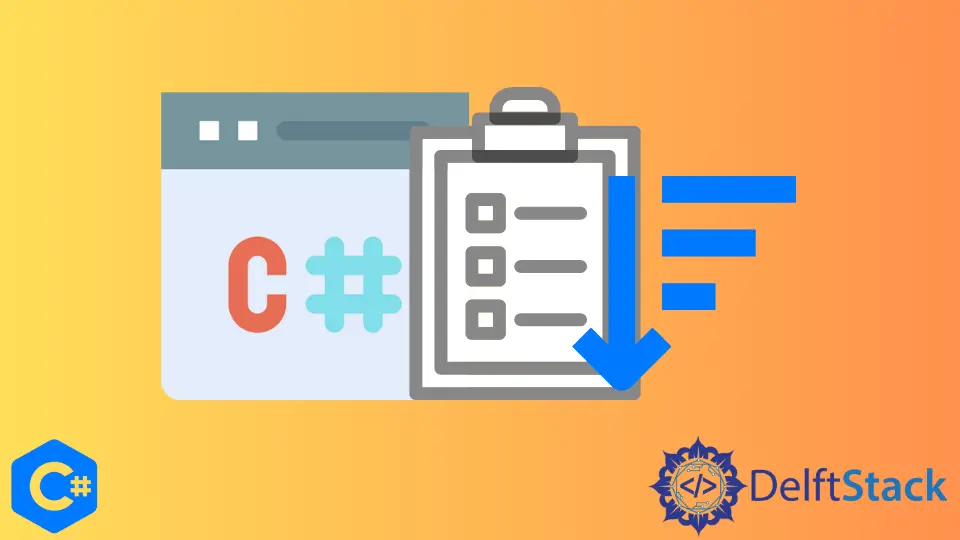
이 문서에서는 C#에서 정수 목록을 내림차순으로 정렬하는 방법을 소개합니다. 목록을 내림차순으로 정렬하는 방법에는 두 가지가 있습니다.
첫 번째 방법은 Reverse()
기능입니다. 목록의 모든 항목을 재정렬하는 데 사용됩니다.
두 번째 방법은 LINQ 라이브러리를 사용하는 것입니다. 이를 위해 orderby
키워드를 사용합니다.
Reverse()
함수를 사용하여 C#
에서 목록을 내림차순으로 정렬
이 시나리오에서는 먼저 Sort()
함수를 사용하여 목록을 오름차순으로 정렬합니다. 그런 다음 Reverse()
함수를 사용하여 목록을 내림차순으로 정렬합니다.
먼저 라이브러리를 가져옵니다.
using System;
using System.Collections.Generic;
main
메서드가 있는 DescSortList
라는 클래스를 만듭니다.
class DescSortList {
static void Main() {}
}
main
메서드 내에서 int 유형의 newList
라는 목록을 만듭니다.
List<int> newList = new List<int>();
이제 정렬해야 하는 목록에 임의의 숫자를 추가합니다.
newList.Add(8);
newList.Add(16);
newList.Add(14);
newList.Add(4);
newList.Add(26);
newList.Add(49);
newList.Add(2);
newList.Add(50);
목록에 추가된 숫자를 출력해 봅시다. 목록에서 반복하고 모든 반복에 대해 각 값을 출력하는 foreach
루프가 필요합니다.
System.Console.WriteLine("List Before Sorting");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
목록을 정렬하려면 newList
에서 Sort()
함수를 호출하면 목록이 오름차순으로 정렬되어 반환됩니다.
newList.Sort();
목록은 오름차순으로 정렬되었지만 내림차순으로 정렬해야 합니다.
따라서 목록에 있는 또 다른 함수인 Reverse()
함수를 호출해야 합니다. 목록을 내림차순으로 반환합니다.
newList.Reverse();
이제 목록이 내림차순으로 정렬되었습니다. foreach
루프를 다시 사용하여 목록을 출력합니다. 이것은 목록에서 반복되고 모든 인덱스에서 각 값을 출력합니다.
System.Console.WriteLine("\n" + "List in Descending Order");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
완전한 예제 코드
using System;
using System.Collections.Generic;
class DescSortList {
static void Main() {
List<int> newList = new List<int>();
newList.Add(8);
newList.Add(16);
newList.Add(14);
newList.Add(4);
newList.Add(26);
newList.Add(49);
newList.Add(2);
newList.Add(50);
System.Console.WriteLine("Given List");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
newList.Sort();
newList.Reverse();
System.Console.WriteLine("\n" + "Sorted List in Descending Order");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
}
}
출력:
Given List
8
16
14
4
26
49
2
50
Sorted List in Descending Order
50
49
26
16
14
8
4
2
LINQ 라이브러리를 사용하여 C#
에서 내림차순으로 목록 정렬
다음은 LINQ 라이브러리를 사용하여 목록을 정렬하는 다른 방법입니다. 이 방법에서는 목록을 만들고 임의의 정수를 저장합니다.
List<int> newList = new List<int>() { 5, 15, 3, 99, 11 };
이제 orderby
키워드를 사용하여 목록을 내림차순으로 정렬하고 List
유형 변수인 sortedList
에 모든 값을 저장합니다.
var sortedList = from y in newList orderby y descending select y;
목록을 내림차순으로 정렬했습니다.
각 목록 값을 출력해야 합니다. 따라서 foreach
루프를 사용합니다. 목록에서 반복하고 모든 반복에 대해 각 값을 출력합니다.
foreach (var y in sortedList) {
Console.WriteLine(y);
}
완전한 예제 코드
using System;
using System.Collections.Generic;
using System.Linq;
class DescSortList {
static void Main() {
List<int> newList = new List<int>() { 5, 15, 3, 99, 11 };
var sortedList = from y in newList orderby y descending select y;
Console.WriteLine("Sorted List\n");
foreach (var y in sortedList) {
Console.WriteLine(y);
}
}
}
출력:
Sorted List
99
15
11
5
3
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn