C# で降順でリストを並べ替える
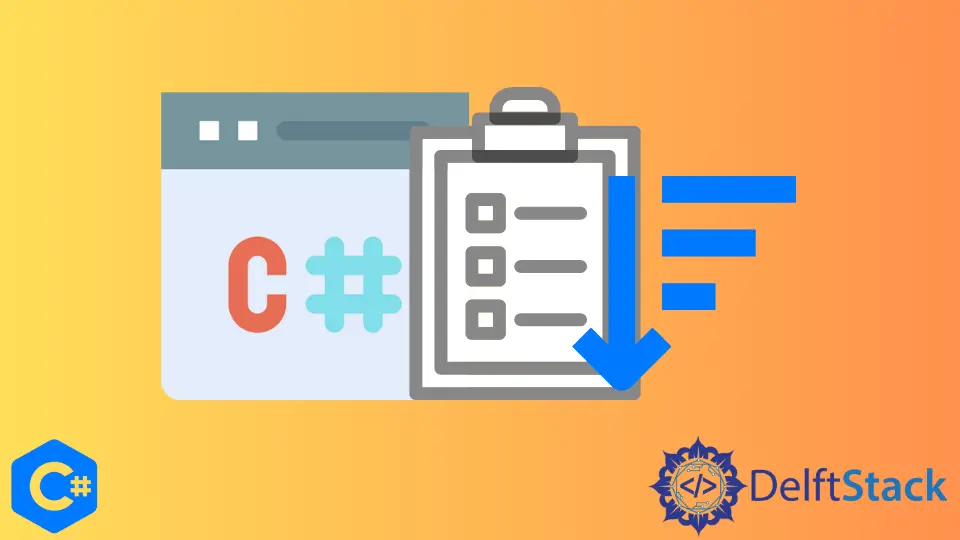
この記事では、C# で整数のリストを降順に並べ替える方法を紹介します。 リストを降順にソートするには、2つの方法があります。
最初のメソッドは Reverse()
関数です。 リスト内のすべてのエントリを並べ替えるために使用されます。
2つ目の方法は、LINQ ライブラリを使用することです。 これを行うには、orderby
キーワードを使用します。
C#
で Reverse()
関数を使用して降順でリストを並べ替える
このシナリオでは、最初に Sort()
関数を使用してリストを昇順に並べ替えます。 次に、Reverse()
関数を使用してリストを降順に並べ替えます。
まず、ライブラリをインポートします。
using System;
using System.Collections.Generic;
main
メソッドを内部に持つ DescSortList
という名前のクラスを作成します。
class DescSortList {
static void Main() {}
}
main
メソッド内で、int 型の newList
という名前のリストを作成します。
List<int> newList = new List<int>();
ここで、ソートする必要があるリストにいくつかの乱数を追加します。
newList.Add(8);
newList.Add(16);
newList.Add(14);
newList.Add(4);
newList.Add(26);
newList.Add(49);
newList.Add(2);
newList.Add(50);
リストに追加された数字を出力してみましょう。 リストを反復し、反復ごとに各値を出力する foreach
ループが必要です。
System.Console.WriteLine("List Before Sorting");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
リストをソートするには、newList
で Sort()
関数を呼び出すと、昇順でソートされたリストが返されます。
newList.Sort();
リストは昇順でソートされていますが、降順でソートする必要があります。
そのため、リストの別の関数 Reverse()
関数を呼び出す必要があります。 リストを降順で返します。
newList.Reverse();
これで、リストが降順にソートされました。 foreach
ループを再び使用して、リストを出力します。 これはリスト内で繰り返され、すべてのインデックスで各値を出力します。
System.Console.WriteLine("\n" + "List in Descending Order");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
完全なサンプルコード
using System;
using System.Collections.Generic;
class DescSortList {
static void Main() {
List<int> newList = new List<int>();
newList.Add(8);
newList.Add(16);
newList.Add(14);
newList.Add(4);
newList.Add(26);
newList.Add(49);
newList.Add(2);
newList.Add(50);
System.Console.WriteLine("Given List");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
newList.Sort();
newList.Reverse();
System.Console.WriteLine("\n" + "Sorted List in Descending Order");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
}
}
出力:
Given List
8
16
14
4
26
49
2
50
Sorted List in Descending Order
50
49
26
16
14
8
4
2
C#
で LINQ ライブラリを使用して降順でリストを並べ替える
LINQ ライブラリを使用してリストを並べ替える別の方法を次に示します。 このメソッドでは、リストを作成し、いくつかのランダムな整数を保存します。
List<int> newList = new List<int>() { 5, 15, 3, 99, 11 };
ここで、orderby
キーワードを使用してリストを降順に並べ替え、すべての値を List
型変数 sortedList
に保存します。
var sortedList = from y in newList orderby y descending select y;
降順でソートされたリストがあります。
各リスト値を出力する必要があります。 したがって、foreach
ループを使用します。 リストを反復し、反復ごとに各値を出力します。
foreach (var y in sortedList) {
Console.WriteLine(y);
}
完全なサンプルコード
using System;
using System.Collections.Generic;
using System.Linq;
class DescSortList {
static void Main() {
List<int> newList = new List<int>() { 5, 15, 3, 99, 11 };
var sortedList = from y in newList orderby y descending select y;
Console.WriteLine("Sorted List\n");
foreach (var y in sortedList) {
Console.WriteLine(y);
}
}
}
出力:
Sorted List
99
15
11
5
3
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn