How to Sort List in Descending Order in C#
-
Use the
Reverse()
Function to Sort List in Descending Order in C# - Use LINQ Query Syntax to Sort List in Descending Order in C#
-
Use the
OrderByDescending
Method to Sort List in Descending Order in C# -
Use the
List.Sort
Method to Sort List in Descending Order in C# - Implement Custom Sort Algorithm to Sort List in Descending Order in C#
- Conclusion
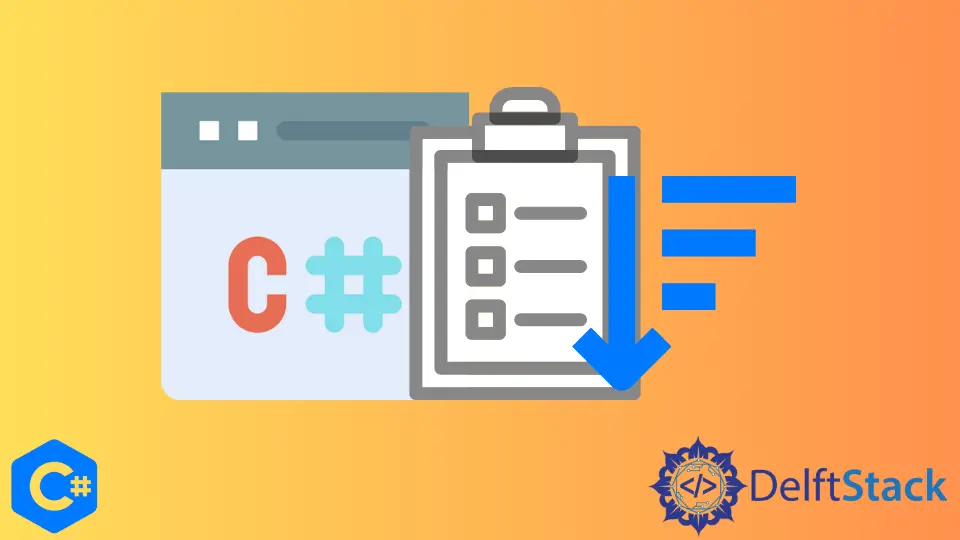
Sorting a list in descending order is a common operation in programming. In C#, there are multiple methods available to achieve this, leveraging different sorting algorithms or built-in functionalities.
This article introduces various methods on how to sort a list of integers in descending order in C#.
Use the Reverse()
Function to Sort List in Descending Order in C#
The Reverse()
function in C# is a method available for collections that reverses the order of elements within the collection. It operates directly on the original collection, altering the sequence of elements to appear in reverse.
Syntax:
List<T>.Reverse();
List<T>
: Represents a generic list collection in C#.T
: Represents the type of elements in the list.
Let’s consider a scenario where you have a list of integers that you want to sort in descending order using the Reverse()
function:
In this scenario, we first use the Sort()
function to arrange the list in ascending order. Then, we sort the list in descending order using the Reverse()
function.
Firstly, import the libraries:
using System;
using System.Collections.Generic;
Create a class named DescSortList
, inside which we’ll have our main
method.
class DescSortList {
static void Main() {}
}
Inside the main
method, create a list named newList
of type int
.
List<int> newList = new List<int>();
Now, add some random numbers to the list that must be sorted.
newList.Add(8);
newList.Add(16);
newList.Add(14);
newList.Add(4);
newList.Add(26);
newList.Add(49);
newList.Add(2);
newList.Add(50);
Let’s output the numbers added to the list. We need a foreach
loop that will iterate in the list and output each value against every iteration.
System.Console.WriteLine("List Before Sorting");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
To sort the list, call the Sort()
function on the newList
, and it will return the list sorted in ascending order.
newList.Sort();
The list has been sorted in ascending order, but we need it in descending order.
So, we need to call another function on the list, the Reverse()
function. It will return the list in descending order.
newList.Reverse();
Now, we have the list sorted in descending order. We’ll use the foreach
loop again to output the list; this will iterate in the list and output each value at every index.
System.Console.WriteLine("\n" + "List in Descending Order");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
Complete Example Code:
using System;
using System.Collections.Generic;
class DescSortList {
static void Main() {
List<int> newList = new List<int>();
newList.Add(8);
newList.Add(16);
newList.Add(14);
newList.Add(4);
newList.Add(26);
newList.Add(49);
newList.Add(2);
newList.Add(50);
System.Console.WriteLine("Given List");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
newList.Sort();
newList.Reverse();
System.Console.WriteLine("Sorted List in Descending Order");
foreach (int num in newList) {
System.Console.WriteLine(num);
}
}
}
This code initializes a list of integers, newList
, adds multiple integer values to it, and then performs sorting and reversing operations to arrange the list elements in descending order. Finally, it displays the original and sorted lists to demonstrate the sorting process.
Output:
Given List
8
16
14
4
26
49
2
50
Sorted List in Descending Order
50
49
26
16
14
8
4
2
Handling Custom Objects
The Reverse()
function is also applicable when dealing with custom objects:
using System;
using System.Collections.Generic;
class Person {
public string Name { get; set; }
public int Age { get; set; }
}
class Program {
static void Main() {
List<Person> people = new List<Person> { new Person { Name = "Alice", Age = 30 },
new Person { Name = "Bob", Age = 25 },
new Person { Name = "Charlie", Age = 40 } };
people.Sort((p1, p2) => p1.Age.CompareTo(p2.Age)); // Sort by Age in ascending order
people.Reverse(); // Reverse the sorted list to achieve descending order by Age
Console.WriteLine("People Sorted by Age in Descending Order:");
foreach (var person in people) {
Console.WriteLine($"Name: {person.Name}, Age: {person.Age}");
}
}
}
In this example, a Person
class with properties for Name
and Age
is defined. Inside the Main()
method, a list of Person
objects named people
is created and populated.
The list is first sorted based on the Age
property in ascending order using a lambda expression within the Sort()
method. Subsequently, the Reverse()
method is employed to reverse the sorted list, effectively arranging the people
list in descending order based on their ages.
Finally, it prints each person’s name and age from the sorted list, demonstrating the successful sorting in descending order by age.
Output:
People Sorted by Age in Descending Order:
Name: Charlie, Age: 40
Name: Alice, Age: 30
Name: Bob, Age: 25
Use LINQ Query Syntax to Sort List in Descending Order in C#
In C#, LINQ (Language-Integrated Query) offers a declarative syntax for querying data from various data sources. The LINQ query syntax resembles SQL syntax and allows developers to write queries against different data sources in a more readable and intuitive way.
It provides a concise way to manipulate data within collections, including filtering, sorting, and projecting data.
Basic Syntax
Here is the basic syntax structure of LINQ query syntax:
var query = from<range variable> in<data source><query body>
from
Clause: Specifies the range variable that represents each element in the data source.in
Keyword: Indicates the data source being queried.<query body>
: Contains query operations such as filtering, sorting, projecting, etc.
Query Operations
LINQ query syntax supports various operations:
where
Clause: Filters elements based on a specified condition.orderby
Clause: Sorts elements in ascending or descending order based on a specified key.select
Clause: Projects elements to a new form or extracts specific properties.group
Clause: Groups elements based on a specified key.join
Clause: Performs an inner or outer join between two data sources based on a common key.
Basic Example
Here’s an example demonstrating LINQ query syntax:
var query = from student in students
where student.Age> 18 orderby student.LastName ascending select new { student.FirstName, student.LastName };
students
: The data source (e.g., a list ofStudent
objects).student
: The range variable representing each element in thestudents
collection.where
: This filters students based on their age.orderby
: This sorts students by their last name in ascending order.select
: This projects a new anonymous type with specific properties (FirstName
andLastName
).
Additional Notes
- LINQ query syntax can be used with various data sources like collections (
List<T>
), arrays, databases, XML, etc. - It offers a more readable and natural way to express queries compared to method syntax.
- It’s versatile and allows developers to perform complex querying and manipulation operations on data sources.
Using LINQ query syntax enhances the readability and maintainability of code when performing data-related operations in C#.
Sorting a List in Descending Order with LINQ Query Syntax
Let’s explore how to sort a list of integers in descending order using LINQ query syntax. In this method, we create a list and store some random integers.
List<int> newList = new List<int>() { 5, 15, 3, 99, 11 };
Now, we use the orderby
keyword to sort a list in descending order, and we save all the values in the List
type variable sortedList
. We have the list sorted in descending order.
var sortedList = from y in newList orderby y descending select y;
We need to output each list value; hence, we use the foreach
loop. It will iterate in the list and output each value against every iteration.
foreach (var y in sortedList) {
Console.WriteLine(y);
}
Complete Example Code:
using System;
using System.Collections.Generic;
using System.Linq;
class DescSortList {
static void Main() {
List<int> newList = new List<int>() { 5, 15, 3, 99, 11 };
var sortedList = from y in newList orderby y descending select y;
Console.WriteLine("Sorted List");
foreach (var y in sortedList) {
Console.WriteLine(y);
}
}
}
This code snippet demonstrates sorting a list of integers in descending order using LINQ query syntax. It initializes a List<int>
named newList
containing integer values.
The code then uses LINQ query syntax to create a new sequence called sortedList
, sorting the elements of newList
in descending order. Finally, it iterates through sortedList
using a foreach
loop and displays each element on a new line, resulting in the sorted list being printed to the console.
Output:
Sorted List
99
15
11
5
3
Handling Custom Objects
LINQ query syntax is adaptable for sorting custom objects based on specific properties. This example demonstrates sorting people
objects by age in descending order using LINQ query syntax.
using System;
using System.Collections.Generic;
using System.Linq;
class Person {
public string Name { get; set; }
public int Age { get; set; }
}
class Program {
static void Main() {
List<Person> people = new List<Person> { new Person { Name = "Alice", Age = 30 },
new Person { Name = "Bob", Age = 25 },
new Person { Name = "Charlie", Age = 40 } };
var sortedPeople = from person in people orderby person.Age descending select person;
Console.WriteLine("People Sorted by Age in Descending Order:");
foreach (var person in sortedPeople) {
Console.WriteLine($"Name: {person.Name}, Age: {person.Age}");
}
}
}
This code defines a Person
class with properties for Name
and Age
. Inside the Main()
method, a list of Person
objects named people
is created and populated with individuals’ information.
The LINQ query syntax is used to sort the people
list based on the Age
property in descending order. The resulting sorted collection of Person
objects, sortedPeople
, is then iterated through, and their details—names and ages—are printed to the console, showcasing the list of people sorted by age in descending order.
Output:
People Sorted by Age in Descending Order:
Name: Charlie, Age: 40
Name: Alice, Age: 30
Name: Bob, Age: 25
Use the OrderByDescending
Method to Sort List in Descending Order in C#
Sorting a list in descending order using LINQ in C# involves the use of the OrderByDescending()
LINQ method. Here’s the syntax for accomplishing this:
var sortedList = collection.OrderByDescending(item => item.Property);
collection
: Represents the data source, such as a list (List<T>
), array, or any enumerable collection.OrderByDescending()
: This method is used to sort elements in descending order.item
: Represents each element in the collection during iteration.=>
: Lambda operator used to specify the sorting criteria.item.Property
: Refers to the property of each element by which the sorting should occur.
Consider a scenario where you have a list of integers and need to sort them in descending order using LINQ:
using System;
using System.Collections.Generic;
using System.Linq;
class Program {
static void Main() {
List<int> numbers = new List<int> { 5, 2, 9, 1, 7 };
var sortedList = numbers.OrderByDescending(x => x).ToList();
Console.WriteLine("Sorted List in Descending Order:");
foreach (var number in sortedList) {
Console.WriteLine(number);
}
}
}
In this code example, the OrderByDescending
method from LINQ sorts the elements in descending order based on the provided lambda expression (x => x
). This lambda expression defines the sorting criteria, indicating to sort the numbers in descending order based on their values.
After sorting, ToList()
converts the result back to a list, allowing easy iteration and access to the sorted elements. Finally, a simple iteration through the sortedList
displays the sorted numbers.
Output:
Sorted List in Descending Order:
9
7
5
2
1
Custom Sorting with LINQ
LINQ allows for custom sorting based on specific criteria beyond simple value sorting:
using System;
using System.Collections.Generic;
using System.Linq;
class Program {
static void Main() {
List<string> names = new List<string> { "Alice", "Bob", "Charlie", "David" };
var sortedNames = names.OrderByDescending(name => name.Length).ToList();
Console.WriteLine("Sorted Names by Length in Descending Order:");
foreach (var name in sortedNames) {
Console.WriteLine(name);
}
}
}
In this example, OrderByDescending
sorts the names based on their length in descending order, showcasing the flexibility of LINQ for customized sorting operations.
Output:
Sorted Names by Length in Descending Order:
Charlie
Alice
David
Bob
Use the List.Sort
Method to Sort List in Descending Order in C#
The List.Sort
method is a part of the List<T>
class in C#, specifically designed to sort the elements of a list. It can arrange elements based on custom comparison logic or by utilizing the default comparison for the data type.
The basic syntax of the List.Sort
method for sorting in descending order is:
list.Sort((x, y) => y.CompareTo(x));
list
: Represents the list of elements to be sorted.(x, y) => y.CompareTo(x)
: Defines a custom comparison delegate that specifies sorting in descending order.
Let’s explore an example demonstrating the usage of List.Sort
to arrange a list of integers in descending order:
using System;
using System.Collections.Generic;
class Program {
static void Main() {
List<int> numbers = new List<int> { 5, 2, 9, 1, 7 };
numbers.Sort((x, y) => y.CompareTo(x));
Console.WriteLine("Sorted List in Descending Order:");
foreach (var num in numbers) {
Console.WriteLine(num);
}
}
}
In the example code, the numbers.Sort((x, y) => y.CompareTo(x));
line uses the Sort
method on the numbers
list, providing a custom comparison delegate (x, y) => y.CompareTo(x)
to define descending order sorting based on integer values. The code then iterates through the sorted numbers
list and displays the elements in descending order.
Output:
Sorted List in Descending Order:
9
7
5
2
1
Implement Custom Sort Algorithm to Sort List in Descending Order in C#
Custom sort algorithms involve creating your logic to arrange elements within a collection based on specific criteria. Sorting algorithms typically focus on comparing elements and rearranging them to achieve an ordered sequence.
QuickSort is a divide-and-conquer algorithm that works by selecting a pivot
element from the array and partitioning the other elements into two sub-arrays according to whether they are less than or greater than the pivot. The sub-arrays are then recursively sorted. The process repeats until the entire array is sorted.
QuickSort Steps:
-
Choose a Pivot
Select an element from the array as the pivot.
-
Partitioning
Rearrange elements such that all elements less than the pivot are placed to the left, and elements greater than the pivot are on the right.
-
Recursion
Apply QuickSort recursively to the left and right sub-arrays.
Let’s implement the QuickSort algorithm to sort a list of integers in descending order. Below, the QuickSortDescending
function sorts the list numbers
in descending order using the QuickSort algorithm.
using System;
using System.Collections.Generic;
class Program {
static void Main() {
List<int> numbers = new List<int> { 5, 2, 9, 1, 7 };
QuickSortDescending(numbers, 0, numbers.Count - 1);
Console.WriteLine("Sorted List in Descending Order:");
foreach (var number in numbers) {
Console.WriteLine(number);
}
}
static void QuickSortDescending(List<int> arr, int low, int high) {
if (low < high) {
int pivot = Partition(arr, low, high);
QuickSortDescending(arr, low, pivot - 1);
QuickSortDescending(arr, pivot + 1, high);
}
}
static int Partition(List<int> arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] >= pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int swapTemp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = swapTemp;
return i + 1;
}
}
This code uses the QuickSort algorithm to sort a list of integers in descending order. The QuickSortDescending
method implements the QuickSort algorithm by recursively sorting sub-arrays based on a chosen pivot element.
The Partition
method determines the pivot, rearranges elements around it, and correctly positions elements greater than or equal to the pivot to the right. Finally, the sorted list in descending order is displayed using Console.WriteLine
.
Output:
Sorted List in Descending Order:
9
7
5
2
1
Conclusion
Sorting a list in descending order is a fundamental operation in programming, and C# offers multiple approaches to accomplish this task. This comprehensive guide explores various methods to sort a list of integers in descending order in C#.
- Using the
Reverse()
Function: This method reverses the elements of a list after sorting it in ascending order, effectively resulting in a descending order arrangement. - LINQ Query Syntax: Leveraging Language-Integrated Query (LINQ), developers can utilize a declarative SQL-like syntax to sort and manipulate data within collections, providing a clear and intuitive way to perform sorting operations.
- Implementing QuickSort Algorithm: QuickSort is a divide-and-conquer sorting algorithm that efficiently sorts lists in descending order. The article illustrates a custom implementation of QuickSort to achieve this.
- Using
OrderByDescending
Method: LINQ’sOrderByDescending
method facilitates sorting a collection in descending order by specifying a lambda expression that defines the sorting criteria. List.Sort
Method: C#’s built-inList<T>.Sort()
method allows sorting in descending order by providing a custom comparison delegate.
Each method is thoroughly explained, along with code examples demonstrating its application. Whether through leveraging built-in functionalities, utilizing LINQ’s query syntax, or implementing custom algorithms, C# provides a diverse set of tools for sorting lists in descending order, catering to different preferences and requirements in software development.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn