C# でリスト内の重複を見つける
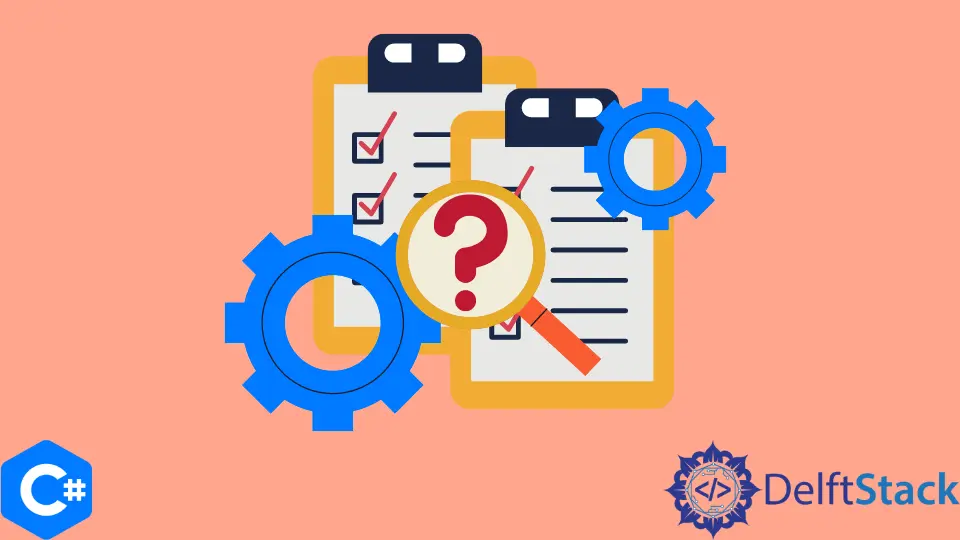
この記事を読むことで、C# プログラミング言語を使用してリスト内の重複するエントリを見つけるために実行する必要がある手順について理解を深めることができます。
このタスクを完了するために使用できる 2つの方法を次に示します。
Enumerable.GroupBy()
HashSet
Enumerable.GroupBy()
を使用して、C#
でリスト内の重複を検索する
Enumerable.GroupBy()
関数を使用して、各要素の値に従って要素をグループ化できます。 次に、フィルタによって一度しか存在しないグループが削除され、残りのグループには重複したキーが残されます。
まず、実装で使用する機能に不可欠なライブラリをインポートする必要があります。
using System;
using System.Collections.Generic;
using System.Linq;
文字列型の新しいリストを作成し、dataList.
という名前を付けます。 Main()
関数内で作成したばかりのリストにいくつかの項目を追加します。
List<string> dataList = new List<string>() { "Saad", "John", "Miller", "Saad", "Stacey" };
ここで、.GroupBy()
、.Where()
、および .Select()
関数を使用する IEnumerable<string>
型の変数 checkDuplicates
を初期化する必要があります。 このプロセスは、dataList
の重複をチェックします。
存在する場合は、checkDuplicates
変数に格納されます。
IEnumerable<string> checkDuplicates =
dataList.GroupBy(x => x).Where(g => g.Count() > 1).Select(x => x.Key);
この条件を使用して、重複が checkDuplicates
に保存されているかどうかを判断します。
この条件が満たされない場合、要素が書き出される代わりに、リストに重複する要素はありません
というメッセージがコンソールに表示されます。 一方、条件を満たしていれば、重複した項目が表示されます。
if (checkDuplicates.Count() > 0) {
Console.WriteLine("The duplicate elements in the list are: " +
String.Join(", ", checkDuplicates));
} else {
Console.WriteLine("No duplicate elements in the list");
}
完全なソース コード:
using System;
using System.Collections.Generic;
using System.Linq;
public class DuplicatesBySaad {
public static void Main() {
List<string> dataList = new List<string>() { "Saad", "John", "Miller", "Saad", "Stacey" };
IEnumerable<string> checkDuplicates =
dataList.GroupBy(x => x).Where(g => g.Count() > 1).Select(x => x.Key);
if (checkDuplicates.Count() > 0) {
Console.WriteLine("The duplicate elements in the list are: " +
String.Join(", ", checkDuplicates));
} else {
Console.WriteLine("No duplicate elements in the list");
}
}
}
出力:
The duplicate elements in the list are: Saad
HashSet を使用して C#
でリスト内の重複を見つける
ほとんどの場合、他のコレクションと重複する部分がコレクションに追加されるのを防ぎたい場合に使用します。 リストのパフォーマンスと比較すると、HashSet は全体的なパフォーマンスが大幅に優れています。
文字列データを格納する文字列型のリスト dataList
を作成します。
List<string> dataList = new List<string>() { "Saad", "John", "Miller", "Saad", "Stacey" };
その後、文字列型の hashSet
という名前の HashSet を作成し、リストからデータを保存する前に初期化する必要があります。
さらに、文字列型の IEnumerable
変数を作成し、duplicateElements
という名前を付ける必要があります。 この変数は dataList
をチェックし、重複が見つかった場合はそれらを追加して保存する必要があります。
最後に、重複した要素をコンソールに出力します。
HashSet<string> hashSet = new HashSet<string>();
IEnumerable<string> duplicateElements = dataList.Where(e => !hashset.Add(e));
Console.WriteLine("The duplicate elements in the list are: " +
String.Join(", ", duplicateElements));
完全なソース コード:
using System;
using System.Collections.Generic;
using System.Linq;
public class DuplicatesBySaad {
public static void Main() {
List<string> dataList = new List<string>() { "Saad", "John", "Miller", "Saad", "Stacey" };
HashSet<string> hashSet = new HashSet<string>();
IEnumerable<string> duplicateElements = dataList.Where(e => !hashSet.Add(e));
Console.WriteLine("The duplicate elements in the list are: " +
String.Join(", ", duplicateElements));
}
}
出力:
The duplicate elements in the list are: Saad
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn