C#의 목록에서 중복 찾기
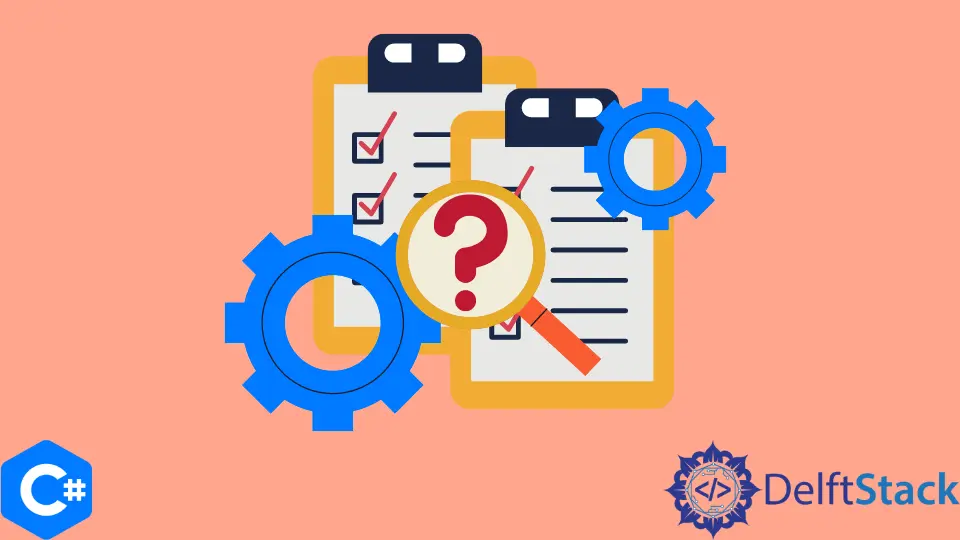
이 기사를 읽으면 C# 프로그래밍 언어를 사용하여 목록에서 중복 항목을 찾기 위해 수행해야 하는 단계를 더 잘 이해할 수 있습니다.
다음은 이 작업을 완료하는 데 사용할 수 있는 두 가지 접근 방식입니다.
Enumerable.GroupBy()
HashSet
Enumerable.GroupBy()
를 사용하여 C#
의 목록에서 중복 항목 찾기
Enumerable.GroupBy()
함수를 사용하여 각 요소의 값에 따라 요소를 그룹화할 수 있습니다. 그런 다음 필터는 한 번만 존재하는 그룹을 제거하고 나머지 그룹에는 중복 키를 남깁니다.
시작하려면 구현에 사용할 함수의 필수 라이브러리를 가져와야 합니다.
using System;
using System.Collections.Generic;
using System.Linq;
문자열 유형의 새 목록을 구성하고 dataList.
라는 이름을 지정합니다. Main()
함수 내에서 방금 생성한 목록에 일부 항목을 추가합니다.
List<string> dataList = new List<string>() { "Saad", "John", "Miller", "Saad", "Stacey" };
이제 .GroupBy()
, .Where()
및 .Select()
기능을 사용하는 IEnumerable<string>
유형의 checkDuplicates
변수를 초기화해야 합니다. 이 프로세스는 dataList
에서 중복을 확인합니다.
있는 경우 checkDuplicates
변수에 저장됩니다.
IEnumerable<string> checkDuplicates =
dataList.GroupBy(x => x).Where(g => g.Count() > 1).Select(x => x.Key);
조건을 사용하여 checkDuplicates
에 중복이 저장되었는지 여부를 결정합니다.
이 조건이 충족되지 않으면 “목록에 중복 요소 없음"이라는 메시지가 콘솔에 표시됩니다. 반면에 조건을 만족하면 중복된 항목이 표시됩니다.
if (checkDuplicates.Count() > 0) {
Console.WriteLine("The duplicate elements in the list are: " +
String.Join(", ", checkDuplicates));
} else {
Console.WriteLine("No duplicate elements in the list");
}
완전한 소스 코드:
using System;
using System.Collections.Generic;
using System.Linq;
public class DuplicatesBySaad {
public static void Main() {
List<string> dataList = new List<string>() { "Saad", "John", "Miller", "Saad", "Stacey" };
IEnumerable<string> checkDuplicates =
dataList.GroupBy(x => x).Where(g => g.Count() > 1).Select(x => x.Key);
if (checkDuplicates.Count() > 0) {
Console.WriteLine("The duplicate elements in the list are: " +
String.Join(", ", checkDuplicates));
} else {
Console.WriteLine("No duplicate elements in the list");
}
}
}
출력:
The duplicate elements in the list are: Saad
HashSet을 사용하여 C#
목록에서 중복 항목 찾기
대부분의 경우 컬렉션이 다른 것과 중복되는 조각으로 채워지는 것을 막고 싶을 때 사용합니다. 목록의 성능과 비교할 때 HashSet의 전반적인 성능이 상당히 우수합니다.
문자열 데이터를 저장할 문자열 유형의 목록 dataList
를 만듭니다.
List<string> dataList = new List<string>() { "Saad", "John", "Miller", "Saad", "Stacey" };
그런 다음 목록에서 데이터를 저장하기 전에 문자열 유형인 hashSet
이라는 이름으로 HashSet을 생성한 다음 초기화해야 합니다.
또한 문자열 유형의 IEnumerable
변수를 생성하고 duplicateElements
라는 이름을 지정해야 합니다. 이 변수는 dataList
를 확인하고 중복 항목이 있으면 추가하고 저장해야 합니다.
마지막으로 복제된 요소를 콘솔에 출력합니다.
HashSet<string> hashSet = new HashSet<string>();
IEnumerable<string> duplicateElements = dataList.Where(e => !hashset.Add(e));
Console.WriteLine("The duplicate elements in the list are: " +
String.Join(", ", duplicateElements));
완전한 소스 코드:
using System;
using System.Collections.Generic;
using System.Linq;
public class DuplicatesBySaad {
public static void Main() {
List<string> dataList = new List<string>() { "Saad", "John", "Miller", "Saad", "Stacey" };
HashSet<string> hashSet = new HashSet<string>();
IEnumerable<string> duplicateElements = dataList.Where(e => !hashSet.Add(e));
Console.WriteLine("The duplicate elements in the list are: " +
String.Join(", ", duplicateElements));
}
}
출력:
The duplicate elements in the list are: Saad
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn