C# 목록에서 항목 제거
Minahil Noor
2023년10월12일
Csharp
Csharp List
-
Remove()
메소드를 사용하여List
에서 항목을 제거하는 C# 프로그램 -
RemoveAt()
메소드를 사용하여List
에서 항목을 제거하는 C# 프로그램 -
RemoveRange()
메소드를 사용하여List
에서 항목을 제거하는 C# 프로그램
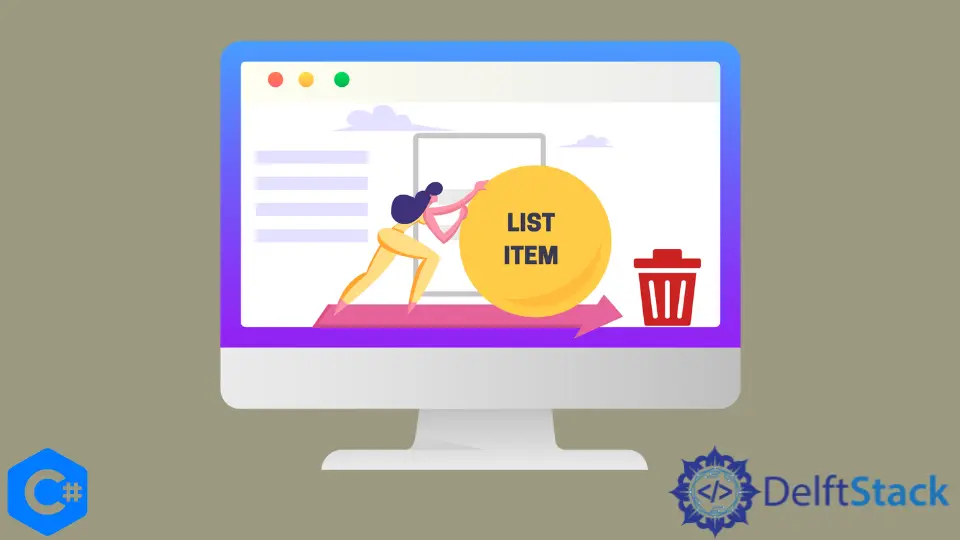
C#에서는List
데이터 구조에 대해 여러 가지 작업을 수행 할 수 있습니다. 항목은 추가, 제거, 교체 등이 가능합니다. C#에서List
에서 항목을 제거하려면Remove()
,RemoveAt()
및RemoveRange()
메소드를 사용합니다.
이 메소드들은 인덱스 나 값에 따라List
에서 아이템을 제거합니다. 다음 몇 가지 예에서는 이러한 구현 방법을 배웁니다.
Remove()
메소드를 사용하여List
에서 항목을 제거하는 C# 프로그램
이Remove()
메소드는List
의 이름을 기준으로 항목을 제거합니다. 이 방법을 사용하는 올바른 구문은 다음과 같습니다.
ListName.Remove("NameOfItemInList");
예제 코드:
using System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of Remove() method
Flowers.Remove("Lili");
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
출력:
List Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Hibiscus
Daisy
RemoveAt()
메소드를 사용하여List
에서 항목을 제거하는 C# 프로그램
RemoveAt()
메소드는 해당 항목의 색인 번호에 따라List
에서 항목을 제거합니다. 우리는 이미 C#의 인덱스가 0으로 시작한다는 것을 알고 있습니다. 따라서 인덱스 번호를 선택할 때주의하십시오. 이 방법을 사용하는 올바른 구문은 다음과 같습니다.
ListName.RemoveAt(Index);
예제 코드:
using System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of RemoveAt() method
Flowers.RemoveAt(3);
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
출력:
List Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Lili
Daisy
RemoveRange()
메소드를 사용하여List
에서 항목을 제거하는 C# 프로그램
C#에서는 여러 항목을 동시에 제거 할 수도 있습니다. 이를 위해RemoveRange()
메소드가 사용됩니다. 제거 할 항목 범위를 매개 변수로 메소드에 전달합니다. 이 방법을 사용하는 올바른 구문은 다음과 같습니다.
ListName.RemoveRange(int index, int count);
index
는 제거 할 요소의 시작 색인이고count
는 제거 할 요소의 수입니다.
예제 코드:
using System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of RemoveRange() method
Flowers.RemoveRange(3, 2);
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
출력:
List Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Lili
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다