C# 從列表中刪除元素
Minahil Noor
2023年10月12日
Csharp
Csharp List
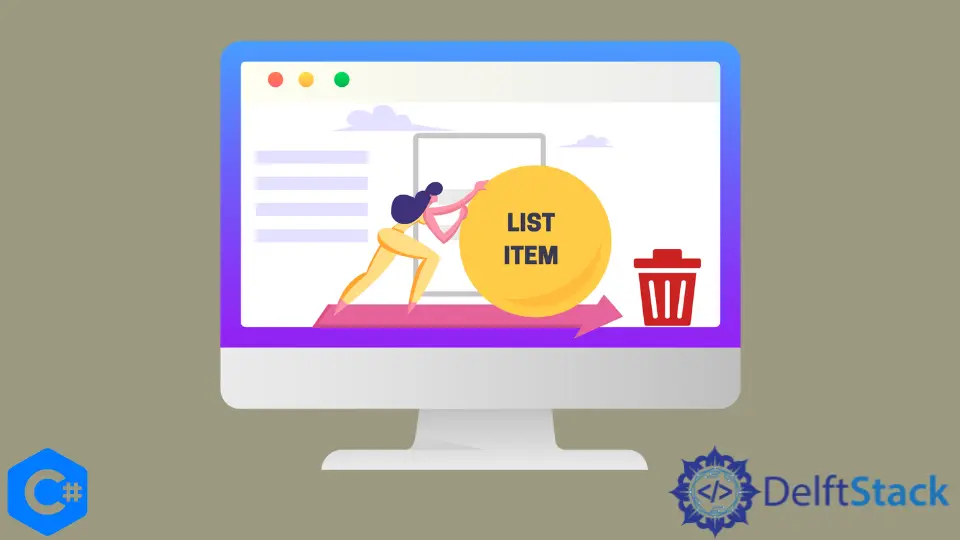
在 C# 中,我們可以對 List
資料結構執行幾種操作。可以新增、刪除和替換這些元素。要在 C# 中從列表中刪除一個元素,我們使用 Remove()
,RemoveAt()
和 RemoveRange()
方法。
這些方法根據索引或值將其從列表中刪除。在接下來的幾個示例中,你將學習如何實現這些。
C# 使用 Remove()
方法從 List
中刪除元素
這個 Remove()
方法根據列表上的名稱刪除元素。此方法的正確語法如下:
ListName.Remove("NameOfItemInList");
示例程式碼:
using System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of Remove() method
Flowers.Remove("Lili");
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
輸出:
List Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Hibiscus
Daisy
C# 使用 RemoveAt()
方法從 List
中刪除元素
RemoveAt()
方法根據該元素的索引號從 List 中刪除該元素。我們已經知道 C# 中的索引以 0 開頭。因此,選擇索引號時要小心。此方法的正確語法如下:
ListName.RemoveAt(Index);
示例程式碼:
using System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of RemoveAt() method
Flowers.RemoveAt(3);
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
輸出:
List Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Lili
Daisy
C# 使用 RemoveRange()
方法從 List
中刪除元素
在 C# 中,我們也可以同時刪除多個元素。為此,可以使用 RemoveRange()
方法。我們將要刪除的元素範圍作為引數傳遞給該方法。此方法的正確語法如下:
ListName.RemoveRange(int index, int count);
index
是要刪除的元素的起始索引,count
是要刪除的元素的數量。
示例程式碼:
using System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of RemoveRange() method
Flowers.RemoveRange(3, 2);
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
輸出:
List Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Lili
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe