C# 中的 HashSet 與列表
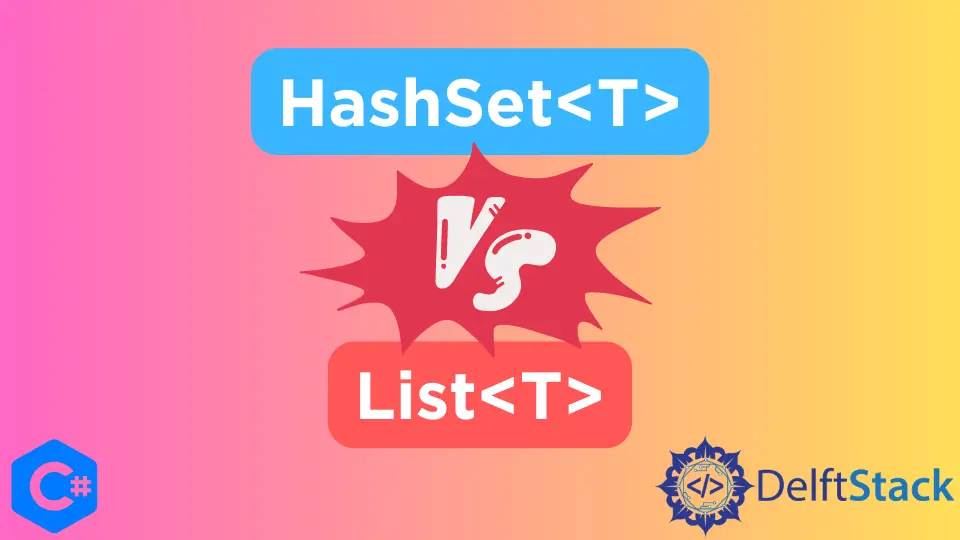
本教程將討論 C# 中的 HashSet<T>
和 List<T>
之間的區別和相似之處。
C# 中的 HashSet<T>
集合的數學定義是不同物件的無序集合。C# 中的 HashSet
資料結構也遵循相同的原則。
HashSet
是不遵循任何特定順序的非重複物件的集合。當我們不希望資料中有重複值時,通常使用 HashSet
。
它是包含在 System.Collection.Generic
名稱空間中的通用集合。
HashSet
的一個優點是我們可以應用所有集合操作,例如並集、交集和集差。使用 HashSet
的一個顯著缺點是我們無法對其進行排序,因為它不遵循任何特定的順序。
以下程式碼片段向我們展示瞭如何在 C# 中使用雜湊集。
HashSet<int> weirdNumbers = new HashSet<int>();
weirdNumbers.Add(10);
weirdNumbers.Add(13);
weirdNumbers.Add(17);
weirdNumbers.Add(78);
weirdNumbers.Add(13);
Console.WriteLine("HashSet before removal");
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
weirdNumbers.Remove(13);
Console.WriteLine("HashSet after removal");
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
輸出:
HashSet before removal
10
13
17
78
HashSet after removal
10
17
78
在上面的程式碼示例中,我們展示瞭如何建立一個通用雜湊集,將值插入其中,將它們從雜湊集中刪除,並在雜湊集中列印它們。
HashSet<T>
類中的 Add()
函式用於將單個值插入雜湊集中。除了 Add()
函式之外,HashSet<T>
類中還提供了許多其他有用的函式。
Remove(T val)
函式從我們的雜湊集中刪除 val
。它將值作為輸入引數並將其從我們的雜湊集中刪除。
C# 中的 List<T>
在 C# 中,list 是強型別物件的集合。可以通過索引訪問列表的元素。
列表物件包含許多用於排序、搜尋和修改列表的有用方法。它包含在 System.Collection.Generic
名稱空間中。
列表的另一個優點是它們提供編譯時型別檢查並且不執行裝箱/取消裝箱,因為它們是通用的。資料型別 T
的列表可以用 List<T>
初始化。
以下程式碼片段演示了我們如何在 C# 中使用列表。
List<int> weirdNumbers = new List<int>();
weirdNumbers.Add(10);
weirdNumbers.Add(17);
weirdNumbers.Add(78);
weirdNumbers.Add(13);
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
輸出:
10
17
78
13
在上面的程式碼中,我們在第一行初始化了整數列表 weirdNumbers
,使用 Add()
函式將值插入到列表中,並使用 foreach
迴圈列印列表中的所有值。
List<T>
類中的 Add()
函式用於將值插入到列表中。除了 Add()
函式之外,List<T>
類中還提供了許多其他有用的函式。
AddRange()
函式可以將另一個陣列或列表插入到現有列表中。它將列表或陣列作為輸入引數,並將列表或陣列的元素附加到主列表的末尾。
我們還可以使用 Insert()
函式在列表中的指定索引處插入單個值。Insert()
函式將整數索引和 T
型別值作為輸入引數。
我們已經討論了很多關於向列表中新增新元素的內容。讓我們討論如何從列表中刪除現有元素。
Remove(T val)
函式刪除列表中第一次出現的 val
。它將值作為輸入引數,並從索引 0 開始從列表中刪除該值的第一次出現。
RemoveAt(int index)
函式刪除列表中特定索引處的值。它將索引作為輸入引數並刪除放置的任何值。
下面的程式碼片段將我們討論的所有函式放在一個簡潔的小塊中。
List<int> weirdNumbers = new List<int>();
weirdNumbers.Add(10);
weirdNumbers.Add(17);
weirdNumbers.Add(78);
weirdNumbers.Add(13);
weirdNumbers.Insert(3, 31);
Console.WriteLine("List before removal");
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
weirdNumbers.Remove(31);
weirdNumbers.RemoveAt(1);
Console.WriteLine("List after removal");
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
輸出:
List before removal
10
17
78
31
13
List after removal
10
78
13
在上面的程式碼示例中,我們展示瞭如何建立一個通用列表、向其中插入值、從列表中刪除值以及列印列表中的值。
C# 中的 HashSet<T>
與 List<T>
列表和雜湊集都有各自的優點和缺點。雖然雜湊集比處理大量資料的列表快得多,但列表提供了索引功能。
雖然我們可以對列表進行排序,但雜湊集提供了執行集合操作的能力。
總之,列表或雜湊集,選擇完全取決於我們的問題。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn