HashSet vs List in C#
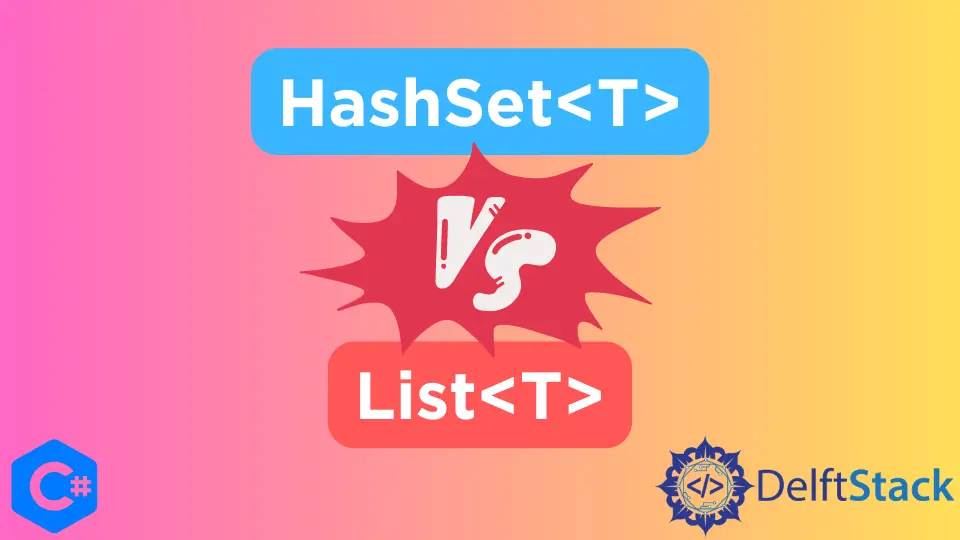
This tutorial will discuss the differences and similarities between a HashSet<T>
and a List<T>
in C#.
HashSet<T>
in C#
The mathematical definition of a set is an unordered collection of distinct objects. The HashSet
data structure in C# also follows the same principle.
A HashSet
is a collection of non-repeating objects that don’t follow any specific sequence. A HashSet
is generally used when we don’t want duplicate values in our data.
It is a generic collection included in the System.Collection.Generic
namespace.
An advantage of HashSet
is that we can apply all the set operations like union, intersection, and set difference. One notable disadvantage of using a HashSet
is that we can’t sort it because it doesn’t follow any specific sequence.
The following code snippet shows us how we can work with hash sets in C#.
HashSet<int> weirdNumbers = new HashSet<int>();
weirdNumbers.Add(10);
weirdNumbers.Add(13);
weirdNumbers.Add(17);
weirdNumbers.Add(78);
weirdNumbers.Add(13);
Console.WriteLine("HashSet before removal");
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
weirdNumbers.Remove(13);
Console.WriteLine("HashSet after removal");
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
Output:
HashSet before removal
10
13
17
78
HashSet after removal
10
17
78
We showed how we could create a generic hash set, insert values into it, remove them from the hash set, and print them inside the hash set in the above code example.
The Add()
function inside the HashSet<T>
class is used to insert a single value into the hash set. Along with the Add()
function, many other useful functions are provided within the HashSet<T>
class.
The Remove(T val)
function removes val
from our hash set. It takes the value as an input parameter and removes it from our hash set.
List<T>
in C#
In C#, a list is a collection of strongly typed objects. The elements of a list can be accessed via an index.
A list object contains many useful methods for sorting, searching, and modifying a list. It is included in the System.Collection.Generic
namespace.
Another advantage of lists is that they provide compile-time type checking and do not perform boxing/un-boxing because they are generic. A list of datatype T
can be initialized with List<T>
.
The following code snippets demonstrate how we can work with lists in C#.
List<int> weirdNumbers = new List<int>();
weirdNumbers.Add(10);
weirdNumbers.Add(17);
weirdNumbers.Add(78);
weirdNumbers.Add(13);
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
Output:
10
17
78
13
In the above code, we initialized the list of integers weirdNumbers
in the first line, inserted values into the list with the Add()
function, and used a foreach
loop to print all the values inside the list.
The Add()
function inside the List<T>
class is used to insert values into the list. Along with the Add()
function, many other useful functions are provided within the List<T>
class.
The AddRange()
function can insert another array or list into an existing list. It takes the list or array as input parameters and appends the elements of the list or array at the end of the primary list.
We can also use the Insert()
function to insert a single value at a specified index in the list. The Insert()
function takes the integer index and the T
type value as input parameters.
We’ve talked a lot about adding new elements to a list. Let’s discuss how we can remove existing elements from a list.
The Remove(T val)
function removes the first occurrence of the val
inside our list. It takes the value as an input parameter and removes the first occurrence of that value inside our list starting from index 0.
The RemoveAt(int index)
function removes the value at a specific index inside our list. It takes the index as an input parameter and removes whatever value is placed.
The following code snippet puts all the functions we discussed in a neat little block.
List<int> weirdNumbers = new List<int>();
weirdNumbers.Add(10);
weirdNumbers.Add(17);
weirdNumbers.Add(78);
weirdNumbers.Add(13);
weirdNumbers.Insert(3, 31);
Console.WriteLine("List before removal");
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
weirdNumbers.Remove(31);
weirdNumbers.RemoveAt(1);
Console.WriteLine("List after removal");
foreach (int val in weirdNumbers) {
Console.WriteLine(val);
}
Output:
List before removal
10
17
78
31
13
List after removal
10
78
13
We showed how we could create a generic list, insert values into it, remove values from the list, and print the values inside the list in the above code example.
HashSet<T>
vs. List<T>
in C#
Both lists and hash sets have their advantages and disadvantages over each other. While hash sets are much faster than lists for large amounts of data, lists provide indexing capabilities.
While we can sort lists, hash sets provide the ability to perform set operations.
In summary, lists or hash sets, the choice completely depends upon our problem.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn