C#에서 목록을 IEnumerable로 변환
-
C#에서
as
키워드를 사용하여 목록을 IEnumerable로 변환 - C#의 Typecasting 메서드를 사용하여 목록을 IEnumerable로 변환
- C#의 LINQ 메서드를 사용하여 목록을 IEnumerable로 변환
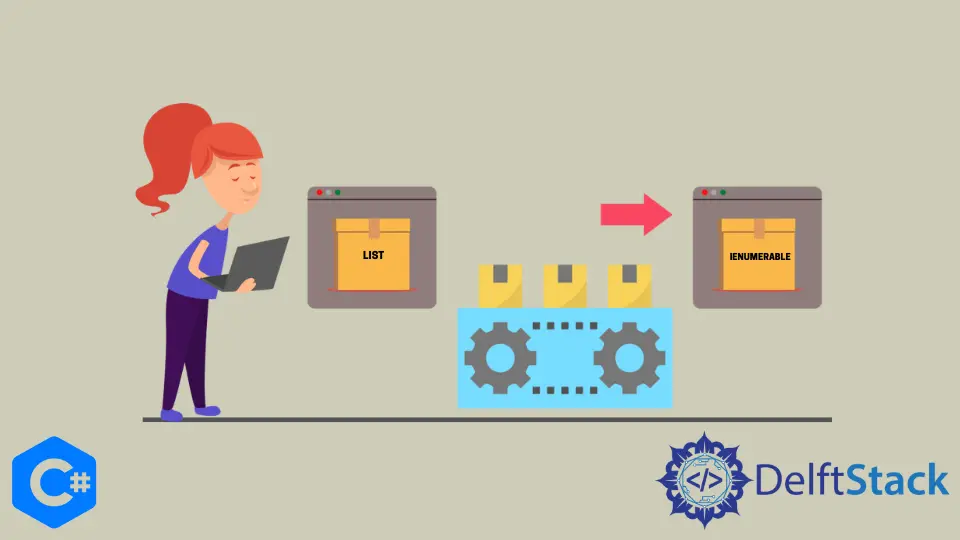
이 자습서에서는 C#에서 목록을IEnumerable
로 변환하는 방법에 대해 설명합니다.
C#에서as
키워드를 사용하여 목록을 IEnumerable로 변환
C#에서as
키워드를 사용하여List
데이터 구조를IEnumerable
데이터 구조로 변환 할 수 있습니다. 다음 예를 참조하십시오.
using System;
using System.Collections.Generic;
namespace list_to_ienumerable {
class Program {
static void Main(string[] args) {
List<int> ilist = new List<int> { 1, 2, 3, 4, 5 };
IEnumerable<int> enumerable = ilist as IEnumerable<int>;
foreach (var e in enumerable) {
Console.WriteLine(e);
}
}
}
}
출력:
1
2
3
4
5
위의 코드에서 우리는 C#에서as
키워드를 사용하여 정수ilist
의List
를 정수enumerable
의IEnumerable
로 변환했습니다.
C#의 Typecasting 메서드를 사용하여 목록을 IEnumerable로 변환
또한 typecasting 메소드를 사용하여List
데이터 유형의 객체를IEnumerable
데이터 유형의 객체에 저장할 수 있습니다 (다음 코드 예제 참조).
using System;
using System.Collections.Generic;
namespace list_to_ienumerable {
class Program {
static void Main(string[] args) {
List<int> ilist = new List<int> { 1, 2, 3, 4, 5 };
IEnumerable<int> enumerable = (IEnumerable<int>)ilist;
foreach (var e in enumerable) {
Console.WriteLine(e);
}
}
}
}
출력:
1
2
3
4
5
위의 코드에서 우리는 C#의 typecasting 메서드를 사용하여 정수ilist
의List
를 정수enumerable
의IEnumerable
로 변환했습니다.
C#의 LINQ 메서드를 사용하여 목록을 IEnumerable로 변환
LINQ는 SQL 쿼리 기능을 C#의 데이터 구조와 통합합니다. LINQ의AsEnumerable()
함수를 사용하여 목록을 C#의IEnumerable
로 변환 할 수 있습니다. 다음 코드 예제는 C#에서 LINQ의AsEnumerable()
함수를 사용하여List
데이터 구조를IEnumerable
데이터 구조로 변환하는 방법을 보여줍니다.
using System;
using System.Collections.Generic;
using System.Linq;
namespace list_to_ienumerable {
class Program {
static void Main(string[] args) {
List<int> ilist = new List<int> { 1, 2, 3, 4, 5 };
IEnumerable<int> enumerable = ilist.AsEnumerable();
foreach (var e in enumerable) {
Console.WriteLine(e);
}
}
}
}
출력:
1
2
3
4
5
위 코드에서 우리는 C#의ilist.AsEnumerable()
함수를 사용하여 정수ilist
의List
를 정수enumerable
의IEnumerable
으로 변환했습니다.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn관련 문장 - Csharp List
- IEnumerable을 C#의 목록으로 변환하는 방법
- C# 목록에서 항목 제거
- C# 두 목록을 함께 결합
- C#에 나열할 고유 항목
- C#에서 값을 사용하여 목록 초기화
- C#에서 내림차순으로 목록 정렬