How to Convert JSON Object to JavaScript Array
-
Convert JSON Object to an Array in JavaScript Using
for...in
Loop -
Convert JSON Object to an Array in JavaScript Using
Object.entries()
Loop
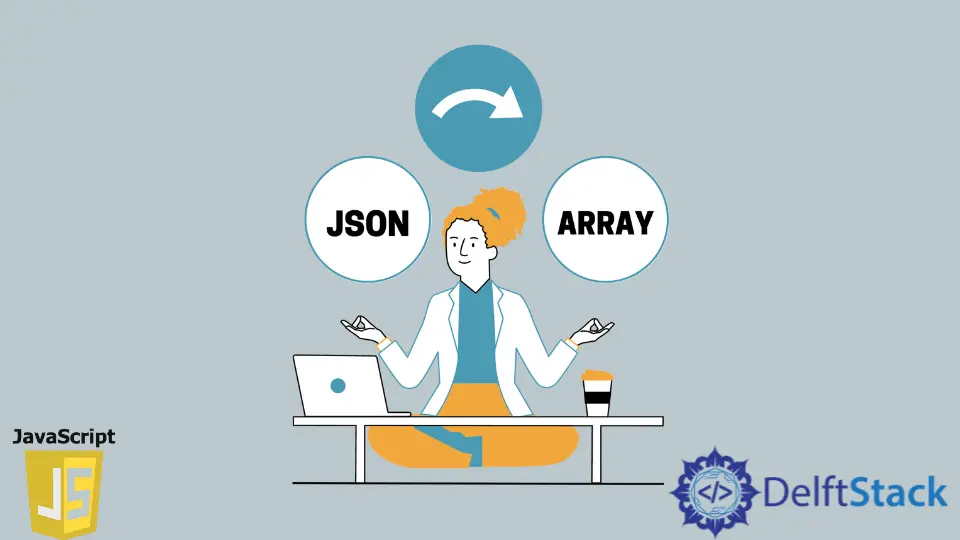
As in many other programming languages, objects in JavaScript can be compared to real-life objects. In JavaScript, an object is a stand-alone entity with properties and a type.
Compare it to a mug, for example. A car is an object with properties. A car has a color, design, model number, brand, etc. Likewise, JavaScript objects can have properties that define their characteristics.
Arrays are special types of objects whose keys are predefined in numeric form. Any Javascript object can be converted into an array. Arrays must use integers instead of strings as element indexes.
In this article, we will learn how to convert a JSON object to an array.
Convert JSON Object to an Array in JavaScript Using for...in
Loop
To convert an object into an array, you can use the for...in
loop in JavaScript. It iterates through all of an object’s enumerable properties that are string-encoded, including inherited enumerable properties. For example, let’s create an object with two properties and convert it to an array using the for...in
loop. See the code below.
Syntax:
for (variable in object) {
statement
}
This function iterates the object
. The variable
is assigned a different property name during each iteration. This method only extracts enumerable properties from an object
. For more information, read the documentation of for...in
method.
const object = {
1: 'Hello',
2: 'World'
};
const array = [];
for (var i in object) {
array.push([i, object[i]]);
}
console.log(array)
In the example above, we have defined two properties of an object 1 and 2. When you iterate the object, all properties in the object are checked, and the key, along with the value, is pushed to an array. When you run the above code in any browser, it will print something like this.
Output:
[["1", "Hello"], ["2", "World"]]
Convert JSON Object to an Array in JavaScript Using Object.entries()
Loop
The Object.entries()
method returns an array of string-key enumerable property pairs specific to a given object. It corresponds to iteration with a for...in
loop. The only difference is that a for...in
loop also lists the properties of the prototype string.
Object.entries()
return the array with the same order as that provided by a for...in
loop.
Syntax
Object.entries(obj);
The entries()
method takes an Object as a parameter whose own enumerable string-keyed property pairs are returned. It returns an array of the given object’s own enumerable string-keyed property pairs. Object.entries()
returns an array whose elements are pairs of enumerable string key properties in an object.
For more information, read the documentation of Object.entries()
method.
const object = {
1: 'Hello',
2: 'World'
};
const array = []
for (const [key, value] of Object.entries(object)) {
array.push([`${key}`, `${value}`]);
}
console.log(array)
In the above example, we are have defined the two properties of an array. When you iterate the object using entries, it will give you a key and property to be pushed to an array. When you run the above code in any browser, it will print something like this.
Output:
[["1", "Hello"], ["2", "World"]]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedInRelated Article - JavaScript JSON
- How to Format JSON in JavaScript
- How to Generate Formatted and Easy-To-Read JSON in JavaScript
- How to Convert XML to JSON in JavaScript
- How to Convert CSV to JSON in JavaScript
- How to POST a JSON Object Using Fetch API in JavaScript
- How to Convert JSON to XML in JavaScript