How to Search Objects From an Array in JavaScript
-
Search Objects in an Array Using
forEach()
in JavaScript -
Search Objects in an Array Using the
find()
Method in JavaScript
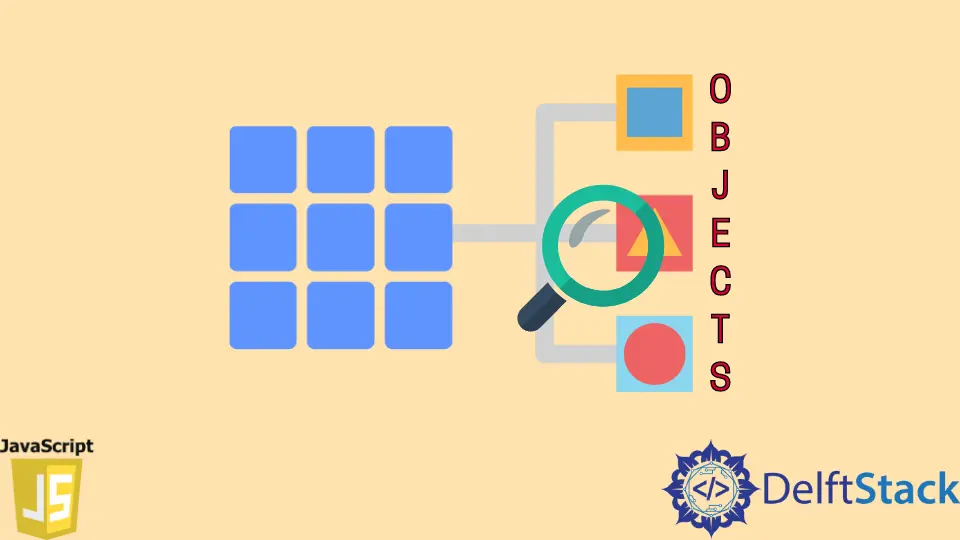
In JavaScript, working with and storing data inside an array data structure is quite common. An array data structure can take various ways such as numbers, strings, another array as an element, or objects.
In this article, let’s discuss how to search objects in an array and various values present inside every individual object.
Here, we have an array of objects with the name arrayofObjects
. Inside each object, there are various values represented by a key-value pair. There are 3 key-value pairs. The keys are name
, profession
and company
. Using these keys, we will be able to access the respective values of every individual object.
var arrayofObjects = [
{name: 'Adam', profession: 'Engineer', company: 'Google'},
{name: 'Sam', profession: 'Manager', company: 'Amazon'},
{name: 'Gerogia', profession: 'Designer', company: 'Netflix'},
{name: 'Kate', profession: 'Engineer', company: 'Microscoft'},
{name: 'James', profession: 'Sales', company: 'Amazon'},
];
An array is represented by square brackets []
whereas the objects are represented by curly braces {}
.
There are few ways by which you can find objects in an array. Some of the ways are shown below.
Search Objects in an Array Using forEach()
in JavaScript
The traditional way of finding objects in an array is by using a forEach()
loop. With this, we can loop through each element present inside the for a loop.
First, let’s access the entire objects using forEach()
from the array. Later we will see how to access individual values that are present inside the object. To print the entire object from the above array, you can use a forEach()
loop. This loop will provide us with two things: the element itself from the array and the element’s index. For that, we have to pass two parameters to forEach()
. In this case, we will only need the elements and not the index, so we will pass one parameter, object
. You can give any name to this parameter.
// print name of the people who work at amazon
arrayofObjects.forEach(object => {
console.log(object);
});
As the forEach()
loop will loop through every element, that element will be stored inside this object
variable. Now the object
variable will contain the entire object from the array. If you print this object
variable using the console, it will print all the objects present in the array.
Output:
Object { name: "Adam", profession: "Engineer", company: "Google" }
Object { name: "Sam", profession: "Manager", company: "Amazon" }
Object { name: "Gerogia", profession: "Designer", company: "Netflix" }
Object { name: "Kate", profession: "Engineer", company: "Microsoft" }
Object { name: "James", profession: "Sales", company: "Amazon" }
We have got every object from the array; let’s access inner values from these objects. Let’s now print the name of the employees who work at Amazon
company. To access any key from the object, we can use a dot after the object
variable and specify the key. For example, to access the company key, we will use object.company
.
// print name of the people who work at amazon
arrayofObjects.forEach(object => {
if (object.company === 'Amazon') {
console.log('Amazon Employee:', object.name);
}
});
Using an if
statement, we will compare the value of the key with the string Amazon
and if that matches, we will enter inside the if
statement. After that, we will print the name of the employees who work at amazon using object.name
as shown above. This will give us the below output.
Output:
Amazon Employee: Sam
Amazon Employee: James
Since only two people work at Amazon, we get Sam
and James
as an output.
Similarly, we can print all the values from all the objects using the name
, profession
, and company
keys below.
// print every details of a person
arrayofObjects.forEach(object => {
console.log(
object.name + ' is ' + object.profession + ' who works at ' +
object.company);
});
Output:
Adam is Engineer who works at Google
Sam is Manager who works at Amazon
Georgia is a Designer who works at Netflix
Kate is Engineer who works at Microsoft
James is Sales who works at Amazon
Search Objects in an Array Using the find()
Method in JavaScript
The find()
method is an alternate way of finding objects and their elements from an array in JavaScript. The find()
is an ES6 method. This method works similar to the forEach()
loop, and accessing the elements inside the object is similar to what we have seen before.
Replace forEach
with find
in your code, and you will be good to go. The below code will also print every object from the array.
arrayofObjects.find(object => {
console.log(object);
});
Output:
Object { name: "Adam", profession: "Engineer", company: "Google" }
Object { name: "Sam", profession: "Manager", company: "Amazon" }
Object { name: "Gerogia", profession: "Designer", company: "Netflix" }
Object { name: "Kate", profession: "Engineer", company: "Microsoft" }
Object { name: "James", profession: "Sales", company: "Amazon" }
Similarly, we can also access every value from the object from the array using their respective keys as follows.
arrayofObjects.find(object => {
console.log(
object.name + ' is ' + object.profession + ' who works at ' +
object.company);
});
Output:
Adam is Engineer who works at Google
Sam is Manager who works at Amazon
Georgia is Designer who works at Netflix
Kate is Engineer who works at Microsoft
James is Sales who works at Amazon
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedInRelated Article - JavaScript Object
- How to Get the Object's Value by a Reference to the Key
- How to Find Object in Array by Property Value in JavaScript
- How to Print Objects in JavaScript
- How to Destroy Object in JavaScript
- Nested Objects in JavaScriptn