How to Print Objects in JavaScript
-
Use the
console.log()
Method to Print Objects in JavaScript -
Use the
console.dir()
Method to Print Objects in JavaScript -
Difference Between
console.log()
andconsole.dir()
in JavaScript -
Use the
JSON.stringify()
Method to Print Objects in JavaScript - Conclusion
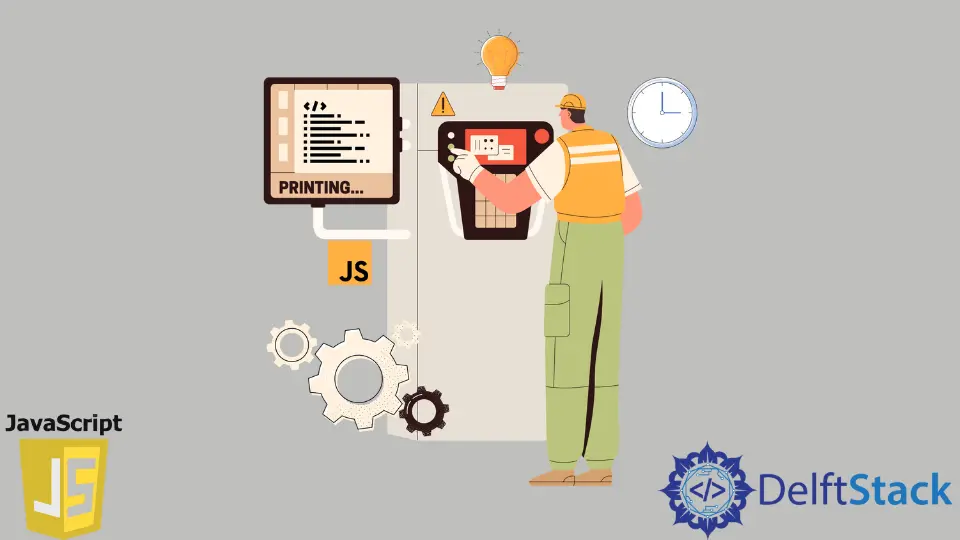
The print
function is one of the main functions of JavaScript.
In this article, you will learn common and easy techniques for printing objects in JavaScript.
The print
function in JavaScript displays or prints a JavaScript file’s content. It can be an object property or an array.
There are several ways to print objects in JavaScript.
Use the console.log()
Method to Print Objects in JavaScript
The console.log()
function is a common way to print an object in JavaScript. This function will display/print the argument on a web console then a string can obtain as a result.
Syntax:
console.log(object);
Let’s create an array called array
that contains values: Sam
and Roger
and an object called car
that contains object properties such as type
and model
. Then we can assign values to those properties as Ford
and Mustang
.
As shown below, we can print the array and object using the console.log()
function.
Example Code:
const array = ['Sam', 'Roger'];
const car = {
type: 'Ford',
model: 'Mustang'
};
console.log(array);
console.log(car);
After executing this code, the array and the object are displayed in string format.
Output:
For example, in the below code, the object car
has two object properties: type
and model
. The console.log()
function has been used to print those properties.
Example Code:
const car = {
type: 'Ford',
model: 'Mustang'
};
console.log(car.type);
console.log(car.model);
As a result, car type Ford
and model Mustang
were obtained.
Output:
Also, you can print the values in a single line using the console.log()
function.
Example Code:
const car = {
type: 'Ford',
model: 'Mustang'
};
console.log(car.type, ',', car.model);
The above code provides the result below.
Output:
Use the console.dir()
Method to Print Objects in JavaScript
The console.dir()
function is another way to print objects in JavaScript. With this, the user can print the properties of a specified object as a hierarchical listing, also known as an interactive listing.
You can see the object’s properties by clicking the disclosure triangle.
Syntax:
console.dir(object);
Let’s take the previous example. As shown below, you can use the console.dir()
function to print the object’s properties.
Here, the object car
does not need to be specified since it is the only object in the code.
Example Code:
const car = {
type: 'Ford',
model: 'Mustang'
};
console.dir(car);
As a result, we can acquire the object in the console with a disclosure triangle. After clicking it, we can view the child objects as follows.
Output:
Difference Between console.log()
and console.dir()
in JavaScript
The main difference between console.log()
and console.dir()
is that the console.log()
function gives us a toString
representation of the object and the console.dir()
function gives us an interactive list of the JavaScript object and its properties. Let’s take an example.
An array is declared with the values Ford
, Toyota
, and BMW
, and the object car
is declared with the properties type
and model
.
Both array and the object are displayed using the console.log()
function and console.dir()
function. As a result of the first two console.log()
functions, we can see their toString
formats.
Example Code:
const array = ['Ford', 'Toyota', 'BMW'];
const car = {
type: 'Ford',
model: 'Mustang'
};
console.log(array);
console.log(car);
console.dir(array);
console.dir(car);
Output:
Use the JSON.stringify()
Method to Print Objects in JavaScript
When considering web development, JSON is used to transform data from a web server and data to a web server. When transferring the data, it must reach the web server in string format; otherwise, errors will begin to occur.
Considering this, we also need to convert the objects in JavaScript into string format before sending them to the console. To achieve this, JavaScript provides the JSON.stringify()
function.
Through this function, objects will convert into string format and then be sent to the server successfully.
Syntax:
JSON.stringify(object);
Let’s add HP
as a new attribute to the previous object car
. HP refers to the horsepower of the car.
Also, HP
is numeric that has 460
as its value. The car
object can’t parse into the console without converting to a string because HP
is numeric.
So, let’s convert it into a string using the JSON.stringify()
function.
First, add a new property to the object as HP
and assign 460
as its value, and then use the JSON.stringify()
function to convert the car object into a string. Assign the JSON.stringify()
function to a new variable (carString
) and print the new variable using console.log()
function.
The code is as follows.
const car = {
type: 'Ford',
model: 'Mustang',
HP: 460
};
let carString = JSON.stringify(car);
console.log(carString);
Also, you can do this without declaring a new variable by making the JSON.stringify()
function the argument for the console.log()
function.
const car = {
type: 'Ford',
model: 'Mustang',
HP: 460
};
console.log(JSON.stringify(car));
The result in both ways is a string of the object but in JSON format.
Output:
Conclusion
Using the above methods, it is clear that we can print an object in JavaScript. There are a few more methods, such as console.table()
and Object.entries()
, where we can display objects in JavaScript, but the methods discussed here are the most commonly and frequently used.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.