How to Get the Object's Value by a Reference to the Key
- Access Property of an Object in JavaScript
- Get the Object’s Value by a Reference Variable Using the Square Bracket in JavaScript
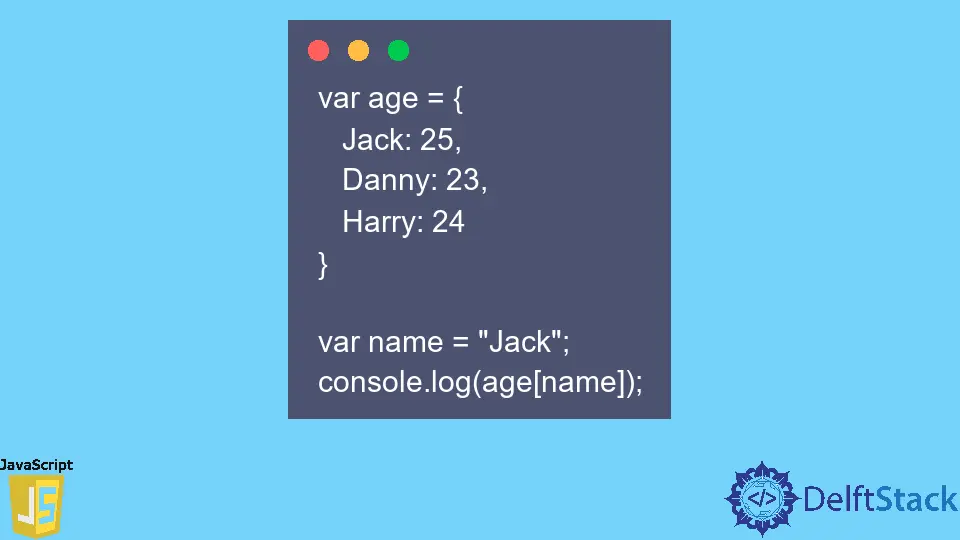
In JavaScript, the objects are written in key-value pairs. We can get the value of an object by the key.
But it is also possible to reference the key by a variable and use the variable to access the value in an object. This article will introduce the way to achieve it.
Access Property of an Object in JavaScript
JavaScript has various ways to access a property of an object. By property, it means the key of an object.
We can get a property’s value using square brackets []
, dot operator .
, and object destructuring. Let’s go through each of them.
We need to reference the property inside the square bracket to get the value, as shown in the example below.
Example Code:
var age = {Jack: 25, Danny: 23, Harry: 24}
console.log(age['Jack']);
Output:
25
In the example above, we have an object age
that contains properties in strings and values in numbers. Using the square bracket, we have accessed a value 25
by invoking the property Jack
.
Let’s try to do this with the dot operator.
console.log(age.Danny); // 23
We can access the value by invoking the property by the object using the dot operator. Notice the difference while using the square bracket notation.
We wrote the property within a quote. It is because the property is a string.
But we need not have to use the quote while using the dot operator even though the property is in string.
Now, let’s access the values by destructuring the object.
var {Harry} = age
console.log(Harry) // 24
Here, we placed the property Harry
inside the curly braces {}
. It works like a variable and holds the age
object as its value.
We can access the value of the property Harry
. This is called object destructuring.
Get the Object’s Value by a Reference Variable Using the Square Bracket in JavaScript
We can also reference the object’s property with a variable and use it to access the value from the object. It can be done using the square bracket notation []
.
For example, consider the age
object we created above. Next, create a variable name
and set it to Jack
.
Then, access the name
variable using the square bracket notation to get the object’s value, as shown below.
Example Code:
var age = {Jack: 25, Danny: 23, Harry: 24}
var name = 'Jack';
console.log(age[name]);
Output:
25
This is not possible while using a dot operator.
console.log(age.name); // undefined
In this way, we can use the square bracket to access the value of an object through the variable referenced by the property in JavaScript.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn