Nested Objects in JavaScriptn
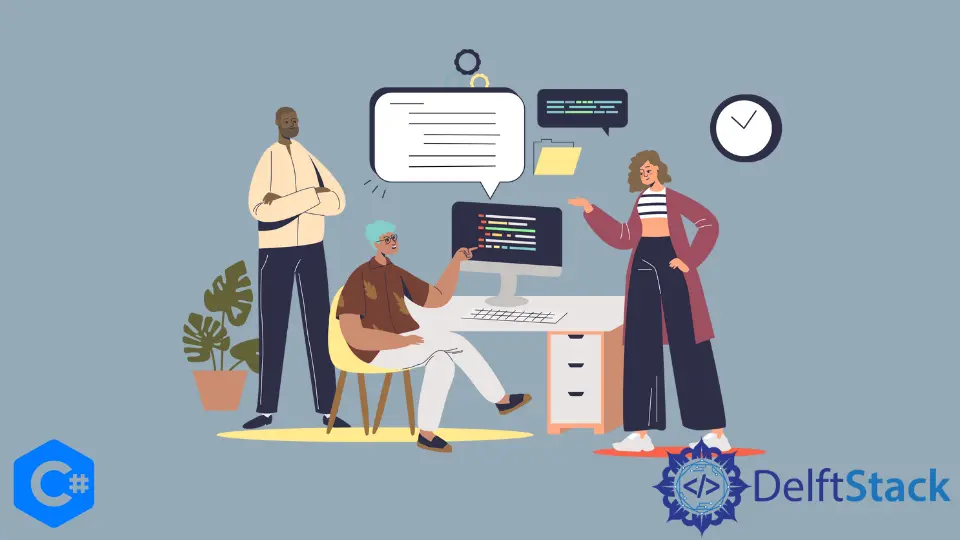
In this tutorial, we’ll learn what nested objects are and how to create them in JavaScript.
Create Nested Objects in JavaScript
In application code, objects are often nested. An object can have another object as a property, which could have a property, an array of even more objects.
Nested objects are objects that are inside another object. You can create nested objects within a nested object.
In the following example, Salary
is an object that resides inside the main object named Employee
. The dot notation can access the property of nested objects.
const employeeInfo = {
employeeName: 'John Doe',
employeeId: 27,
salary:
{2018 - 19: '400000INR', 2019 - 20: '500000INR', 2020 - 21: '650000INR'},
address: {
locality: {
address1: '1600 pebble road',
address2: 'Nearby XYZ Bank',
},
city: 'Mumbai',
state: 'Maharashtra',
country: 'India'
}
}
Access Nested Objects Properties by Destructuring the Object
The destructuring assignment syntax allows you to unpack array values or object properties into different variables.
Destructuring is a handy feature added to the ES6 version of JavaScript. It can quickly and conveniently extract properties or data from objects and arrays into separate variables.
Syntax:
({key1, key2, ...rest} = {key1: value1, key2: value2, key3: value3});
The rest
properties collect the remaining enumerable property keys the destructuring pattern has not yet selected.
You can find more information about Destructuring assignments in the documentation for Destructuring assignment
.
Let’s first understand how to destructure objects in JavaScript.
const {salary} = employeeInfo;
console.log(salary);
const {address: {locality} = {}} = employeeInfo;
console.log(locality);
In the above example, we extract the salary
object from the employee
object and the location
object from the address
object, nested under the employee object.
If the address object is not found, it will throw an error. To avoid this error, we can initialize empty objects while extracting the address object.
Output:
{
2018-19: "400000INR",
2019-20: "500000INR",
2020-21: "650000INR"
}
{
address1: "1600 pebble road",
address2: "Nearby XYZ Bank"
}
Access Nested Objects Properties Using the .(dot)
Notation
Property access methods provide access to properties of an object using either the dot notation or bracket notation. The dot notation is used to extract object properties step by step.
First, let’s look at how to access nested properties of objects in JavaScript.
Syntax:
object.property
object['property']
When discussing the properties of an object, it’s common to distinguish between properties and methods.
A method is a property that can be invoked (for example, if it has a reference to a Function instance as its value).
You can find more information about Property accessors in the documentation for Property accessors
.
console.log(employeeInfo.salary);
console.log(employeeInfo.address.locality);
In the example, we assumed that the address object would be present in the employee object. If the address object is not present, it will throw an error: can not read the property of undefined
.
Output:
{
2018-19: "400000INR",
2019-20: "500000INR",
2020-21: "650000INR"
}
{
address1: "1600 pebble road",
address2: "Nearby XYZ Bank"
}
You can run the code discussed in this tutorial here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn