How to Find Object in Array by Property Value in JavaScript
-
Find Object in Array by Property Value Using the
find()
Method -
Find Object in Array by Property Value Using the
filter()
Method -
Find Object in Array by Property Value Using JavaScript
for
Loop -
Find Object in Array by Property Value Using JavaScript
for...in
Loop
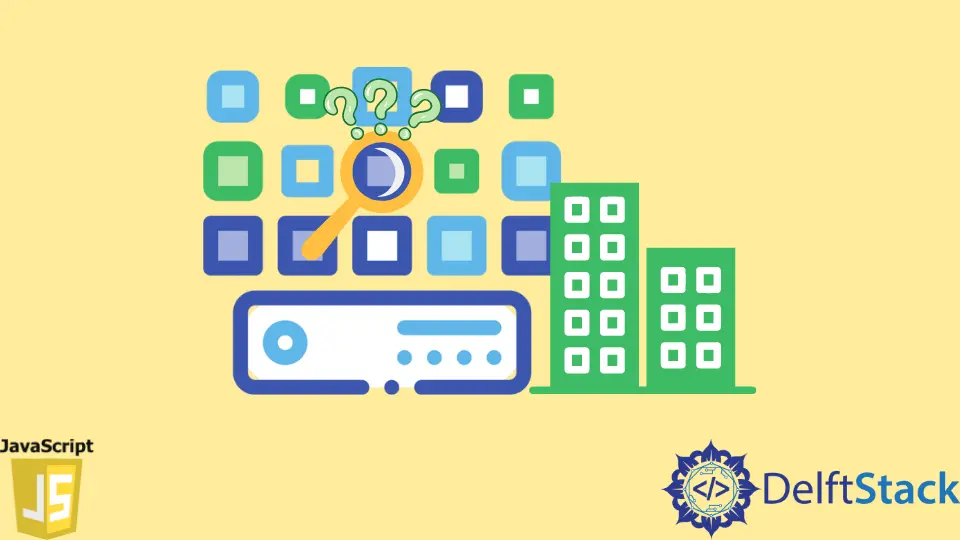
An array refers to an ordered list of values, each known as an element specified by an index. A JavaScript array can hold different values of mixed types such as string, null, or boolean, and the size of the array is not needed to specify where it is auto-growing and dynamic.
When considering the array object, it stores multiple values in a single variable and a fixed-size sequential collection of elements with the same data type. When specifying a single numeric parameter with an array constructor, the user should set the initial length of the array.
The maximum length for an array allowed is 4,294,967,295. Although an array holds data collection, it is frequently more helpful to think of an array as a collection of variables of a similar type.
Also, the JavaScript array consists of different methods and properties that will help a program execute efficiently without much coding.
Different implementations can be used in JavaScript when finding an object in an array by its property value.
Find Object in Array by Property Value Using the find()
Method
We can use the find()
method to find an object in an array of objects in JavaScript by its property value. Here, the find()
method returns the first array element provided that satisfies the given testing function.
Any values that don’t fulfill the testing function will return undefined
. The below codes indicate how to find an object by id in an array of JavaScript objects.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript Find Object In Array By Property Value</title>
</head>
<body>
<script>
var sampleArray = [
{"id": 1, "animal": "Dog"},
{"id": 2, "animal": "Cat"},
{"id": 3, "animal": "Bird"},
{"id": 4, "animal": "Fish"}
];
//getting the object by its id
var output = sampleArray.find(object => object.id === 3);
//outputs the animal to be found
document.write(output.animal);
</script>
</body>
</html>
The const
keyword is sometimes used as a common practice to declare arrays instead of var
.
Here the user needs to find the animal with the given id, and as the output, the animal was Bird
that matches the id (3) provided by the user.
Output:
If necessary, the findIndex()
method can be used in the following code to find the index of the matched object in the array.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Javascript Find Object In Array By Property Value</title>
</head>
<body>
<script>
var sampleArray = [
{"id": 1, "animal": "Dog"},
{"id": 2, "animal": "Cat"},
{"id": 3, "animal": "Bird"},
{"id": 4, "animal": "Fish"}
];
//getting the index of the object that matches the id
var objectIndex = sampleArray.findIndex(object => object.id === 3);
//getting the output as the index and the animal to be found
document.write(objectIndex + "<br>");
document.write(sampleArray[objectIndex].animal);
</script>
</body>
</html>
Here the user needs to find the animal with the given id and the index. As the output, the animal found was Bird
with its index as 2, which matches the id (3) provided by the user.
Output:
Find Object in Array by Property Value Using the filter()
Method
We can also utilize the filter()
method to create a new array filled with elements that pass through the testing function. The filter()
method does not run the function if the elements are null and do not make any changes to the original array.
The below codes indicate how to find an object by id in an array of JavaScript objects.
var animals = [
{animalName: 'Dog', ability: 'Bark'}, {animalName: 'Cat', ability: 'Meow'},
{animalName: 'Bird', ability: 'Fly'}, {animalName: 'Fish', ability: 'Swim'}
];
var animalAbility = animals.filter(function(animal) {
return animal.ability == 'Bark';
});
console.log(animalAbility);
Here the user can get the required output by entering the relevant ability
that is needed to get from the array.
Output:
Find Object in Array by Property Value Using JavaScript for
Loop
First, declare an array of objects, each object with an id and name properties. When it comes to the program’s execution, a function is created with an array, object key, and value.
The for
loop is used to iterate through the objects in an array. Each object is checked with the assigned key and the value using the equality operator(===
).
If it matches, the program returns an object. Else, it returns null
as the output.
The below codes indicate how to find an object by the key in an array of JavaScript objects. This code does not use any array methods to find an array object.
let animals =
[
{'id': 1, 'animal': 'Dog'}, {'id': 2, 'animal': 'Cat'},
{'id': 3, 'animal': 'Bird'}, {'id': 4, 'animal': 'Fish'}
]
// declaration of the function and iteration through the objects
function getObjectByKey(array, key, value) {
for (var c = 0; c < array.length; c++) {
if (array[c][key] === value) {
return array[c];
}
}
return null;
} console.log(getObjectByKey(animals, 'animal', 'Fish'))
The user can get the required output by providing the relevant key.
Output:
Find Object in Array by Property Value Using JavaScript for...in
Loop
If necessary, the for...in
loop can be used to find an array object by property value as it iterates through all property values of an object.
The below code shows how the for...in
loop can be used to find an object.
var animals = [
{'id': 1, 'animal': 'Dog'}, {'id': 2, 'animal': 'Cat'},
{'id': 3, 'animal': 'Bird'}, {'id': 4, 'animal': 'Fish'}
];
for (const c in animals) {
if (animals[c].id == 2) {
console.log(animals[c]);
}
}
Here, the user can get the output by providing the relevant id as required.
Output:
With further implementations, other methods exist to get a JavaScript object from an array of objects by the property’s value.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.