How to Destroy Object in JavaScript
-
Use the
delete
Operator to Destroy Object in JavaScript -
Use the
destroy
Method and Pass Object Instances in JavaScript -
Set Object Variable Reference to
null
With JavaScript
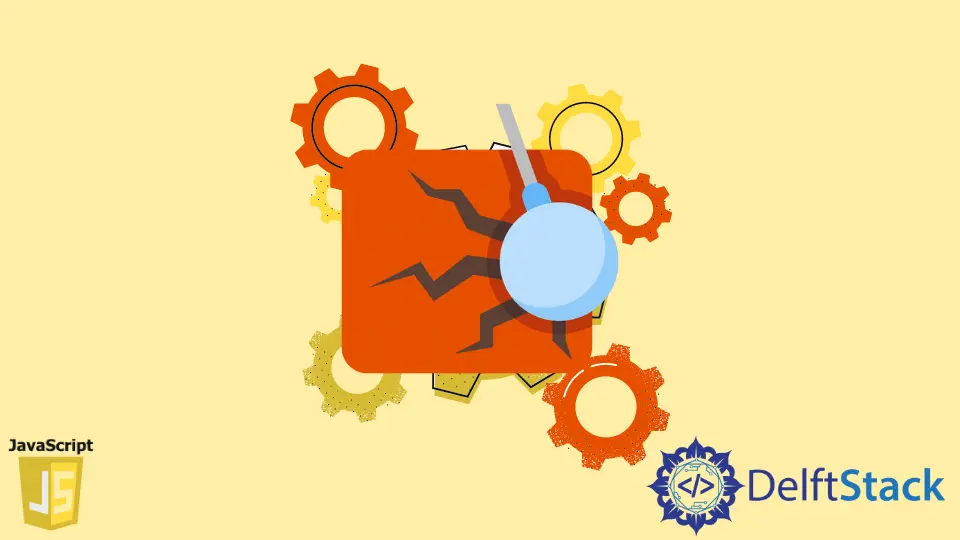
The JavaScript destroy
method does not have access to the object instances. In our examples, we will see two ways of solving the case.
In one instance, we will introduce the delete
operator, and in the next one, we will try to pass the object and its instance. Thus, we will examine how our object and its instance are set undefined
.
Use the delete
Operator to Destroy Object in JavaScript
An object consists of multiple properties as the key-value pair. As JavaScript is not introduced to any functionality that will directly vanish an object, we will have to remove the properties and corresponding values from the reference.
So, in this case, remove the name
property. Let’s see the impact of using the delete
operator.
Code Snippet:
const Employee = {
name: 'xyz',
id: 1
};
console.log(Employee.name);
delete Employee.name;
console.log(Employee.name);
Output:
It can be visualized that the later Employee.name
values as undefined
. The object still consists of the id
property.
If we remove that property, then the object is logically empty. But the object will not be uprooted from the memory.
Still, the object does not hold any property of the past.
Use the destroy
Method and Pass Object Instances in JavaScript
We will initiate a class constructor that defines the destroy
method. Here, the destroy
function takes the object to be operated and its specific instances.
Later, we will instantiate an instance for the object property, and this new instance will be operated to be null or vanish.
Code Snippet:
// Class constructor
var Class = function() {
this.destroy = function(baseObject, refName) {
delete baseObject[refName];
};
};
// instanciate
var store = {'name': 'abc', 'id': 2};
console.log(store.id);
store.id = new Class();
console.log(store.id);
store.id.destroy(store, 'id');
console.log(store.id);
Output:
Even though the store.id
still has a new Class()
initiation, the delete operation didn’t start before the parameters (object, instance) were passed in the destroy
method. This is the way how an object can tend to be null.
Set Object Variable Reference to null
With JavaScript
If a variable points to an object and sets it to null
, you won’t have access to the object. Therefore, the JavaScript garbage collector will delete the object.
The variable userName
points to an object in the following code example. We can now access the object via the userName
variable.
However, if we set the userName
variable to null
, you’ll no longer have access to the object. Resulting in deleting the object and freeing the memory.
Code:
let userName = {id: 1, position: 2} console.log('Before deletion: ', userName);
// Set the object reference to null
userName = null;
// Check if we can reach the object
console.log('After deletion: ', userName);
Output:
Before deletion: Object { id: 1, position: 2 }
After deletion: null