How to Create Array of Specific Length in JavaScript
-
Create an Array of Length Using
Array()
Constructor in JavaScript -
Create an Array of Lengths Using the
apply()
Method in JavaScript -
Create an Array of Lengths Using the
map()
Method in JavaScript -
Creates an Array of Length Using the
fill()
Method in JavaScript
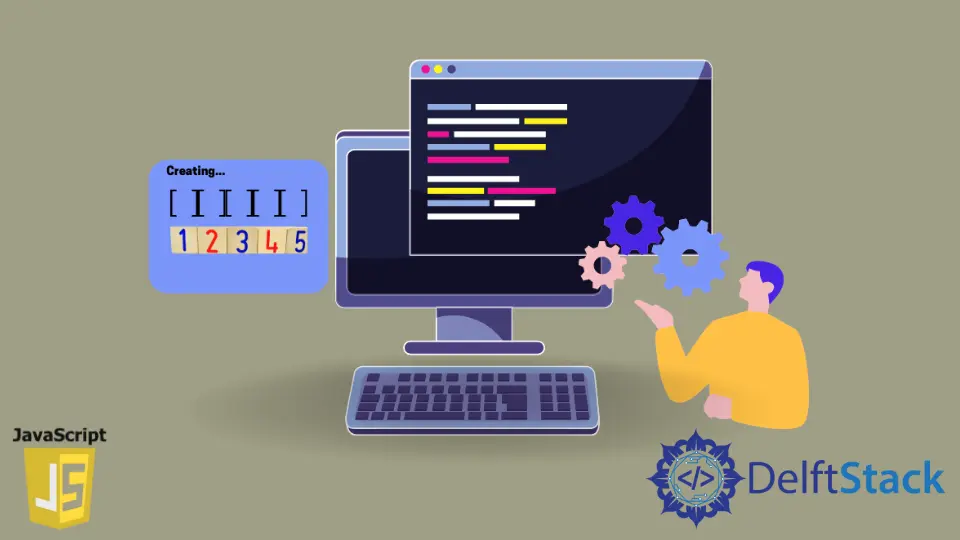
In JavaScript, you might need to create or declare an array of specific lengths and insert values you want inside the array. It can be achieved using different ways.
Below are some of the most ways to create an array of specific lengths in JavaScript. Now only use this in case you think there is a need for this because most of the time, there is no need to create or declare an array of specific lengths; you can dynamically make an array and use it.
Create an Array of Length Using Array()
Constructor in JavaScript
The first way of creating an empty array of specific lengths is by using the Array()
constructor and passing an integer as an argument to it. Since we are calling a constructor, we will be using the new
keyword.
var arr = new Array(5);
console.log(arr)
Output:
[undefined, undefined, undefined, undefined, undefined]
This way of creating an array of specific lengths is not recommended if you are using JSlint, as it might cause issues. Since this way is ambiguous and it does not just create an array of a particular length, it can also be used to do other things like creating an array containing a value as follows.
var arr_2 = new Array('5');
console.log(arr_2)
Output:
[ "5" ]
Create an Array of Lengths Using the apply()
Method in JavaScript
The Array
constructor provides a method called apply()
. Using this apply()
method, you can provide arguments to a method in the form of an array. The apply()
method takes two arguments, the first is the reference to this
argument, and the second is the array.
var myArr = Array.apply(null, Array(5));
console.log(myArr);
Output:
[undefined, undefined, undefined, undefined, undefined]
To create an empty array of a specific length, let’s say 5
, we will pass null
as the first argument, and the second is the array Array(5)
. This will create an array of length 5 and initialize each element with an undefined
value.
Alternatively, you can also do the following. Here, you can pass an array object as the second parameter and then inside it define the length of the array that you want to create, in this case, 5
.
var arr = Array.apply(null, {length: 5});
console.log(arr);
console.log(arr.length);
Output:
[undefined, undefined, undefined, undefined, undefined]
5
Create an Array of Lengths Using the map()
Method in JavaScript
Another way of creating an array of specific lengths is to use the map()
method in JavaScript. Here, the Array(5)
constructor will create an empty array of length 5. This is similar to what we saw previously. Then using the spread operator ...
, we will spread every element of the array and enclose this inside a square bracket like this [...Array(5)]
. It will create an array of length 5 where the value of each element will be undefine
. Then to initialize this array, we will make use of the map()
method.
[...Array(5)].map(x => 0);
Output:
[0, 0, 0, 0, 0]
Using the map()
method, we will take every element inside the x
variable and then add value zero to it. This will initialize all the elements of the array to zero.
Creates an Array of Length Using the fill()
Method in JavaScript
The fill()
method also works the same way as map()
does; the only thing is that the fill()
does not take any function as a parameter. It directly takes a value as an input. You can create an array of specific length Array(5)
and then fill the array with some integer value, as shown below.
Array(5).fill(0);
// [0, 0, 0, 0, 0]
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn