JavaScript Array.fill() Method
-
Syntax of JavaScript
Array.fill()
Method -
Example Codes: Replace All the Elements of an Array With
0
Using JavaScriptArray.fill()
Method -
Example Codes: Use
start_index
to Initialize Upper Triangular Matrix With JavaScriptArray.fill()
Method -
Example Codes: Use the
end_index
Parameter With JavaScriptArray.fill()
Method
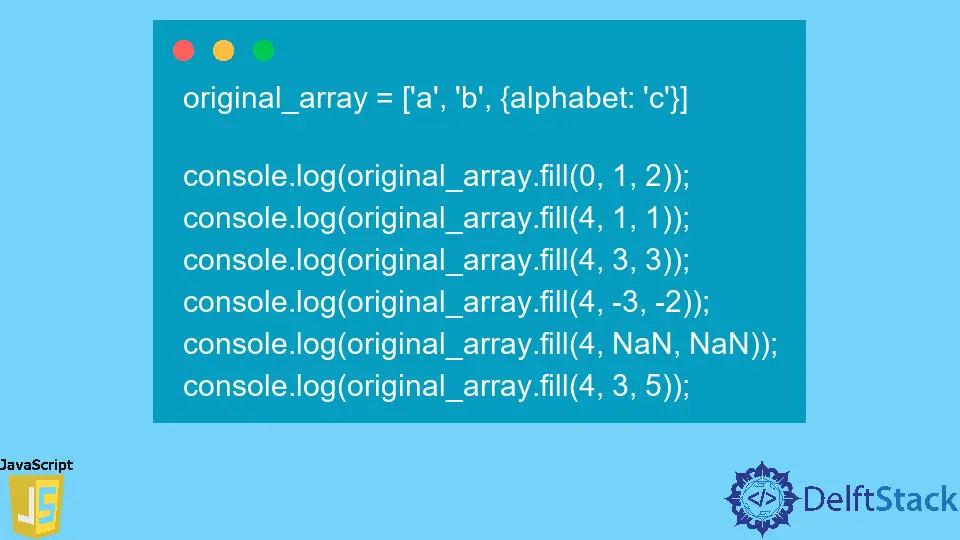
The JavaScript array.fill()
method inserts elements of an array with the same static value from a specified start index up to the end index of the array. If the start index is not specified, the value fills the elements of the array.
Syntax of JavaScript Array.fill()
Method
array.fill(fill_value);
array.fill(fill_value, start_index)
array.fill(fill_value, start_index, end_index)
Parameters
fill_value |
An object that replaces the elements of the array with the fill() method. |
start_index (optional) |
An integer that represents the index at which fill() starts inserting fill_value in the array. If it is a negative integer, fill() considers the start_index as start_index + array.length ; the default is 0. |
end_index (optional) |
An integer that represents the index at which fill() ends by inserting fill_value in the array. If it is a negative integer, fill() considers the end_index as end_index + array.length ; the default is array.length() . |
Return
The array.fill()
method returns the changed version of the original array.
Example Codes: Replace All the Elements of an Array With 0
Using JavaScript Array.fill()
Method
In this example, the value specified in the fill_value
replaces all array elements. Before altering the array with the fill()
method, slots must be assigned to it.
When no start_index
and end_index
is provided in the parameters, the JavaScript fill()
replaces all the values in the array with the fill_value
from the start_index = 0
to the end_index = array.length
.
const array = [31, 32, 34, 35];
const fill_value = 0
array.fill(fill_value);
console.log(array)
The JavaScript fill()
method considers the start_index = 0
to the end_index = array.length
, by default.
Output:
[ 0, 0, 0, 0 ]
The fill_value = 0
replaces all of the array elements above. The terminal displays the modified original array returned by the fill()
method.
Example Codes: Use start_index
to Initialize Upper Triangular Matrix With JavaScript Array.fill()
Method
const matrix = new Array(3); //initializes an empty array of 3 rows
for (let i = 0; i < matrix.length; i++) {
matrix[i] = new Array(3).fill(0); // initializes an array of size 3 and
matrix[i].fill(1,i) //replaces all the 0 with 1 from start_index
}
console.log(matrix);
Above, new Array(3)
makes an empty array of length 3, as it is necessary for the fill()
method to have slots to change its value.
The fill()
method then fills the array of three columns with 0 while the start index = i
. It replaces the 0
s in the matrix’s upper triangle with 1.
Output:
[ [ 1, 1, 1 ], [ 0, 1, 1 ], [ 0, 0, 1 ] ]
Example Codes: Use the end_index
Parameter With JavaScript Array.fill()
Method
original_array = ['a', 'b', {alphabet: 'c'}]
console.log(original_array.fill(0, 1, 2));
console.log(original_array.fill(4, 1, 1));
console.log(original_array.fill(4, 3, 3));
console.log(original_array.fill(4, -3, -2));
console.log(original_array.fill(4, NaN, NaN));
console.log(original_array.fill(4, 3, 5));
It is clear from the preceding example that:
When start_index
and end_index
parameters are equal to each other, the fill()
method does not change the original_array
.
When start_index = -3
and end_index = -2
parameters are considered as start_index= -3 + 3
and end_index= -2 + 3
, the method does not change the original_array
.
Output:
[ 'a', 0, { alphabet: 'c' } ]
[ 'a', 'b', { alphabet: 'c' } ]
[ 'a', 'b', { alphabet: 'c' } ]
[ 4, 'b', { alphabet: 'c' } ]
[ 'a', 'b', { alphabet: 'c' } ]
[ 'a', 'b', { alphabet: 'c' } ]