JavaScript Array.includes() Method
-
Syntax of
Array.includes()
-
Example 1: Use the
Array.includes()
Method With Required ParametertargetValue
-
Example 2: Use the
Array.includes()
Method With Optional ParameterstartPosition
-
Example 3: Use the
Array.includes()
Method With Case-Sensitivity
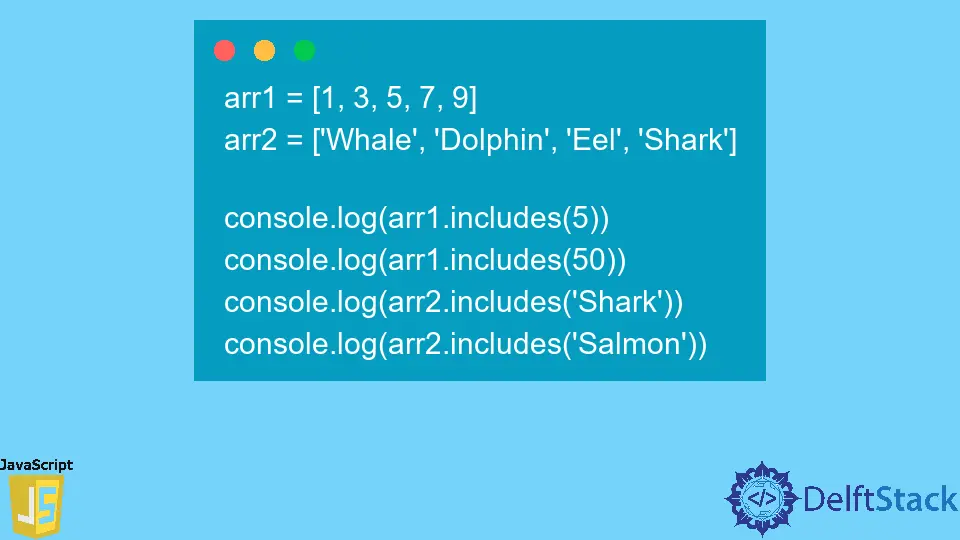
In JavaScript, Array
is considered an object that can store multiple types of values in a single variable. The JavaScript Array
object provides various methods to perform different kinds of action.
The Array.includes()
method is one of them. This method is used to check whether the array contains a specific value or not and return a Boolean value based on the result.
Syntax of Array.includes()
Array.includes(targetValue, startPosition)
Parameters
targetValue |
It refers to a specific value that will be searched. It is required. |
startPosition |
A specific index position from where you want to start searching. By default, it starts from the index position 0 . It is optional. |
Return
The Array.includes()
method returns a Boolean value. If the searched value is found in the array, it will return true
, and if it is not, it will return false
.
Important: Array.includes()
method is case-sensitive. If your array contains some string value that is in the lowercase form and you searched with the uppercase form, this method will not be able to recognize it.
Example 1: Use the Array.includes()
Method With Required Parameter targetValue
arr1 = [1, 3, 5, 7, 9]
arr2 = ['Whale', 'Dolphin', 'Eel', 'Shark']
console.log(arr1.includes(5))
console.log(arr1.includes(50))
console.log(arr2.includes('Shark'))
console.log(arr2.includes('Salmon'))
Output:
true
false
true
false
First, we searched for a value of 5
in the arr1
. This method finds the value in the array and returns true
.
We again searched for another value of 50
in the same array. This method did not find any value and returned false
.
Second, we searched with the array that contained string values. It gives a similar result, true
when it founds the value and false
when it does not.
Example 2: Use the Array.includes()
Method With Optional Parameter startPosition
arr1 = [1, 3, 5, 7, 9]
console.log(arr1.includes(5, 1))
console.log(arr1.includes(5, 3))
Output:
true
false
The array indexing starts from 0
. We take an array containing five elements whose index number is 4
.
We use the optional parameter startPosition
to specify the searching position. For the first case, we search for 5
, which is at the index position 2
.
We start searching from the index position 1
, and this method founds the result. Finally, it returns true
in the output.
For the second case, we again search for the same value. But this time, we start searching from index position 3
.
As a result, this method could not find the value in the array and returned false
.
Example 3: Use the Array.includes()
Method With Case-Sensitivity
arr1 = ['Whale', 'Dolphin', 'Eel', 'Shark']
console.log(arr1.includes('Shark'))
console.log(arr1.includes('shark'))
Output:
true
false
First, we use the same case as the parameter to check whether the value is in the array or not. As a result, the value matches the array’s value and returns true
.
We again searched for the same value in the lowercase form. Even if the spelling is the same for both, it returns false
as both are not in the same case.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn