JavaScript Array.copyWithin() Method
-
Syntax of JavaScript
Array.copyWithin()
: -
Example Code: Use the
Array.copyWithin()
Method With thetarget
Parameter -
Example Code: Use the
Array.copyWithin()
Method With thetarget
andstart
Parameters -
Example Code: Use the
Array.copyWithin()
Method With thetarget
,start
, andend
Parameters -
Example Code: Use the
Array.copyWithin()
Method With Negativetarget
,start
, andend
Parameters
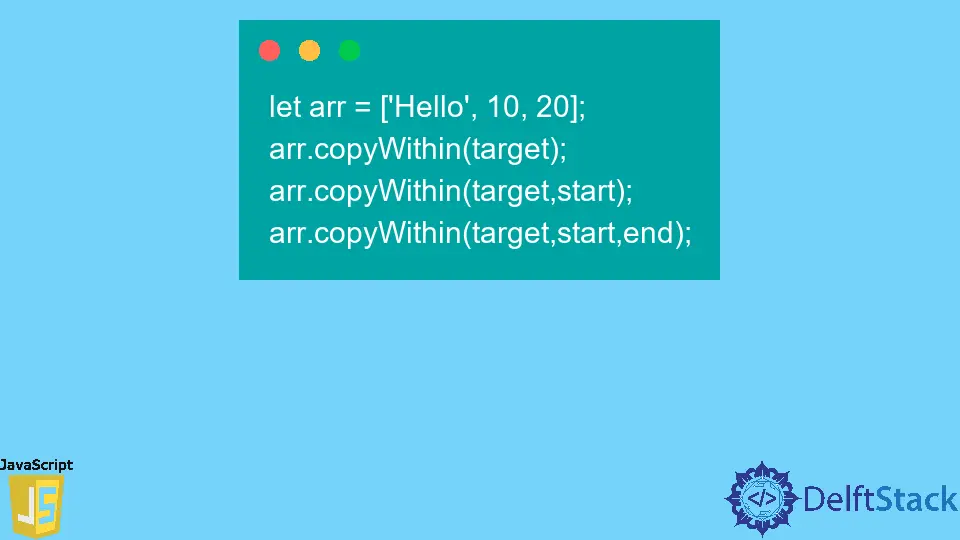
In JavaScript, the array.copyWithin()
method is the built-in library method. We can use it to copy some parts of the array at different positions and change the content of the original array.
The method only changes the content of the array, but it never changes the array’s length.
Syntax of JavaScript Array.copyWithin()
:
let arr = ['Hello', 10, 20];
arr.copyWithin(target);
arr.copyWithin(target,start);
arr.copyWithin(target,start,end);
Parameters
target |
The target is a zero-based index, and from the target index, the method will start to replace the copied content. The method will not copy anything if target > arr.length . |
start |
The start is a zero-based start index from which we must start copying the element. If we don’t pass the start , the method will start copying from the 0th index. |
end |
The end is also an optional zero-based index, up to which we must copy the elements. The method doesn’t include the element of the end index; if we remove the end , the method copies all the elements till the last index. |
Return
The Array.copyWithin()
method returns the modified array.
Example Code: Use the Array.copyWithin()
Method With the target
Parameter
In the example below, we created the array of numbers and used 2 as a target
parameter. As we haven’t passed the start
parameter, it will consider it 0, and for the end
parameter, the method will consider the last index of the array.
In the output, all array elements are replaced from the 2nd index (zero-based indexing) as the target
parameter is 2.
let array = [10, 20, 30, 40, 50, 60, 70];
array = array.copyWithin(2);
console.log(array);
Output:
[ 10, 20, 10, 20, 30, 40, 50 ]
Example Code: Use the Array.copyWithin()
Method With the target
and start
Parameters
In this example, we also used the start
parameter with the target
parameter. The value of the start
parameter is 1, which means the method will start copying the array elements from the first index and replacing array elements from the target
index, which is 3 in the below example.
let array = [10, 20, 30, 40, 50, 60, 70];
array = array.copyWithin(3,1);
console.log(array);
Output:
[ 10, 20, 30, 20, 30, 40, 50 ]
Example Code: Use the Array.copyWithin()
Method With the target
, start
, and end
Parameters
Here, we have also used the end
parameter. The value of the start
and end
parameters is 1 and 3, respectively, which means the method will copy the elements from the 1st index to the 3rd index (excluding) and start replacing them from the target
index, which is 4.
Also, users must remember that all indexes are zero-based indexes.
let array = [10, 20, 30, 40, 50, 60, 70];
array = array.copyWithin(4,1,3);
console.log(array);
Output:
[ 10, 20, 30, 40, 20, 30, 70 ]
Example Code: Use the Array.copyWithin()
Method With Negative target
, start
, and end
Parameters
We have used the negative values for the target
, start
, and end
parameters in the example below. When we use the negative parameter, the method still starts copying or replacing elements from left to right, which we can understand from the below example.
In the below example, the value of the target
parameter is -5, which means it will start replacing the elements from the last 5th index, which is the 2nd (zero-based) index from the start. Also, values of start
and end
are -3 and -1, which means the method will copy the elements from the last 3rd index to the last index (excluding).
If the user passes the value of the start
index greater than the end
index, the array.copyWithin()
method will not replace any element in the original array.
let array = [10, 20, 30, 40, 50, 60, 70];
array = array.copyWithin(-5,-3,-1);
console.log(array);
Output:
[ 10, 20, 50, 60, 50, 60, 70 ]
The Array.copyWithin()
method is compatible with all browsers, and we can use it to copy the content of the existing array to different locations in the same array.