JavaScript Array.lastIndexOf() Method
-
Syntax of
Array.lastIndexOf()
-
Example 1: Use
Array.lastIndexOf()
Method With Required ParametertargetValue
-
Example 2: Use
Array.lastIndexOf()
Method With Optional ParameterstartPosition
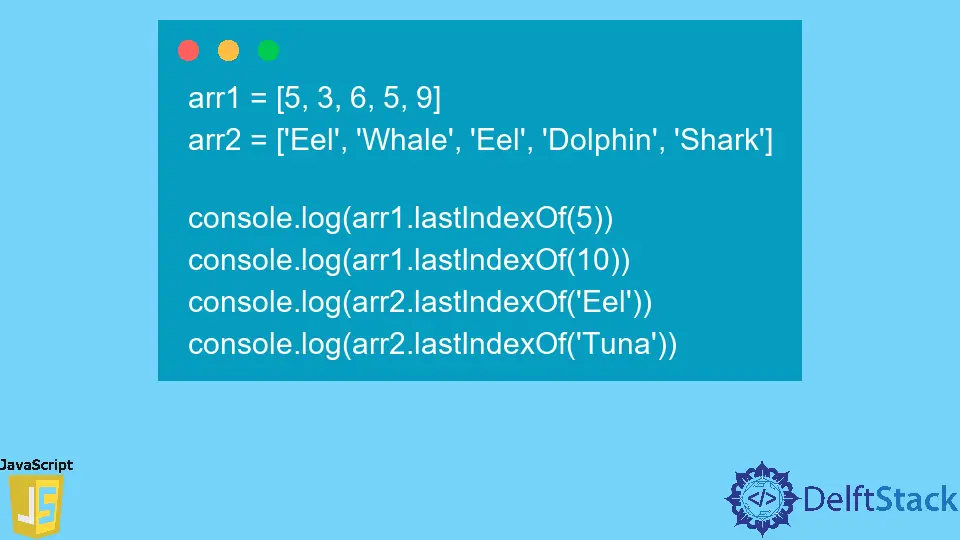
In JavaScript, Array
is considered an object that can store multiple types of values in a single variable. The JavaScript Array
object provides various methods to perform different kinds of action.
The Array.lastIndexOf()
method is one of them. This method finds the array’s last index position from a specific value.
Syntax of Array.lastIndexOf()
Array.lastIndexOf(targetValue, startPosition)
Parameters
targetValue |
It refers to a specific value that will be searched. It is required. |
startPosition |
A specific index position from where you want to start searching. By default, it starts from the array.length-1 index position. It is optional. |
Return
The Array.lastIndexOf()
method returns the last index position of a specified value. If the searched value is not found in the array, it will return -1
.
Example 1: Use Array.lastIndexOf()
Method With Required Parameter targetValue
arr1 = [5, 3, 6, 5, 9]
arr2 = ['Eel', 'Whale', 'Eel', 'Dolphin', 'Shark']
console.log(arr1.lastIndexOf(5))
console.log(arr1.lastIndexOf(10))
console.log(arr2.lastIndexOf('Eel'))
console.log(arr2.lastIndexOf('Tuna'))
Output:
3
-1
2
-1
First, we searched for a value of 5
in the arr1
. This value occurs two times in the array.
The array.lastindexOf()
starts searching from the last index position of the array and returns the index position when it finds the value in the array. In this case, this method returns index position 3
.
We again searched for another value of 50
in the same array. This time this method did not find any value and returned -1
.
Second, we searched with the array that contained string values. It gives a similar result, index position 2
when it founds the value first from the last of the array and -1
when it does not find any value.
Example 2: Use Array.lastIndexOf()
Method With Optional Parameter startPosition
arr1 = [2, 3, 9, 5, 2]
console.log(arr1.lastIndexOf(2, 3))
console.log(arr1.lastIndexOf(2, 4))
Output:
0
4
The array indexing starts from 0
. We take an array containing five elements whose index number is four
.
We use the optional parameter startPosition
to specify the searching position. For the first case, we search for 2
at the index positions 0
and 4
.
We start searching backward from the index position 3
, and this method founds the result. Finally, it returns index position 0
in the output.
For the second case, we again search for the same value. But this time, we start searching backward from index position 4
.
As a result, this method finds the value at the index position 4
and returns it.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn