JavaScript Array.filter() Method
-
Syntax of
Array.filter()
-
Example 1: Use the
Array.filter()
Method in JavaScript -
Example 2: Create Your Own
Array.filter()
Function
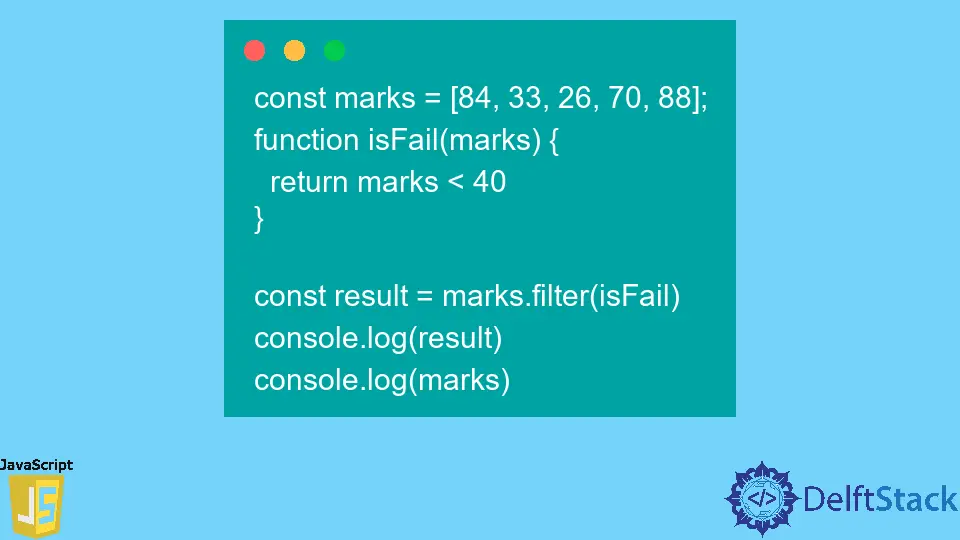
In JavaScript, Array
is considered an object that can store multiple types of values in a single variable. The JavaScript Array
object provides various methods to perform different kinds of action.
The Array.filter()
method is one of them. This method is used to find some specific elements based on the criteria from the array.
It created a shallow copy of the original array and returned it.
Syntax of Array.filter()
Array.filter(cb(element, index, arr), thisValue)
Parameters
cb |
It represents the callback function. It is used to test each element of an array. This parameter is required. |
element |
It refers to the value of the present element. It is required. |
index |
It represents the current element’s index and is optional. |
arr |
It is the array of the present element, and it is also optional. |
thisValue |
It refers to the value of the callback function. This parameter is also optional. |
Return
The Array.filter()
method returns a new array from the original array with those elements that fulfilled the criteria. If no elements match the requirements, it returns an empty array.
Example 1: Use the Array.filter()
Method in JavaScript
const marks = [84, 33, 26, 70, 88];
function isFail(marks) {
return marks < 40
}
const result = marks.filter(isFail)
console.log(result)
console.log(marks)
Output:
[ 33, 26 ]
[ 84, 33, 26, 70, 88 ]
We create a function that will return anything less than 40 from the marks
array. Later, we use the filter
method and pass this function as the parameter.
This method runs the function each time to check whether the value is less than 40. If it finds any, it simply stores that value in a new array.
Finally, return the array with the matched elements.
This method returns a shallow copy of the original array with the tested values instead of changing the original array.
Example 2: Create Your Own Array.filter()
Function
function myFilter (arr, cb){
let newArr = []
for(let i=0; i<arr.length; i++){
if(cb(arr[i], i, arr)){
newArr.push(arr[i])
}
}
return newArr
}
const marks = [84, 33, 26, 70, 88]
function isFail(marks) {
return marks < 40
}
result = myFilter(marks, isFail)
console.log(result)
console.log(marks)
Output:
[ 33, 26 ]
[ 84, 33, 26, 70, 88 ]
We create our own filter
function that works exactly as the Array.filter()
method. There is no rocket science behind this method.
All are written in simple JavaScript code. We can see how this method creates a new array instead of changing the original array elements.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn