How to Remove First Element From an Array in JavaScript
- Remove the First Element of an Array by Changing the Original Array in JavaScript
- Remove the First Element of the Array by Keeping the Original Array Unchanged in JavaScript
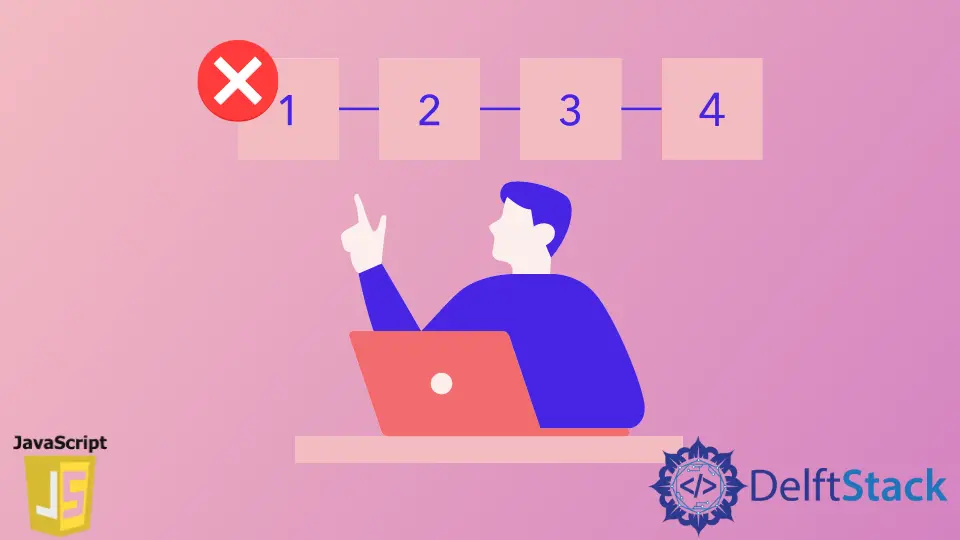
The JavaScript programming language has lots of pre-build methods that can help us make the development process faster. For any task you may think of, there will always be a pre-built method available in JavaScript to do that particular task. For example, slicing a string, inserting and deleting the elements from the array, converting one data type to another, or generating random numbers, and many more.
Similarly, while dealing with arrays, quite a few handy methods help us work and manipulate the array data structures in JavaScript. In this article, we will see three methods using which we can remove the first element from the array.
There are two types of methods available in JavaScript. One type of method will change the original array, and the second type of method will keep the original array unchanged. If you don’t have any issues if the original array gets altered, go with the splice()
and the shift
methods. But if you don’t want to change the original array, go with the filter()
and the slice
methods.
Remove the First Element of an Array by Changing the Original Array in JavaScript
splice()
method:
The splice()
method is used to remove or replace existing elements from the array. This method changes or modifies the original array. The splice()
method also returns the elements which have been removed from the array. If you want to get the deleted elements, you can also store that returned element into some variable for further use.
Syntax:
splice(start)
splice(start, deleteCount)
splice(start, deleteCount, item1)
splice(start, deleteCount, item1, item2, itemN)
The start
parameter tells us from which element to start manipulating the array. The start
can take either positive or negative values. If you use the positive values (1, 2, 3…., n) then it will start changing the array from left to right, and if you use the negative values (-1, -2, -3, …, -n) then it will start changing the array from right to left.
Then we also have deleteCount
and item1, item2, ...
parameters, both of which are optional. If you want to delete some elements from the array, you can pass the total count of the elements you want to delete as a value to the deleteCount
parameter. If passed 0
, no element will be deleted from the array. In item1, item2, ...
you can pass the elements that you want to add to the array. For more information, read the documentation of splice()
method.
Below we have an array of numbers stored inside the array
variable that consists of 5
elements. In our case, we need to delete the first element of the array. Here, we have to specify the start index and the number of elements that we want to delete. Since we want to remove the first element, therefore our starting index would be 0
and the total element that we need to delete is 1
. So, we will pass the values (0, 1)
as a parameter to the splice()
method.
let array = [1, 2, 3, 4, 5] let firstElement = array.splice(0, 1);
console.log(array, firstElement);
We are also storing the element deleted from the array into a variable called firstElement
. After removing the first element from the array, we are printing the array
and the value stored inside the firstElement
variable.
You can run the above code by pasting it into the browser’s console then you will get the output as follows.
Output:
Array(4) [ 2, 3, 4, 5 ]
Array [ 1 ]
As you can see from the output, the first element, in this case, 1
is successfully removed from the original array.
shift()
method:
Another way of removing the first element from the array is by using the shift()
method. With the splice()
method, you can add or remove any element at any index from the array, but the shift()
method is specifically used for removing the element at the 0
index only. It removes the first element (element present at index 0
) and moves the remaining elements of the array to the left.
This method also returns the element which has been removed from the array. Here, we have also created a firstElement
variable that will store the first element removed from the array.
let array = [1, 2, 3, 4, 5];
let firstElement = array.shift();
console.log(array, firstElement);
Finally, we will print the array
and firstElement
variables.
Output:
Array(4) [ 2, 3, 4, 5 ]
1
Remove the First Element of the Array by Keeping the Original Array Unchanged in JavaScript
filter()
method:
We have seen the methods that change or modify the original array; let’s now see some methods that keep the original array as it is and store the results of the operation into a new array. One such method is the filter()
method.
The filter()
method works based on condition. And based on whether the condition is true or false, it decides whether to add the elements into a new array or not.
let array_1 = [1, 2, 3, 4, 5];
let array_2 = array_1.filter((element, index) => index > 0);
console.log(array_1, array_2);
The filter()
method takes a callback function (arrow function) as an argument. This callback function is called on each element of the array. This function will give us the element
of the array and its index
.
Since we want to remove the first element, we will add a condition that the element’s index is greater than zero or not (index > 0
). If this is true, then only the filter()
function will insert the element into the new array and not otherwise. All the other elements will be inserted into the new array except for the element at index 0
(i.e, the first element).
Output:
Array(5) [ 1, 2, 3, 4, 5 ]
Array(4) [ 2, 3, 4, 5 ]
slice()
method:
The next method which keeps the original array is the slice()
method. The slice()
method cuts the array at two places. For that, we have to specify the start and end index. The start
represents the first index from where we want to start slicing the array, and the slicing will stop one element before the end
.
Any elements present within the start
and end
(including start value and stopping one element before the end) will be inserted into the new array. Both of these parameters are optional. For more information, read the slice()
method documentation.
let array_1 = [1, 2, 3, 4, 5];
let array_2 = array_1.slice(1);
console.log(array_1, array_2);
We will be passing the start
parameter only since we want to remove the first element from the array. Not specifying the end
means that all the elements of the array starting from the start
value will be included. In our case, we will pass slice(1)
. This will copy the elements [2,3,4,5]
from original array i.e array_1
into array_2
. In the end, we will print both arrays using the console.log()
method. The output of the above code is as shown below.
Output:
Array(5) [ 1, 2, 3, 4, 5 ]
Array(4) [ 2, 3, 4, 5 ]
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn