How to Convert Array to String in JavaScript
-
Use the
toString()
Method to Convert Array to String in JavaScript -
Join the Elements of the Array Using
.join()
Method in JavaScript -
Use
JSON.stringify()
to Convert Array to String in JavaScript - Use Type Coercing to Convert Array to String in JavaScript
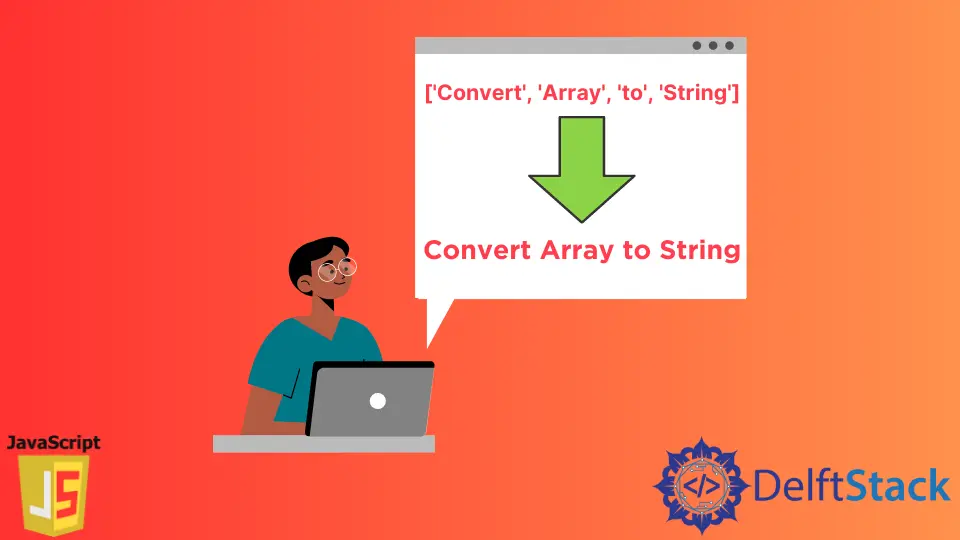
The arrays are the most common and flexible data structures you might use in your day-to-day programming life. Since you have tons of array methods to your disposal provided by the JavaScript programming language, it makes it easy for you to manipulate the arrays and perform whatever operations you want to perform.
Similarly, converting an array into a string can also be done easily with the help of predefined methods and by following some other ways. In this article, we will see the different ways in which we can convert the entire array data structure (i.e., all the elements present inside that array) into a single string.
Use the toString()
Method to Convert Array to String in JavaScript
The easiest way of converting an array into a string is by using a predefined method in JavaScript called toString()
. This method does not just work with arrays but also with various other data types. Almost anything can be converted into a string using toString()
.
You can add this at the end of the array to use this method, as shown below. It will take all the elements inside that array and concatenate them as a single string.
var arr = ['Google', 'is', 'no', '1', 'search engine'].toString();
console.log(arr);
Output:
"Google,is,no,1,search engine"
Here, if you see the output, it is a string but comma-separated. Now, if you want to remove commas from the above string, you can use the replace()
method as follows. The replace()
method takes two parameters. The first parameter is the character itself that needs to be replaced (in this case, comma, which is represented by /,
), and the second parameter decides what to replace the character with (in this case, blank space). The /
is the escape character.
arr.replace(/,/g, ' ')
Output:
"Google is no 1 search engine"
In this case, we want to remove all the commas from the string, represented by /g
.
Join the Elements of the Array Using .join()
Method in JavaScript
Another way of converting an array to a string is by using the join()
method. This method will take each element from the array and together to form a string. Here, if you directly use this method onto an array similar to that of toString()
, it will also generate a string separated by commas.
But here, you don’t need to use any other methods like the replace()
because you can directly pass any other separators as a parameter to separate the elements within the string.
var arr_1 = ['Google', 'is', 'no', '1', 'search engine'].join();
var arr_2 = ['Google', 'is', 'no', '1', 'search engine'].join('-');
var arr_3 = ['Google', 'is', 'no', '1', 'search engine'].join('##space##');
console.log(arr_1);
console.log(arr_2);
console.log(arr_3);
Output:
"Google,is,no,1,search engine"
"Google-is-no-1-search engine"
"Google##space##is##space##no##space##1##space##search engine"
Use JSON.stringify()
to Convert Array to String in JavaScript
The JSON.stringify()
method allows you to convert any JavaScript object or a value into a string. This is cleaner, as it quotes strings inside of the array and handles nested arrays properly. This method can take up to three parameters as follows.
JSON.stringify(value, replacer, space)
The value
parameter takes any value that needs to be converted into a string. This parameter is mandatory to pass. The replacer
is a function that you can pass to replace some of the elements inside the string. If you want to add white space to the output string for readability purposes, you can use the space
parameter. Both of these parameters, replacer
and space
, are optional.
var arr = JSON.stringify(['Google', 'is', 'no', '1', 'search engine']);
console.log(arr);
Output:
"[\"Google\",\"is\",\"no\",\"1\",\"search engine\"]"
In the above example, we definitely want to use a replacer function because the output we got above is not readable. The JSON.stringify()
method directly takes individual elements of the array and converts them into the string, including its structure.
Use Type Coercing to Convert Array to String in JavaScript
The last way to convert an array into a string is by using type coercion. The type coercion is a process of converting a value from one type to another. There are two types of coercion in JavaScript, implicit and explicit coercion.
The implicit coercion is when you apply various operators (+
, -
, ' '
, /
, and more) to the values of different types, and explicit coercion is when you use a function such as String()
, Number()
, etc. The example for both the type coercion is as shown below.
var str_1 = ['This', 'is', 11, 'clock'] + '';
var str_2 = String(['This', 'is', 11, 'clock']);
console.log(str_1);
console.log(str_2);
Output:
This,is,11,clock
This,is,11,clock
str_1
is an example of implicit coercion where we are just using an operator between two different types of values (one is an array, another is a string). The resulting output of this operation is a string. The str_2
is an example of explicit coercion where we have just passed the entire array inside the String()
function to convert the array into a string.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript