How to Check String Equality in JavaScript
- When to Use an Operator for String Equality in JavaScript
- Understand String Equality in JavaScript with Examples
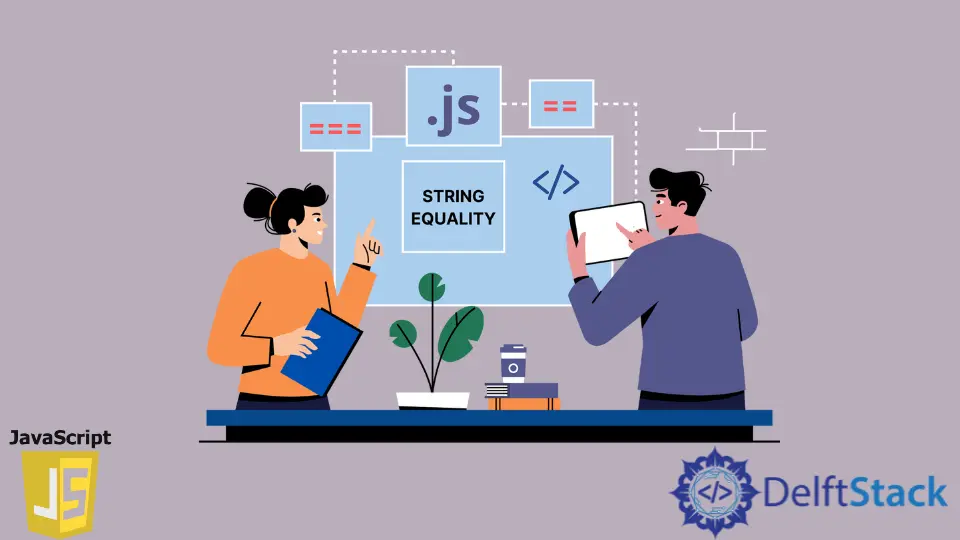
In JavaScript, there are four operators you can use for checking string equality. These operators are called the comparison operators.
- Strict equal (
===
): The Strict equal (also known as the triple equals operator) checks the value of the variable and its data type. If both of these things are equal, then it only returnstrue
, else it returnsfalse
. - Strict not equal (
!==
): This is the opposite of Strict equal. If either value or the data type does not match, it returnstrue
; otherwise,false
. - Equal (
==
): The double equal sign checks only the value of a variable. If the value matches,it returnstrue
, else it returnsfalse
. It doesn’t care about the variable type. - Not Equal (
!=
): This is the opposite of Equal (==
). It returnstrue
only if the values inside the variables don’t match, irrespective of their data type. If the values match with each other, then it returnsfalse
.
These operators are used not just for checking the string equality but also for checking the equality of other data types. Out of these four operators, let’s figure out the best one for checking the string equality depending on the use cases.
You might already have seen the 3rd and the 4th operator, i.e., ==
and !=
, which are pretty common in other programming languages as well. But the way it works in JavaScript is a little bit different than other languages.
When to Use an Operator for String Equality in JavaScript
In connection with JavaScript, below are some use cases where you can use either of the ===
or ==
operators.
- If a value in comparison could be either
true
orfalse
value, use===
and not==
. - If a value in comparison could be any of these specific values (
0
,""
, or[]
– empty array), use===
instead of==
. - In any other cases, you’re safe to use
==
. It’s not only safe, but it also simplifies your code and improves readability.
The same above rules are applicable while using the Strict not equal (!==
) and Not equal (!=
) operators as well. To learn more about the specific rules, you can read the 11.9.3 section of the ES5 specification.
Understand String Equality in JavaScript with Examples
Let’s take some of the examples and understand these things in detail.
In this example, we have taken two variables, name_1
and name_2
. Both of them take "adam"
as the string value. Now let’s apply each of the above operators and see the output we get.
Here, we have used four if
and else
statements, each representing different comparison operators. Since both the value and the data type are the same for the name_1
and name_2
variables, the triple equals or Strict equal operator (===
) will print True
as the output. And the double equal ==
will also print True
, since both the variables have the same values.
let name_1 = 'adam';
let name_2 = 'adam';
// Strict Equal
if (name_1 === name_2)
console.log('True');
else
console.log('False');
// Equal
if (name_1 == name_2)
console.log('True');
else
console.log('False');
// Strict not equal
if (name_1 !== name_2)
console.log('True');
else
console.log('False');
// Not equal
if (name_1 != name_2)
console.log('True');
else
console.log('False');
Output:
True
True
False
False
When using the Strict not equal (!==
) operator and not equal (!=
) operator, they both will print False
as the output since the value itself is the same in both the variables.
Below is another example where we will compare a string with an integer. Here, we have a variable str
with a value of "80"
, a string value. We have another variable, num
, which contains an integer value of 80
. Since both these variables have the same values with different data types, let’s see what happens when using the various comparison operators on them.
let str = '80';
let num = 80;
// Strict Equal
if (str === num)
console.log('True');
else
console.log('False')
// Equal
if (str == num) console.log('True');
else console.log('False')
// Strict not equal
if (str !== num) console.log('True');
else console.log('False')
// Not equal
if (str != num) console.log('True');
else console.log('False')
Output:
False
True
True
False
Like the first example, we also have four if
and else
statements, each representing different comparison operators.
When we are using Strict equals or triple equals (===
), we are getting False
as the output. The reason is the data type is different even though the values are the same. But when we use the double equals (==
), we get True
as the output because double equals only check for value and not the type.
And when we are using Strict not equal (!==
), we are getting True
as the output because the string value "80"
is not equal to the integer value 80
; therefore, it returns True
.
Lastly, the not equal (!=
) operator only compares the value of the two variables. The values need to be different to print True
. Since the values are the same in this case, it returns False
.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn