How to Filter String in JavaScript
-
Use the
filter()
Method With theArrow
Function to Filter String in JavaScript -
Use the
indexOf()
Method With thefilter()
Method to Filter String in JavaScript -
Use the
test()
Method With thefilter()
Method to Filter String in JavaScript -
Use the
includes()
Method With thefilter()
Method to Filter String in JavaScript
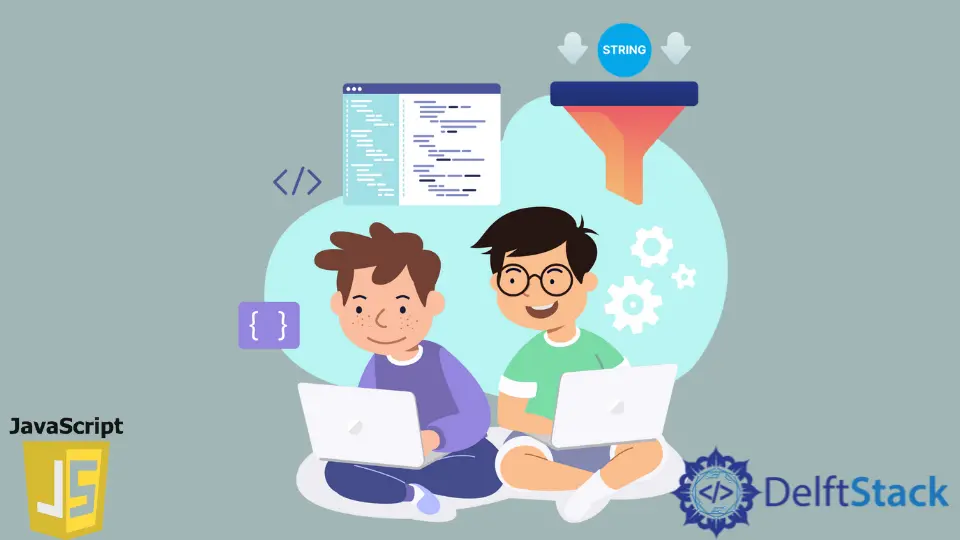
When dealing with a wide range of array elements of string and extracting preferable matches, we can depend on multiple methods. The basic filter()
method of JavaScript initiates the process, and later, based upon preference, we can select other methods.
We must note that other conventions like find()
and findIndex()
methods can also acquire matches. But these functions only return the number of indexes of the matched string; these cannot return the sequence of the given pattern to be examined with the array string.
Also, these can be used and adapted with additional conditions to retrieve the matched sequence.
Here, we will demonstrate using the filter()
method with the basic arrow operation and specific conditions. Also, we will see the use of indexOf()
, test()
, and include()
methods to get the string that satisfies the given pattern.
Not all of these methods return the string directly. Thus we will incorporate them with the filter()
method to track the array elements.
Use the filter()
Method With the Arrow
Function to Filter String in JavaScript
In this case, we will choose an array with string elements. The filter()
method will iterate through each element and see if the condition matches the condition applied with the arrow function.
Here, the task only needs a few lines of code. Let’s check on that.
Code Snippet:
var myArray = ['abadef', 'sbade', 'psadbe']
filtered = myArray.filter(x => x.length <= 5)
console.log(filtered)
Output:
As it can be seen, we have added the restriction that only string elements with lengths less than or equal to 5
will be the selected candidate. And based on that specification, the output was derived.
Use the indexOf()
Method With the filter()
Method to Filter String in JavaScript
We will work on the same categorized string array with multiple string elements. In this case, we will choose a pattern of string that we want to match with our given string, and whichever string is the superset of the pattern is the targeted string element.
The indexOf()
method typically returns the index of the string where the pattern is to be found. But in this case, we have strings as array elements, and so, whichever element will be matched, the function will start counting the index from the starting of the element’s character position.
Though we have only shown the selected element in the example, you can try rechecking the words.
Code Snippet:
var myArray = ['abadef', 'sbade', 'psadbe'];
var PATTERN = 'sad',;
filtered = myArray.filter(function(str) {
return str.indexOf(PATTERN) !== -1;
});
console.log(filtered)
Output:
Use the test()
Method With the filter()
Method to Filter String in JavaScript
The test()
function in JavaScript returns either true
or false
. As we incorporate the method with the filter()
method, we get the matched string element as the new elements for the filtered
object.
Also, a notable section is that the test()
method matches RegExp
with the elements.
Code Snippet:
var myArray = ['abadef', 'sbade', 'psadbe']
var PATTERN = /bad/,
filtered = myArray.filter(function(str) {
return PATTERN.test(str);
});
console.log(filtered)
Output:
Use the includes()
Method With the filter()
Method to Filter String in JavaScript
Here, the includes()
method returns in boolean form, but, in this case, the matching pattern can be a string and not a regular expression. Again, we will use the filter()
method to iterate through the array elements and grab the accepted cases.
So, let’s jump to the code fence.
Code Snippet:
var myArray = ['abadef', 'sbade', 'psadbe']
var PATTERN = 'ef',
filtered = myArray.filter(function(str) {
return str.includes(PATTERN);
});
console.log(filtered)
Output: