How to Get First Character From a String in JavaScript
-
Get the First Character of a String Using
slice()
in JavaScript -
Get the First Character of a String Using
charAt()
in JavaScript -
Get the First Character of a String Using
substring()
in JavaScript -
Get the First Character of a String Using
substr()
in JavaScript
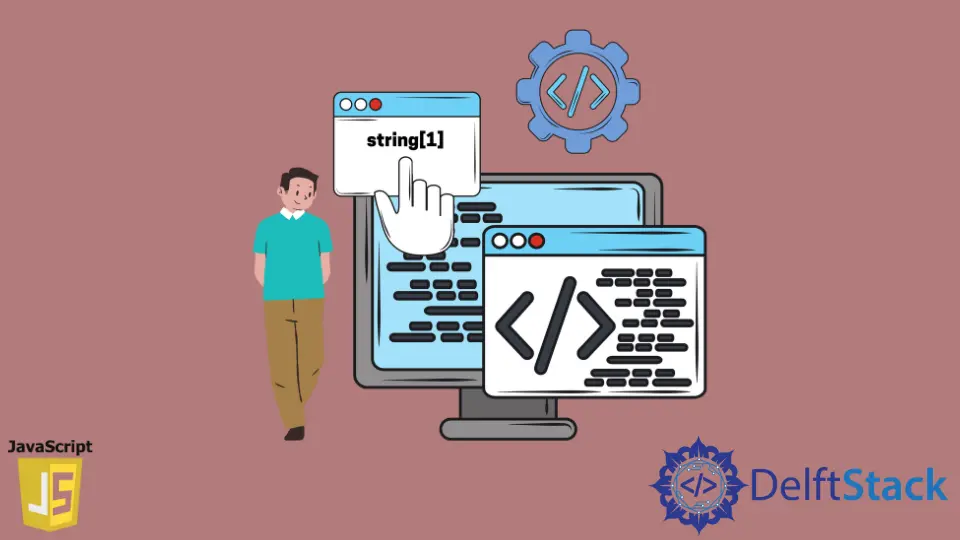
When dealing with strings, some useful methods help us work with and manipulate the data structures of strings in JavaScript.
This article will guide you in using built-in JavaScript methods to get the first character of a string.
Four methods, slice
, charAt
, substring
, and substr
, are available in JavaScript, which will return a new string without mutating the original string.
Get the First Character of a String Using slice()
in JavaScript
The slice()
method is an in-built method provided by JavaScript.
This method cuts the string at two places. This cut happens by taking two inputs, the beginning, and the ending index.
And based on that, it will return the part of the string between indexes. If only the beginning index is provided, it will return to the last character.
Syntax:
slice(beginIndex)
slice(beginIndex, endIndex)
Any character present within the begin
and end
index (including start char and stopping one char before the end) will be inserted into the new string. The ending index is an optional parameter.
For more information, read the slice()
method documentation.
const inputString = 'Welcome to JavaScript tutorial';
const outputString1 = inputString.slice(0, 1);
const outputString2 = inputString.slice(4, -2);
console.log(outputString1);
console.log(outputString2);
If we call slice(1, 8)
, this will copy the character W
from the original string, inputString
into outputString1
. The fascinating part here is that when you give a negative index, the input parameter will be considered str.length + index
.
The above code will give you the below output.
Output:
"W"
"ome to JavaScript tutori"
Get the First Character of a String Using charAt()
in JavaScript
This method gets the single UTF-16 code unit present at the specified index. This method does not mutate or modify the original string.
Syntax:
charAt(index)
Any character present at the index will be inserted into the new string. If no index is provided, 0
will be considered by default.
For more information, read the charAt()
method documentation.
const inputString = 'Welcome to JavaScript tutorial';
const outputString1 = inputString.charAt(0);
const outputString2 = inputString.charAt(11);
console.log(outputString1);
console.log(outputString2);
If we call charAt(0)
, this will copy the character W
from the original string, inputString
into outputString1
. The above code will give you the below output.
Output:
"W "
"J"
Get the First Character of a String Using substring()
in JavaScript
The substring()
method is an in-built method provided by JavaScript.
This method cuts the string at two places. This cut happens by taking two inputs, the start index, and the end index.
And based on that, it will return the part of the string between indexes. If only the start index is provided, it will return the end of the string.
Syntax:
substring(indexStart);
substring(indexStart, indexEnd);
Any character present within the start
and end
index (including start char and stopping one char before the end) will be inserted into the new string. The end index is optional.
For more information, read the substring()
method documentation.
The sole difference between substring()
and slice()
is in the arguments.
If indexStart
is greater than indexEnd
, the substring
method swaps two arguments. Meaning a string is still returned.
The slice
method, in this case, returns an empty string. The substring method treats both arguments as 0
if any of the arguments are negative
or NaN
.
slice
also treats NaN arguments as 0
. But when negative values are passed, it counts down from the end of the string to find the indexes.
const inputString = 'Hello World!';
const outputString = inputString.substring(0, 1);
console.log(inputString);
console.log(outputString);
If we call substring(0, 1)
, this will copy the character H
from the original string, inputString
into outputString
. In the end, we will print both strings using the console.log()
method.
The above code will give you the below output.
Output:
"Hello World!"
"H"
Get the First Character of a String Using substr()
in JavaScript
The substr()
method is an in-built method provided by JavaScript.
This method cuts the string at two places. This cut happens by taking two inputs, the start index and a total number of characters after that.
And based on that, it will return the part of the string between the index and total number. If only the start index is provided, it will return the end of the string.
Syntax:
substr(start);
substr(start, length);
Any character present within the start
index and length
will be inserted into the new string. Length is an optional parameter here.
For more information, read the substr()
method documentation.
The sole difference between substring()
and substr()
is in the arguments.
The substring()
methods represent the starting and ending indexes as arguments. In contrast, the substr()
represents the starting index and the number of characters in the returned string as an argument.
const inputString = 'Hello World!';
const outputString1 = inputString.substr(0, 1);
const outputString2 = inputString.substr(1, 0);
console.log(inputString);
console.log(outputString1);
console.log(outputString2);
If we call substr(0, 1)
, this will copy the character H
from the original string, inputString
into outputString1
. The fascinating part here is that swapping the input parameter will not copy any character since the length is 0
.
The above code will give you the below output.
Output:
"Hello World!"
"H"
""
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn