How to Get Last Character of a String in JavaScript
-
Extracting Last Character of String With
substr(-1)
Function of JavaScript -
Using the
charAt
Function to Get the Last Character of a String -
Using
slice(-1)
Function to Get the Last Character - Getting the Last Index of the String
-
Using the
split()
Function of Javascipt - Summary
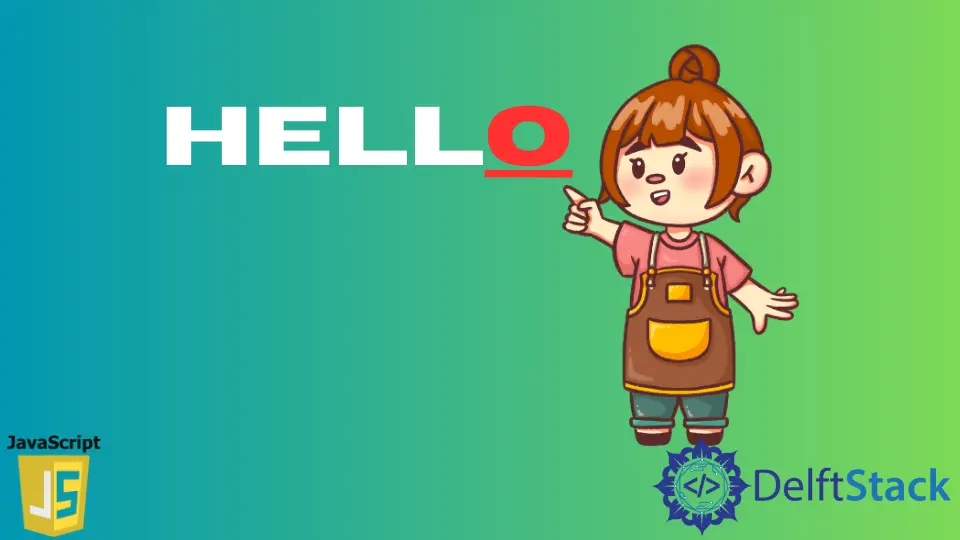
Before we proceed, let us know how JavaScript understands a string
object. Unlike other programming languages like C, Java, etc., javascript considers a string
as an array of characters. Hence, we can perform array operations on a string to get its last character. We can use the following inbuilt functions of javascript can be used for this purpose.
Extracting Last Character of String With substr(-1)
Function of JavaScript
substr()
is similar to the most commonly used function substring()
. We can use either of these functions to get a portion of a string based on the parameter values. substr()
accepts two parameters, one the index
(starting from 0
) from where we need to fetch the substring and the length of the substring. For getting the last character of a string, we can pass -1 as a parameter to the substr()
function as shown by the following code snippet.
var a = 'hello';
console.log(a.substr(-1));
Output:
o
We can also use the substring()
function to get the last character of a string. It takes two parameters, the start index and the end index. Hence, to get the last letter from the string object, we define the starting index as string length - 1
and the end index as the length of the string.
var a = 'hello';
console.log(a.substring(a.length - 1, a.length));
Output:
o
Using the charAt
Function to Get the Last Character of a String
The charAt()
function returns the character
at the specified position of a string. It takes the position of the intended character as a parameter. Hence to fetch the last character of the string, we can pass length - 1
(as the string index starts from 0
) of the string as a parameter to it. Refer to the following usage.
var a = 'hello';
console.log(a.charAt(a.length - 1));
Output:
o
Using slice(-1)
Function to Get the Last Character
The slice()
function is also a commonly used method to operate on strings. It is similar to the substring()
function for the arguments accepted by it. Both the functions accept two parameters, the start index and the end index. They differ in their behavior as slice(-1)
gives the last character of the string. Hence, it resembles substr()
for getting the last character of the string.
var a = 'hello';
console.log(a.slice(-1));
Output:
o
Getting the Last Index of the String
We can get the last character of a string in javascript using the conventional methods too. As javascript considers a string object as an array of characters, we can retrieve the last element of that array using the string[length - 1]
syntax. It is similar to dealing with a character array in other programming languages like C and Java.
var a = 'hello';
console.log(a[a.length - 1]);
Output:
o
Using the split()
Function of Javascipt
With the split()
function, we can break a string into various substrings. The function splits the string based on the delimiter that we pass as a parameter to it. For getting the last character of the string, we can use ""
(empty character) as a delimiter and get all the characters individually in an array. Then we need to get the last element of the array. We can do that by using the array[array.length]
, the commonly used array syntax, to fetch the element at a given index from an array.
var a = 'hello';
console.log(a.split('')[a.length - 1]);
Output:
o
Summary
Summarizing all the methods we discussed above to extract the last character from a string, we can club them all in a single code snippet. The substr(-1)
and the slice(-1)
are the easiest to use among the others.
var a = 'hello';
console.log(a.substr(-1));
console.log(a.substring(a.length-1, a.length));
console.log(a.charAt(a.length - 1));
console.log(a.slice(-1));
console.log(a[a.length-1]);
console.log(a.split("")[a.length - 1]);
Output:
o
o
o
o
o
o
Note
- None of the functions discussed in this article alter the original string. Hence, they can be used safely throughout the application without any side effects.
- All the functions are supported across all browsers, including the older versions of Internet Explorer.