How to Convert a String Into a Date in JavaScript
-
Use the
new Date()
Function to Convert String to Date in JavaScript -
Use the
Date.parse()
Function to Convert String to Date in JavaScript - Split String and Convert It to Date in JavaScript
- Conclusion
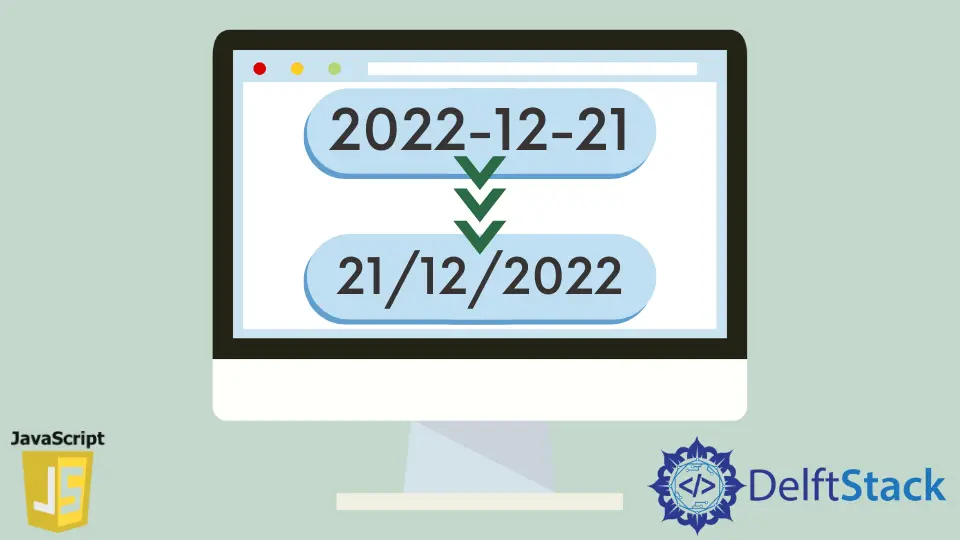
At times it is needed to convert a string into a date format. The string may be a date value stored as a string in the database or a value returned from the API. In either case, this string value cannot be directly used in date pickers or input type date. Hence, the string will need to be converted to a date object to display in the HTML UI. Being in a string format, it is not possible to perform date-specific operations. Hence we need to convert the string to a date object. Here we list down a few ways to convert a string to a date:
new Date()
Date.parse()
- Split and convert to Date
Use the new Date()
Function to Convert String to Date in JavaScript
The most commonly used way to convert a string to date is with the new Date()
function. new Date()
takes arguments in various forms described below but returns a date object.
- No parameters as the argument.
new Date()
will return the current system date and time, including the timezone information in the local system time, if no parameter is passed to thenew Date()
function. - Passing date object as the argument. The
new Date()
will return a date object as depicted by the date string passed as an argument. - Passing a string date. The most interesting part of the
new Date('2018-12-3')
function is that it can convert a date passed in a string format as an argument into a date format. We will discuss this in detail.
Passing String Date as an Argument
When we pass a date in string format to the new Date()
, it is converted into a date object. The string format needs to be YYYY-MM-DD
, the ISO 8601 format, for it to work. Other date formats may not be converted to a date object by this method. For example, refer to the code as follows:
new Date('2021-05-23');
new Date('2020/2/29');
new Date('2020-14-03');
new Date('14-02-2021');
Output:
Sun May 23 2021 05:30:00 GMT+0530 (India Standard Time)
Sat Feb 29 2020 00:00:00 GMT+0530 (India Standard Time)
Invalid Date
Invalid Date
As you see from the above examples, the only accepted string format for the date is 2021-05-23
and 2020/2/29
. The new Date()
function can process strings in the format YYYY-MM-DD
or YYYY/MM/DD
. If we have a different string format that does not conform to ISO 8601 standards, the new Date()
will not be able to parse the date and will return as an Invalid date
.
Remarks
new Date()
can be used to convert the string into a date only if it qualifies the ISO 8601 formatYYYY-MM-DD hh:mm:ss
.- For a different format that cannot be comprehended by
new Date()
, it is better to split the string and pass them as arguments into thenew Date()
, as introduce later in this article. new Date()
can also convert a string of the format01 Mar 2020 12:30
or evenMM DD YYYY HH:mm
. In case we are using a value passed from the backend via API for conversion, we should make sure that the format passed is as understood by thenew Date()
function, and it is good to have a check thatnew Date()
returns a valid date object. Else the code may break.
Use the Date.parse()
Function to Convert String to Date in JavaScript
Date.parse()
is an alternate option to convert the string date. It returns a numeric value instead of a date object. Hence it will require further processing if you expect a date object. It converts the parsed date to a number representing the milliseconds that have passed since 1-Jan-1970 midnight time. It is similar to the timestamp format with the difference that instead of seconds, Date.parse()
returns a milliseconds value.
Date.parse('2020-11-21')
Date.parse('2019-01-01T12:30:00.000Z')
Date.parse('20-11-2021')
Date.parse('11-20-2021')
Output:
1605916800000
1546345800000
NaN
1637346600000
It is worthy to note that Date.parse()
is the same as new Date()
when it comes to the type of input values it can accept but offers a better hand checking if the date is of a valid format. Such a method comes in handy, especially when we are dealing with an API response value. In such cases, we may need to perform a check to ensure the value returned by the backend conforms to the date format accepted by the Date.parse()
or even the new Date()
function. Just an isNaN()
check can help identify and safely land into the date conversion method.
let stringsFromAPI = ['2020-11-21', '20-11-2021'];
stringsFromAPI.forEach((d) => {
if (!isNaN(Date.parse(d))) {
console.log(new Date(d));
}
})
Output:
Sat Nov 21 2020 05:30:00 GMT+0530 (India Standard Time)
Note that here the date 20-11-2021
is of the format DD-MM-YYYY
. Hence, it will not be understood by the Date.parse()
function nor by the new Date()
function. Hence if the stringsFromAPI
was a value coming from the backend, then the above function will convert only those dates that conform to the acceptable format. The !isNaN(Date.parse(d))
silently rejects the improper date value and logs the value that are successful in conversion.
Remarks
Date.parse()
is similar to thenew Date()
except for the return type, which makes it suitable for checking if a date value is of a correct format and can also be used to assign a date by using thedateObj.setTime(Date.parse(DateString))
.- Compared to the Unix Time Stamp, the
Date.parse()
returns milliseconds that can be used for precision comparison of dates even without converting them to actual date objects withnew Date()
. Date.parse()
internally uses thenew Date()
for parsing of date, hence the formats accepted by thenew Date()
function will also be supported in theDate.parse()
function.
Split String and Convert It to Date in JavaScript
Both the Date.parse()
and the new Date()
functions are designed based on the ISO 8601 extended date format. Sometimes, if the date does not conform to the expected format, we will have to manually work it out by splitting the date string, extracting the values, and convert them to a date object. Surprisingly, the new Date()
also supports the date parameters be passed as arguments, and we get the date object as output.
The syntax for creating a date from arguments:
new Date(yearValue, IndexOfMonth, dayValue, hours, minutes, seconds)
Where,
yearValue
: Should conform to the ISO 8061 YYYY format. For example, 2021. If we specify a value in theYY
format, it will take it wrongly. For example, just mentioning 21 for2021
will be taken as 1921 instead of 2021.IndexOfMonth
: Starts with the index 0. Hence, subtract 1 from the Month value. For example, for March, the value is 3, butmonthIndex
will be 2 (i.e. 3-1 = 2). Index of the month should typically lie in the0-11
rangedayValue
: Indicates the day of the month. It should lie in the range of 1-31, depending on the number of days in a month. For example: for 21-05-2021, the day value is 21hours
: The hour of the day. For example 10 O’clock.minutes
: The minutes past an hour.seconds
: Holds the second’s value past a minute.
The following is a function that takes a string having a custom date format - DD-MM-YYYYTHH:mm:SS
and returns a date object.
function convertFromStringToDate(responseDate) {
let dateComponents = responseDate.split('T');
let datePieces = dateComponents[0].split('-');
let timePieces = dateComponents[1].split(':');
return (new Date(
datePieces[2], (datePieces[1] - 1), datePieces[0], timePieces[0],
timePieces[1], timePieces[2]))
}
convertFromStringToDate('21-03-2020T11:20:30')
Output:
Sat Mar 21 2020 11:20:30 GMT+0530 (India Standard Time)
Conclusion
The most commonly used new Date()
function is pretty useful to convert a string date into a date object, provided the string conforms to the ISO 8601 format. As compared to the new Date()
, the Date.parse()
function differs in a way that it returns a numeric value containing the milliseconds since January first of 1970 that elapsed till the date passed as a string argument. A different date format can be converted by splitting the date string and passing the date components as arguments to the new Date()
function.