How to POST a JSON Object Using Fetch API in JavaScript
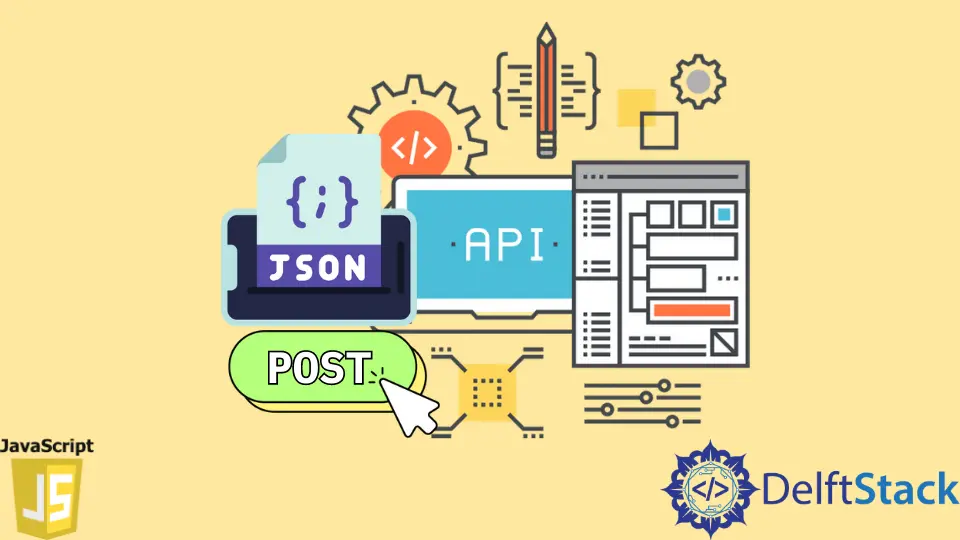
The Fetch API’s fetch()
method allows you to send and receive HTTP responses and requests to and from the server.
This article will discuss how to send the JSON object as a POST request to the server using the fetch()
method.
POST a JSON Object Using Fetch API in JavaScript
The Fetch API allows you to access and modify the HTTP requests and responses. The Fetch API provides the fetch()
method that will enable you to fetch resources asynchronously across the network.
The fetch()
method takes two parameters, url
and options
.
The url
is the endpoint to which the request is made. The options
is an object with many properties, but this is an optional parameter.
You can learn more about the fetch()
method on MDN.
let jsonObj = {
firstname: 'Adam',
lastname: 'Smith',
mob: [111111, 888888, 999999],
address: {state: 'Texas', country: 'USA', pincode: 123456}
};
Here, we will first create an object called jsonObj
. This object will contain properties, such as the first name, last name, address, etc.
We aim to send this object to the server by making a POST request using the fetch()
method.
We will use the httpbin.org
, a simple HTTP request & response service, to act as a back-end server. Since we don’t have any back-end server, we will use this service and access its /post
endpoint to make a POST request.
But if you have a back-end server with a POST route, you can use that server.
First, we will create a variable url
to store the URL of the httpbin
back-end server. After that, we also have to define some HTTP headers.
For this, we have to create an object. Here, we have defined only two headers inside the object, Accept
and Content-Type
, which have a value of application/json
.
We are setting the values of these headers as application/json
because we will be sending the JSON data over the server. In the end, we will store the object that contains headers inside a variable called headers
, as shown below.
Now that we have the jsonObj
, url
, and headers
, let’s create a function makePostRequest()
to perform a POST request and send the jsonObj
to the server. We will call the fetch()
method inside this function.
This method will return a promise. It might take some time to resolve; we have to wait until it is resolved using the await
keyword.
We will also make the makePostRequest()
function an asynchronous function using the async
keyword.
let url = 'https://httpbin.org/post';
let headers = {
'Accept': 'application/json',
'Content-Type': 'application/json'
};
async function makePostRequest(url, requestType, headers) {
await fetch(
url,
{method: requestType, headers: headers, body: JSON.stringify(jsonObj)},
)
.then(async rawResponse => {
var content = await rawResponse.json()
console.log(content);
});
}
makePostRequest(url, 'POST', headers);
The makePostRequest()
will take three parameters, the url
, requestType
, and headers
. We have already created the url
and headers
variables that we will pass to this function as a parameter whenever we call this function.
The requestType
represents the type of request we want to perform. Since we wish to make a POST request, we will pass POST
as a string to this function.
As we have already seen, the fetch()
method will take two parameters. So, we directly pass the url
as a first parameter to the fetch()
method.
We will pass another object with three properties method
, headers
, and body
inside the second parameter. As a value to the method
property will give the requestType
variable, we tell the browser to perform a POST request.
Inside the headers
property, we will assign the headers we have created. Here comes the important part; inside the body
property, we have to pass the jsonObj
we have created.
Whenever we send or receive any data to and from the server, the data should be in a specific format. The format in which the data is transferred between the server and client is either JSON or XML format.
But the most popular out of these two is the JSON string format. We can’t directly send the object jsonObj
created by performing a POST request.
We first have to convert this object into a string, and then we will be able to send this object to the server.
To convert the jsonObj
into a string, we must pass this object to the JSON.stringify()
method. This method will convert the object into a string and assign it the body
property.
After the promise returned by the fetch()
method is resolved, we will print the response into the console. We will use the then()
function to do this.
The then()
function takes a callback function as an input. The fetch()
method will return a raw response, then it will pass this raw response to the then()
function, and the callback function inside then()
will take it as an argument.
Before printing the response, we will first convert it into JSON using the json()
function. This might take some time to convert the rawResponse
back to JSON; we will use the await
keyword.
Since we use await
, we must make the callback function inside then()
asynchronous using the async
keyword.
Finally, we will store the result inside the content
variable, and then we will print the data stored inside the variable on the console as shown in the above image.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn