How to Convert CSV to JSON in JavaScript
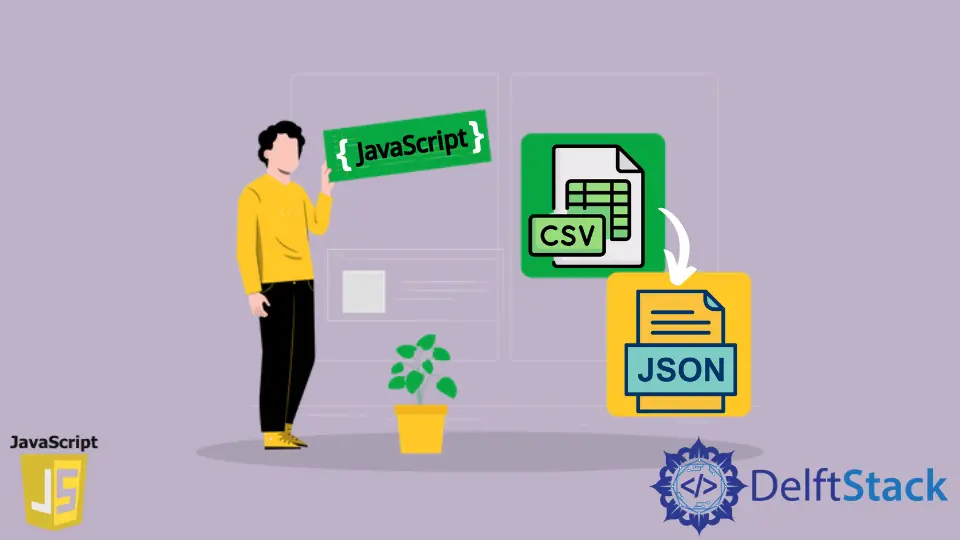
A CSV is a comma-separated value file with a .csv
extension that allows data to be stored in a tabular format. Today’s article will teach how to convert data from a CSV file to JavaScript Object Notation (JSON) without using a third-party npm
package.
The main difference from the normal conversion is that commas can separate the values of each line, and as we know, the different columns are also separated by commas.
Convert CSV to JSON in JavaScript
In this approach, we put the contents of the CSV file into an array and split the contents of the array based on a delimiter. All rows from the CSV file are converted to JSON objects that are added to the resulting array, which is then converted to JSON, and a corresponding JSON output file is generated.
- Read the CSV file with the default
fs npm
package. (It’s an optional step, you can directly provide the data in array format) - Convert the data to a string and split it into an array.
- Create a separate array of headers.
- For each remaining data row, do the following:
4.1. Create an empty object to store resultant values from the current row.
4.2. Declare a stringcurrentArrayString
as the current array value to change the delimiter and store the generated string in a new stringstring
.
4.3. If we encounter double-quotes ("
), we keep the commas; otherwise, we replace them with a pipe|
.
4.4. Continue adding the characters we’re looping through to strings.
4.5. Divide the string using the|
and store the values in a property array.
4.6. If the value for each header contains multiple data separated by commas, we store it as an array; otherwise, the value is saved directly.
4.7. Add the generated object to our result array. - Convert the resulting array to JSON, print the data, or store it in a JSON file.
The following code shows the implementation of the mentioned above in JavaScript.
const fs = require('fs');
csv = fs.readFileSync('username.csv')
const array = csv.toString().split('\n');
/* Store the converted result into an array */
const csvToJsonResult = [];
/* Store the CSV column headers into seprate variable */
const headers = array[0].split(', ')
/* Iterate over the remaning data rows */
for (let i = 1; i < array.length - 1; i++) {
/* Empty object to store result in key value pair */
const jsonObject = {}
/* Store the current array element */
const currentArrayString = array[i] let string = ''
let quoteFlag = 0
for (let character of currentArrayString) {
if (character === '"' && quoteFlag === 0) {
quoteFlag = 1
} else if (character === '"' && quoteFlag == 1)
quoteFlag = 0
if (character === ', ' && quoteFlag === 0) character = '|'
if (character !== '"') string += character
}
let jsonProperties = string.split('|')
for (let j in headers) {
if (jsonProperties[j].includes(', ')) {
jsonObject[headers[j]] =
jsonProperties[j].split(', ').map(item => item.trim())
} else
jsonObject[headers[j]] = jsonProperties[j]
}
/* Push the genearted JSON object to resultant array */
csvToJsonResult.push(jsonObject)
}
/* Convert the final array to JSON */
const json = JSON.stringify(csvToJsonResult);
console.log(json)
The output of the above code will vary depending on the input data provided.
Output:
[
{
"name": "John",
"quote": "Hello World"
},
{
"name": "Alma",
"quote": "Have a nice day"
}
]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn