在 JavaScript 中将 CSV 转换为 JSON
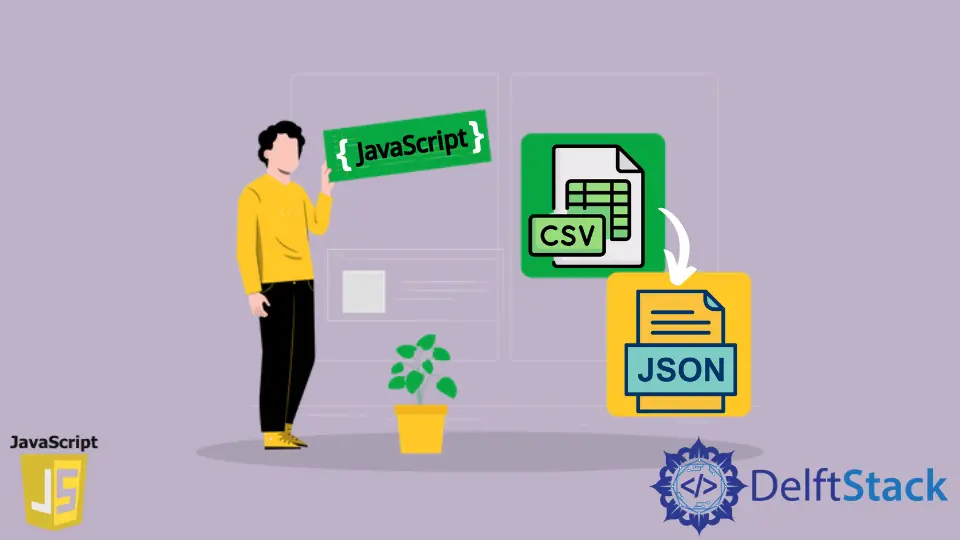
CSV 是一个逗号分隔值文件,扩展名为 .csv
,允许以表格格式存储数据。今天的文章将介绍如何在不使用第三方 npm
包的情况下将数据从 CSV 文件转换为 JavaScript Object Notation (JSON)。
与普通转换的主要区别在于逗号可以分隔每一行的值,而我们知道,不同的列也是用逗号分隔的。
在 JavaScript 中将 CSV 转换为 JSON
在这种方法中,我们将 CSV 文件的内容放入一个数组中,并根据分隔符拆分数组的内容。CSV 文件中的所有行都转换为 JSON 对象,这些对象添加到结果数组中,然后将其转换为 JSON,并生成相应的 JSON 输出文件。
- 使用默认的
fs npm
包读取 CSV 文件。 (这是一个可选步骤,你可以直接提供数组格式的数据) - 将数据转换成字符串,拆分成数组。
- 创建一个单独的标题数组。
- 对于每个剩余的数据行,执行以下操作:
4.1. 创建一个空对象来存储当前行的结果值。
4.2. 将字符串currentArrayString
声明为当前数组值以更改分隔符并将生成的字符串存储在新字符串string
中。
4.3. 如果遇到双引号 ("
),我们保留逗号;否则,我们用管道|
替换它们。
4.4. 继续将我们循环的字符添加到字符串中。
4.5.使用|
分割字符串并将值存储在属性数组中。
4.6.如果每个头的值包含多个用逗号分隔的数据,我们将其存储为数组;否则,直接保存该值。
4.7.将生成的对象添加到我们的结果数组中。 - 将结果数组转换为 JSON,打印数据,或将其存储在 JSON 文件中。
下面的代码展示了上面提到的在 JavaScript 中的实现。
const fs = require('fs');
csv = fs.readFileSync('username.csv')
const array = csv.toString().split('\n');
/* Store the converted result into an array */
const csvToJsonResult = [];
/* Store the CSV column headers into seprate variable */
const headers = array[0].split(', ')
/* Iterate over the remaning data rows */
for (let i = 1; i < array.length - 1; i++) {
/* Empty object to store result in key value pair */
const jsonObject = {}
/* Store the current array element */
const currentArrayString = array[i] let string = ''
let quoteFlag = 0
for (let character of currentArrayString) {
if (character === '"' && quoteFlag === 0) {
quoteFlag = 1
} else if (character === '"' && quoteFlag == 1)
quoteFlag = 0
if (character === ', ' && quoteFlag === 0) character = '|'
if (character !== '"') string += character
}
let jsonProperties = string.split('|')
for (let j in headers) {
if (jsonProperties[j].includes(', ')) {
jsonObject[headers[j]] =
jsonProperties[j].split(', ').map(item => item.trim())
} else
jsonObject[headers[j]] = jsonProperties[j]
}
/* Push the genearted JSON object to resultant array */
csvToJsonResult.push(jsonObject)
}
/* Convert the final array to JSON */
const json = JSON.stringify(csvToJsonResult);
console.log(json)
上述代码的输出将根据提供的输入数据而有所不同。
输出:
[
{
"name": "John",
"quote": "Hello World"
},
{
"name": "Alma",
"quote": "Have a nice day"
}
]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn