在 JavaScript 中检查字符串是否是有效的 JSON 字符串
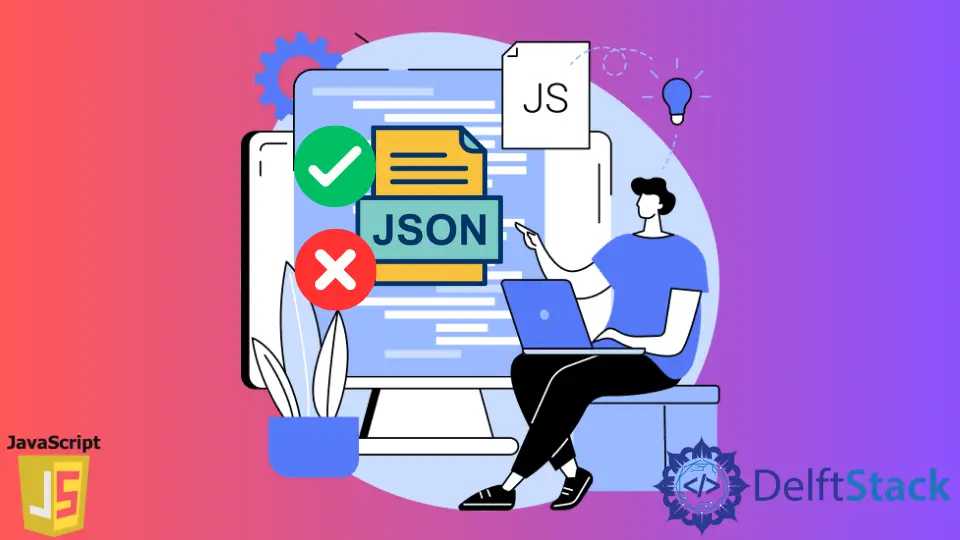
本文将介绍如何在不使用 JavaScript 中的 try...catch
的情况下检查给定字符串是否是有效的 JSON 或 JavaScript Object Notation 字符串。让我们从 JSON 字符串的定义开始,以及它如何帮助我们简化工作流程。
JSON 是一种独立于语言的文本格式,用于结构化数据,与 XML 非常相似;它可以由字符串、整数、浮点数等、布尔值、数组或用大括号括起来的 Java 对象(键值对)组成
JSON 和 Javascript 对象之间的区别
JavaScript 中的对象是这样的:
object = {
name: 'Haseeb',
age: 31,
city: 'New York',
male: true
}
当该对象以 JSON 格式保存时,它看起来像这样:
{"name":" Haseeb ", "age":31, "city":"New York", "male":true}
当我们从文本文件中检索对象时,它会被转换为 JSON 字符串格式:
{ 'name': ' Haseeb ', 'age': 31, 'city': 'New York', 'male': true }
我们可以看到 JSON 格式如何是人类可读的并且更容易被语言操作。
除此之外,它还有很多优点; JSON 除了易于读写之外,还可以与所有语言集成,并且由于其详细的结构,现在可以方便地使用解析。
JSON 通常用于应用程序编程接口 (API),因为它主要用于将数据传输到服务器或从服务器传输到 Web 或移动应用程序,反之亦然。
通过使用 JSON 的 parse 函数,我们现在可以从 JSON 字符串创建对象,这是一个如何完成的示例:
string = '{"name":"John", "age":31, "city":"New York", "male":true}';
object = JSON.parse(string);
console.log(object);
输出:
{
name: 'John',
age: 31,
city: 'New York',
male: true
}
正如预期的那样,解析函数将所有内容缩减为对象的键和值(JSON 对象),这就是 JSON 字符串现在已成为在系统之间传输数据的约定的原因。
在 JavaScript 中检查 JSON 字符串的有效性
try...catch
用于处理可能在给定 JSON 字符串中弹出的任何潜在错误,而不会停止程序的执行。字符串可能有错误的语法、缺少参数,或者可能是错误的拆分参数。
下面是我们如何使用 try...catch
检查 JSON 字符串的有效性:
try {
object = JSON.parse(json);
} catch (error) {
is_json = false;
console.log('Invalid JSON string');
}
让我们尝试给我们的代码这个字符串:
json = '{"name":"John","city""New York"}';
输出:
Invalid JSON string
正如我们所看到的,我们的 try...catch
并没有告诉我们字符串有什么问题。它只是告诉我们存在一些问题。
为了深入了解 JSON 字符串中的问题,我们可以使用自定义函数来检测(如果不是全部)在不使用 try...catch
的情况下在字符串中弹出的一些错误。
isJSON = function(json) {
// Nested Count function only to be used for counting colons and commas
countCharacter =
function(string, character) {
count = 0;
for (var i = 0; i < string.length; i++) {
if (string.charAt(i) == character) { // counting : or ,
count++;
}
}
return count;
}
json = json.trim(); // remove whitespace, start and end spaces
// check starting and ending brackets
if (json.charAt(0) != '{' || json.charAt(json.length - 1) != '}') {
console.log('Brackets {} are not balanced')
return false
}
// else this line will check whether commas(,) are one less than colon(:)
else if (!(countCharacter(json, ':') - 1 == countCharacter(json, ','))) {
console.log('comma or colon are not balanced');
return false;
} else {
json = json.substring(1, json.length - 1); // remove first and last
// brackets
json = json.split(','); // split string into array, and on each index there
// is a key-value pair
// this line iterate the array of key-value pair and check whether key-value
// string has colon in between
for (var i = 0; i < json.length; i++) {
pairs = json[i];
if (pairs.indexOf(':') == -1) { // if colon not exist in b/w
console.log('No colon b/w key and value');
return false;
}
}
}
return true;
};
让我们使用这两个 JSON 字符串并找出它们的错误。
json = ' {"name":"John","city""New York"} ';
json2 = ' {"name":"John","city":"New York" ';
输出:
comma or colon are not balanced
false
Brackets {}
are not balanced
false
这段代码很弱,因为它对于一些错误的情况是正确的,所以我们找到了一种混合方法来合并上层函数和 try...catch
代码,以通过一些调试获得绝对答案。
isJSON = function(json) {
is_json = true; // true at first
// Try-catch and JSON.parse function is used here.
try {
object = JSON.parse(json);
} catch (error) {
is_json = false;
console.log('might be a problem in key or value\'s data type');
}
if (!is_json) {
countCharacter =
function(string, character) {
count = 0;
for (var i = 0; i < string.length; i++) {
if (string.charAt(i) == character) { // counting : or ,
count++;
}
}
return count;
}
json = json.trim(); // remove whitespace, start and end spaces
if (json.charAt(0) != '{' || json.charAt(json.length - 1) != '}') {
console.log('Brackets {} are not balanced')
}
else if (!(countCharacter(json, ':') - 1 == countCharacter(json, ','))) {
console.log('comma or colon are not balanced');
} else {
json =
json.substring(1, json.length - 1); // remove first and last brackets
json = json.split(',');
for (var i = 0; i < json.length; i++) {
pairs = json[i];
if (pairs.indexOf(':') == -1) { // if colon not exist in b/w
console.log('No colon b/w key and value');
}
}
}
}
return is_json;
};
json = ' {"name":"John", "birth":"1986-12-14", "city":5a0} ';
输出:
might be a problem in key or value's data type
false
这个函数被赋予了与前一个函数相同的调试级别,但这次的答案将是绝对的。