JavaScript で文字列が有効な JSON 文字列かどうかをチェックする
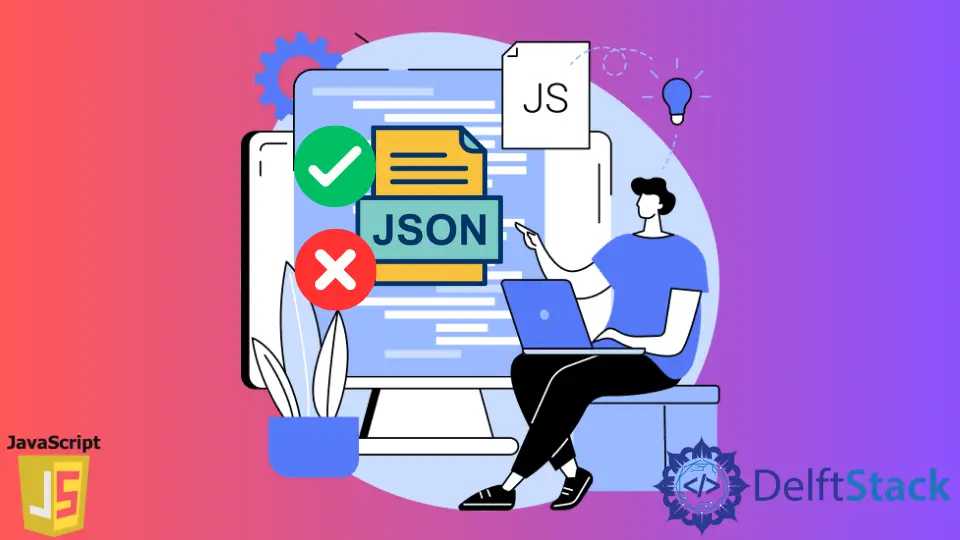
この記事では、JavaScript で try...catch
を使用せずに、特定の文字列が有効な JSON または JavaScript ObjectNotation 文字列であるかどうかを確認する方法について説明します。JSON 文字列の定義と、それがワークフローを容易にするのにどのように役立つかから始めましょう。
JSON は、XML と非常によく似たデータの構造化に使用される言語に依存しないテキスト形式です。文字列、数値 int、float など、ブール値、配列、または中括弧で囲まれた Java オブジェクト(キーと値のペア)で構成できます。
JSON オブジェクトと Javascript オブジェクトの違い
JavaScript のオブジェクトは次のようになります。
object = {
name: 'Haseeb',
age: 31,
city: 'New York',
male: true
}
そのオブジェクトを JSON 形式で保存すると、次のようになります。
{"name":" Haseeb ", "age":31, "city":"New York", "male":true}
オブジェクトをテキストファイルから取得すると、JSON 文字列形式に変換されます。
{ 'name': ' Haseeb ', 'age': 31, 'city': 'New York', 'male': true }
JSON 形式が人間が読める形式であり、言語が操作しやすいことがわかります。
それ以外にもさまざまな利点があります。JSON は、読み取りと書き込みが簡単であるだけでなく、すべての言語と統合でき、詳細な構造により、解析を便利に使用できるようになりました。
JSON は、主にサーバーと Web またはモバイルアプリケーション間でデータを転送したり、その逆を行ったりするために使用されるため、アプリケーションプログラミングインターフェイス(API)で一般的に使用されます。
JSON の解析関数を使用することで、JSON 文字列からオブジェクトを作成できるようになりました。その方法の例を次に示します。
string = '{"name":"John", "age":31, "city":"New York", "male":true}';
object = JSON.parse(string);
console.log(object);
出力:
{
name: 'John',
age: 31,
city: 'New York',
male: true
}
予想どおり、解析関数はすべてをオブジェクトのキーと値(JSON オブジェクト)にトリミングします。これが、JSON 文字列がシステム間でデータを転送するための規則になっている理由です。
JavaScript で JSON 文字列の有効性を確認する
try...catch
は、プログラムの実行を停止せずに、指定された JSON 文字列にポップアップする可能性のある潜在的なエラーを処理するために使用されます。文字列の構文に誤りがあるか、パラメータが欠落しているか、または分割引数が間違っている可能性があります。
try...catch
を使用して JSON 文字列の有効性を確認する方法は次のとおりです。
try {
object = JSON.parse(json);
} catch (error) {
is_json = false;
console.log('Invalid JSON string');
}
コードに次の文字列を付けてみましょう。
json = '{"name":"John","city""New York"}';
出力:
Invalid JSON string
ご覧のとおり、try...catch
では、文字列の何が問題になっているのかはわかりません。それは単に問題があることを示しています。
JSON 文字列の何が問題になっているのかを理解するために、try...catch
を使用せずに文字列に表示されるエラーをすべてではないにしても検出する、カスタムメイドの関数を使用する場合があります。
isJSON = function(json) {
// Nested Count function only to be used for counting colons and commas
countCharacter =
function(string, character) {
count = 0;
for (var i = 0; i < string.length; i++) {
if (string.charAt(i) == character) { // counting : or ,
count++;
}
}
return count;
}
json = json.trim(); // remove whitespace, start and end spaces
// check starting and ending brackets
if (json.charAt(0) != '{' || json.charAt(json.length - 1) != '}') {
console.log('Brackets {} are not balanced')
return false
}
// else this line will check whether commas(,) are one less than colon(:)
else if (!(countCharacter(json, ':') - 1 == countCharacter(json, ','))) {
console.log('comma or colon are not balanced');
return false;
} else {
json = json.substring(1, json.length - 1); // remove first and last
// brackets
json = json.split(','); // split string into array, and on each index there
// is a key-value pair
// this line iterate the array of key-value pair and check whether key-value
// string has colon in between
for (var i = 0; i < json.length; i++) {
pairs = json[i];
if (pairs.indexOf(':') == -1) { // if colon not exist in b/w
console.log('No colon b/w key and value');
return false;
}
}
}
return true;
};
2つの JSON 文字列を使用して、それらの欠点を見つけましょう。
json = ' {"name":"John","city""New York"} ';
json2 = ' {"name":"John","city":"New York" ';
出力:
comma or colon are not balanced
false
Brackets {}
are not balanced
false
このコードは、一部の誤ったケースに当てはまるため弱いため、上位関数と try...catch
コードの両方をマージして、デバッグで絶対的な答えを得るハイブリッドアプローチを見つけます。
isJSON = function(json) {
is_json = true; // true at first
// Try-catch and JSON.parse function is used here.
try {
object = JSON.parse(json);
} catch (error) {
is_json = false;
console.log('might be a problem in key or value\'s data type');
}
if (!is_json) {
countCharacter =
function(string, character) {
count = 0;
for (var i = 0; i < string.length; i++) {
if (string.charAt(i) == character) { // counting : or ,
count++;
}
}
return count;
}
json = json.trim(); // remove whitespace, start and end spaces
if (json.charAt(0) != '{' || json.charAt(json.length - 1) != '}') {
console.log('Brackets {} are not balanced')
}
else if (!(countCharacter(json, ':') - 1 == countCharacter(json, ','))) {
console.log('comma or colon are not balanced');
} else {
json =
json.substring(1, json.length - 1); // remove first and last brackets
json = json.split(',');
for (var i = 0; i < json.length; i++) {
pairs = json[i];
if (pairs.indexOf(':') == -1) { // if colon not exist in b/w
console.log('No colon b/w key and value');
}
}
}
}
return is_json;
};
json = ' {"name":"John", "birth":"1986-12-14", "city":5a0} ';
出力:
might be a problem in key or value's data type
false
この関数には前の関数と同じデバッグレベルが与えられますが、今回は絶対的な答えになります。