How to Check if a String Is a Valid JSON String in JavaScript
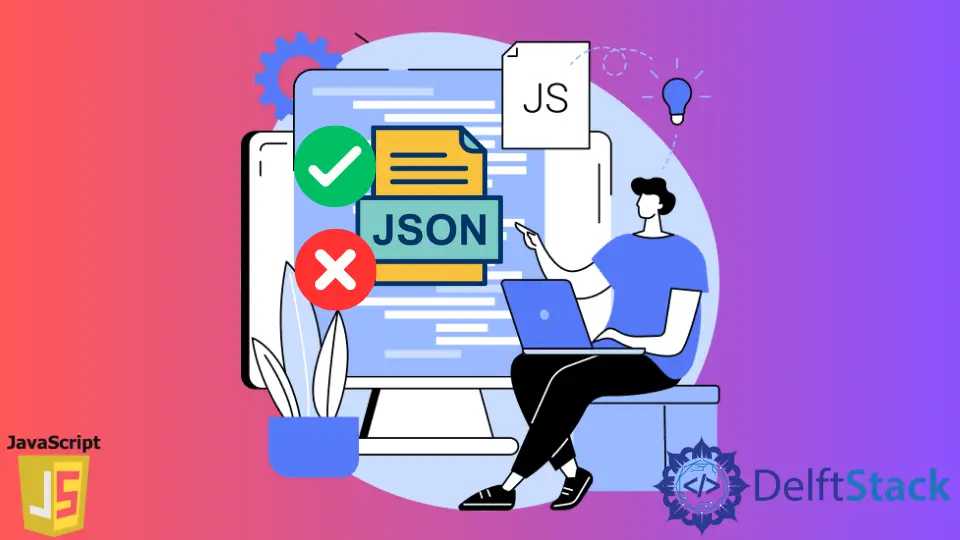
This article will teach how to check if a given string is a valid JSON or JavaScript Object Notation string without using try...catch
in JavaScript. Let’s begin with the definition of JSON string and how it helps us ease our workflow.
JSON is a language-independent text format used for structuring data very similar to XML; it can consist of strings, numbers int, float, etc., Boolean, array, or Java objects (key-value pair) enclosed in curly brackets
Difference Between JSON and Javascript Objects
This is how object in JavaScript looks like:
object = {
name: 'Haseeb',
age: 31,
city: 'New York',
male: true
}
When that object is saved in JSON format, it looks like this:
{"name":" Haseeb ", "age":31, "city":"New York", "male":true}
When we retrieve object back from text file it gets converted into JSON string format:
{ 'name': ' Haseeb ', 'age': 31, 'city': 'New York', 'male': true }
We can see how JSON format is human readable and easier for languages to manipulate.
It has various advantages other than that; JSON, aside from being easy to read and write, can integrate with all of the languages, and parsing can now be used conveniently because of its detailed structure.
JSON is commonly used for Application programming interfaces (APIs) since it is mainly used to transfer data to and from a server to a web or mobile application and vice versa.
By using JSON’s parse function, we can now create objects from JSON string, here’s an example of how it’s done:
string = '{"name":"John", "age":31, "city":"New York", "male":true}';
object = JSON.parse(string);
console.log(object);
Output:
{
name: 'John',
age: 31,
city: 'New York',
male: true
}
As expected, the parse function trims everything down to the object’s key and value (JSON object) which is why JSON string has now become a convention to transfer data to and from between systems.
Check the Validity of JSON String in JavaScript
try...catch
is used to handle any potential error that may pop up in the given JSON string without halting the program’s execution. The string could have faulty syntax, missing parameters, or perhaps a wrong splitting argument.
Here’s how we can check the validity of a JSON string using try...catch
:
try {
object = JSON.parse(json);
} catch (error) {
is_json = false;
console.log('Invalid JSON string');
}
Let us try giving our code this string:
json = '{"name":"John","city""New York"}';
Output:
Invalid JSON string
As we can observe, our try...catch
doesn’t tell us what is wrong with the string. It simply tells us there exists some problem.
To get an insight into what is wrong in the JSON string, we may turn to our custom-made function that detects, if not all, some errors that pop up in a string without the use of try...catch
.
isJSON = function(json) {
// Nested Count function only to be used for counting colons and commas
countCharacter =
function(string, character) {
count = 0;
for (var i = 0; i < string.length; i++) {
if (string.charAt(i) == character) { // counting : or ,
count++;
}
}
return count;
}
json = json.trim(); // remove whitespace, start and end spaces
// check starting and ending brackets
if (json.charAt(0) != '{' || json.charAt(json.length - 1) != '}') {
console.log('Brackets {} are not balanced')
return false
}
// else this line will check whether commas(,) are one less than colon(:)
else if (!(countCharacter(json, ':') - 1 == countCharacter(json, ','))) {
console.log('comma or colon are not balanced');
return false;
} else {
json = json.substring(1, json.length - 1); // remove first and last
// brackets
json = json.split(','); // split string into array, and on each index there
// is a key-value pair
// this line iterate the array of key-value pair and check whether key-value
// string has colon in between
for (var i = 0; i < json.length; i++) {
pairs = json[i];
if (pairs.indexOf(':') == -1) { // if colon not exist in b/w
console.log('No colon b/w key and value');
return false;
}
}
}
return true;
};
Let’s use the two JSON strings and find their faults.
json = ' {"name":"John","city""New York"} ';
json2 = ' {"name":"John","city":"New York" ';
Output:
comma or colon are not balanced
false
Brackets {}
are not balanced
false
This code is weak since it is true for some false cases, so we find a hybrid approach to merge both the upper function and try...catch
code to get an absolute answer with some debugging.
isJSON = function(json) {
is_json = true; // true at first
// Try-catch and JSON.parse function is used here.
try {
object = JSON.parse(json);
} catch (error) {
is_json = false;
console.log('might be a problem in key or value\'s data type');
}
if (!is_json) {
countCharacter =
function(string, character) {
count = 0;
for (var i = 0; i < string.length; i++) {
if (string.charAt(i) == character) { // counting : or ,
count++;
}
}
return count;
}
json = json.trim(); // remove whitespace, start and end spaces
if (json.charAt(0) != '{' || json.charAt(json.length - 1) != '}') {
console.log('Brackets {} are not balanced')
}
else if (!(countCharacter(json, ':') - 1 == countCharacter(json, ','))) {
console.log('comma or colon are not balanced');
} else {
json =
json.substring(1, json.length - 1); // remove first and last brackets
json = json.split(',');
for (var i = 0; i < json.length; i++) {
pairs = json[i];
if (pairs.indexOf(':') == -1) { // if colon not exist in b/w
console.log('No colon b/w key and value');
}
}
}
}
return is_json;
};
json = ' {"name":"John", "birth":"1986-12-14", "city":5a0} ';
Output:
might be a problem in key or value's data type
false
This function is given the same debugging level as the previous function, but the answer will be absolute this time.