How to Format JSON in JavaScript
-
Use the
JSON.stringify()
Method to Format a JSON Object in JavaScript -
Use the
JSON.stringify()
andJSON.parse()
Methods to Format a JSON String in JavaScript
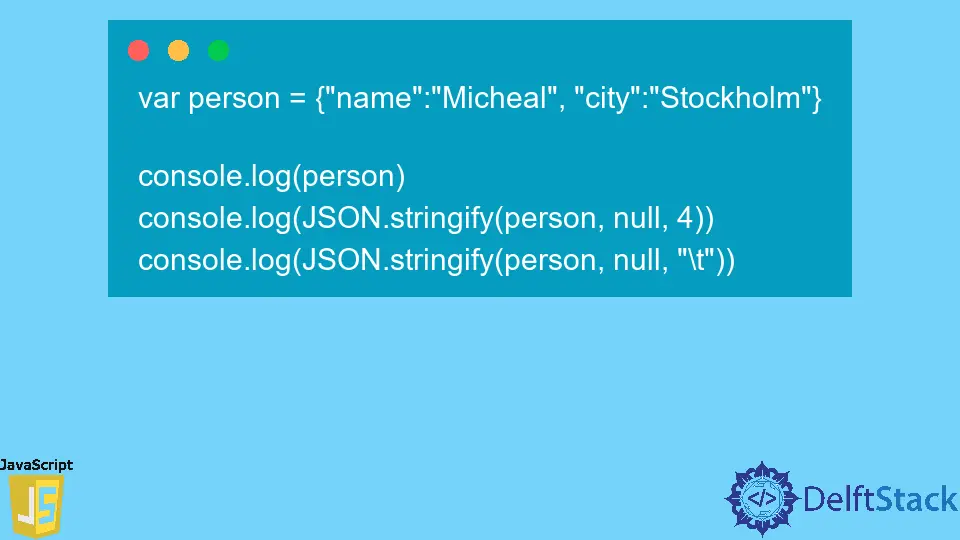
This article will introduce a few methods to format a JSON value in JavaScript.
Use the JSON.stringify()
Method to Format a JSON Object in JavaScript
We can use the JSON.stringify()
method to format JSON in JavaScript. The method is used to convert a JavaScript value to a JSON string.
A JavaScript value can be anything like an object, array, string, etc. This section will use the method to format a JSON object.
First, let’s understand the method syntactically. The syntax of the JSON.stringify()
method is shown below.
JSON.stringify(value, replacer, space)
Here, value
is any JavaScript values like objects, arrays, etc., that are to be converted to a string. The replacer
parameter modifies the way value
is stringified.
The space
parameter sets the white space in the stringified output.
It can be of two types: number or string. The parameters replacer
and value
are optional.
We can use the JSON.stringify()
method to format a raw JSON object and set the space
parameter.
For the replacer
option, we can set null
. This will stringify the keys of the object.
For example, create a variable person
and assign a raw object.
var person = {'name': 'Micheal', 'city': 'Stockholm'}
Next, use the JSON.stringify()
method, where the person
object is the value. Set null
as the second parameter and the number 4
as the third parameter.
Again, replicate the method but this time change 4
with "\t"
. Finally, print the source object and the two stringified objects.
Example Code:
var person = {'name': 'Micheal', 'city': 'Stockholm'}
console.log(person)
console.log(JSON.stringify(person, null, 4))
console.log(JSON.stringify(person, null, "\t"))
Output:
{ name: 'Micheal', city: 'Stockholm' }
{
"name": "Micheal",
"city": "Stockholm"
}
{
"name": "Micheal",
"city": "Stockholm"
}
In the above example, we printed the raw JSON object at first. It is not formatted.
Next, we used the number 4
as space
to format the object. As a result, each key-value pair in the object are formatted to new lines.
The number 4
determines that there are four whitespaces before the start of each line. Similarly, we also used the string "\t"
as space
in the third call.
It also formatted the object but with some extra spaces.
This way, we can use the JSON.stringify()
method to format a JSON object in JavaScript.
Use the JSON.stringify()
and JSON.parse()
Methods to Format a JSON String in JavaScript
We can use the combination of the JSON.stringify()
and JSON.parse()
methods to format the JSON string in JavaScript. This approach is similar to how we format the JSON object, as we did in the first method.
The only difference is we will parse the string with the JSON.parse()
method before using it as a value
in the JSON.stringify()
method.
The JSON.parse()
method will convert the string into a JSON object. Then, the object is used as the parameter for the JSON.stringify()
method.
For example, we can use the same person
object to format the JSON string. However, this time, wrap the person
variable with a quote, which will be a JSON string.
Look at the following example.
var person = '{"name":"Micheal", "city":"Stockholm"}'
console.log(person)
console.log(JSON.stringify(JSON.parse(person), null, 4))
console.log(JSON.stringify(JSON.parse(person), null, '\t'))
Output:
{"name":"Micheal", "city":"Stockholm"}
{
"name": "Micheal",
"city": "Stockholm"
}
{
"name": "Micheal",
"city": "Stockholm"
}
Here, we have parsed a JSON string into an object using the JSON.parse()
method. Then, we used the JSON.stringify()
method to format the object.
This way, you can use the combination of the JSON.parse()
and JSON.stringify()
methods to format a JSON string in JavaScript.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn