How to Generate Formatted and Easy-To-Read JSON in JavaScript
- Generate Formatted and Easy-To-Read JSON in JavaScript
-
Generate Formatted and Easy-To-Read JSON Using the
parse()
Method -
Generate Formatted and Easy-To-Read JSON Using the
stringify()
Method
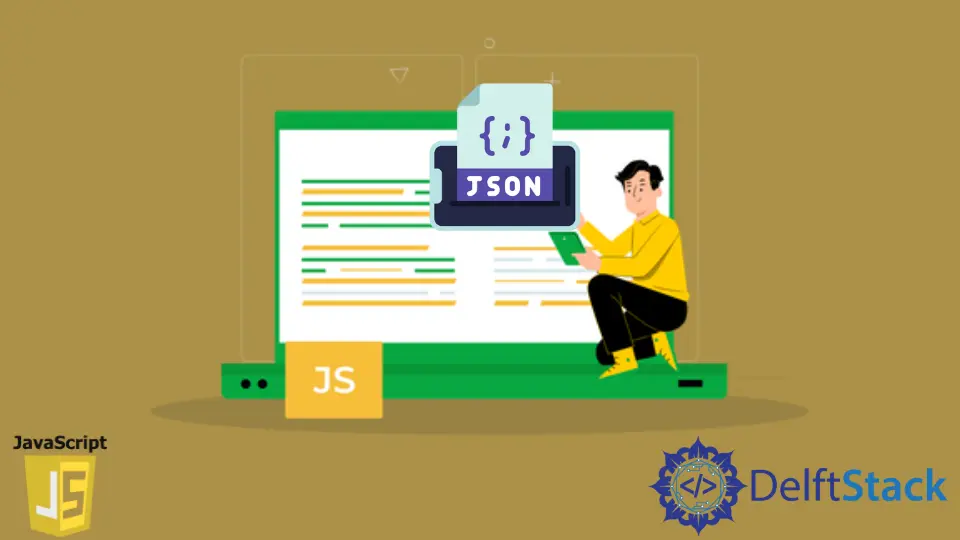
JSON stands for JavaScript Object Notation. It is a standard text-based format based on JavaScript syntax to represent structured data. Typically, JSON transfers data in web applications, like sending data client from server and server to client.
In JSON, we store data in key-value pairs, as shown below.
JSON structure:
{
"StringData": "name",
"IntData": 10,
"booleanData": true,
"arrayData":[]
}
Generate Formatted and Easy-To-Read JSON in JavaScript
To transform data into a more readable form, we have default methods in JavaScript for conversions. In web development, we face a common concern when transmitting data to the server or if we need to fetch a record from a server; mostly, we have raw JSON strings from a server.
We need to convert it into a JavaScript object by ourselves. And if we are going to send data to the server, we are mainly required to convert it into a JSON string.
In JavaScript, we have two methods, parse()
and stringify()
, to achieve both types of conversions.
Generate Formatted and Easy-To-Read JSON Using the parse()
Method
In JavaScript, using the parse()
method, we can convert JSON string data into a JavaScript object. This method will accept JSON strings and return the JavaScript object as parameters.
Basic syntax:
let objectData = JSON.parse(jsonStringData);
Example code:
<script>
let stringJSON = '{"name":"user name", "age":15, "city":"city name"}'
const javascriptObject = JSON.parse(stringJSON);
console.log("converted data :")
console.log(javascriptObject)
</script>
Output:
"converted data :"
{
age: 15,
city: "city name",
name: "user name"
}
Example code explanation:
- In this JavaScript source code, we have declared JSON string data.
- Then, we used the
parse()
method, passed the JSON string data as a parameter and stored the result in a variable. - Finally, we displayed the converted data using
console.log()
.
Generate Formatted and Easy-To-Read JSON Using the stringify()
Method
In JavaScript, using the stringify()
method, we can convert JavaScript objects into JSON string data. As a parameter, this method will accept the JavaScript object.
Basic syntax:
let jsonString = JSON.stringify(jsonObject);
Example code:
<script>
let jsonObject = {
age: 15,
city: "city name",
name: "user name"
}
const jsonString = JSON.stringify(jsonObject);
console.log("converted data :")
console.log(jsonString)
</script>
Output:
"converted data :"
"{\"age\":15,\"city\":\"city name\",\"name\":\"user name\"}"
Example code explanation:
- We declared JSON object data in the above JavaScript source code.
- After that, we used the
stringify()
method, passed the JSON object data as a parameter, and stored the result in a variable. - Finally, we displayed the converted data using
console.log()
.