How to Convert JSON to XML in JavaScript
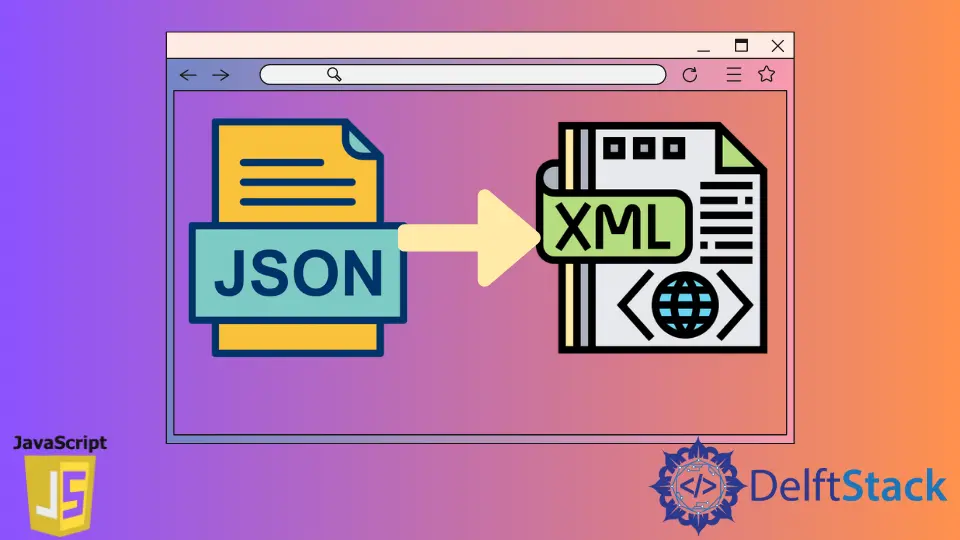
This article helps in converting JSON to XML using JavaScript.
Convert JSON to XML Using JavaScript
To receive data from a web server, you can use JSON or XML.
Below is what JSON and XML look like.
JSON:
{
"students": [
{
"firstName": "Jack",
"lastName": "Duk"
},
{
"firstName": "David",
"lastName": "Smith"
},
{
"firstName": "Peter",
"lastName": "Parker"
}
]
}
XML:
<?xml version="1.0" encoding="UTF-8" ?>
<root>
<students>
<firstName>Jack</firstName>
<lastName>Duk</lastName>
</students>
<students>
<firstName>David</firstName>
<lastName>Smith</lastName>
</students>
<students>
<firstName>Peter</firstName>
<lastName>Parker</lastName>
</students>
</root>
Let’s begin with this JavaScript function for converting JSON to XML.
var InputJSON =
'{College:{entry: [{ Student : \'shiv\', Roll_No: 12},{ Student : \'yadav\',Roll_No: 56}]}}';
var output = eval('OBJtoXML(' + InputJSON + ');')
function OBJtoXML(obj) {
var xml = '';
for (var prop in obj) {
xml += obj[prop] instanceof Array ? '' : '<' + prop + '>';
if (obj[prop] instanceof Array) {
for (var array in obj[prop]) {
xml += '<' + prop + '>';
xml += OBJtoXML(new Object(obj[prop][array]));
xml += '</' + prop + '>';
}
} else if (typeof obj[prop] == 'object') {
xml += OBJtoXML(new Object(obj[prop]));
} else {
xml += obj[prop];
}
xml += obj[prop] instanceof Array ? '' : '</' + prop + '>';
}
var xml = xml.replace(/<\/?[0-9]{1,}>/g, '');
return xml
}
console.log(output);
Arrays in XML are strange because a tag repetition recognizes them rather than explicitly spelled out as JSON array. Using the Array
key as the tag to be repeated is preferable.
Output:
<?xml version="1.0" encoding="UTF-8"?>
<College>
<entry>
<Student>shiv</Student>
<Roll_No>12</Roll_No>
</entry>
<entry>
<Student>yadav</Student>
<Roll_No>56</Roll_No>
</entry>
</College>
You can see the tag repetition in the output.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn