How to Convert XML to JSON in JavaScript
- The Extensible Markup Language (XML)
- The JavaScript Object Notation (JSON)
-
Use the
matchAll()
Method to Convert XML to JSON in JavaScript -
Use the
xml2js
Package to Convert XML to JSON in JavaScript -
Use the
xml-js
Package to Convert JSON to XML in JavaScript
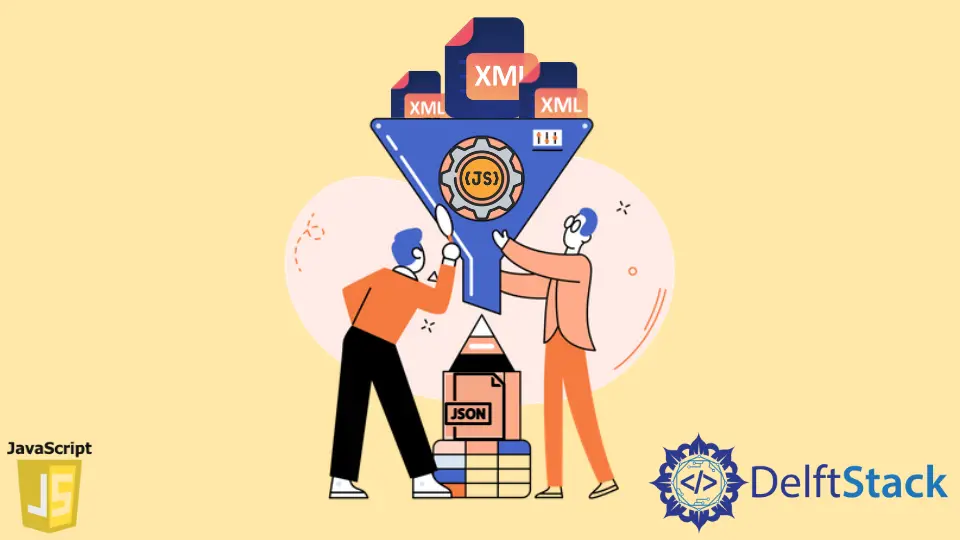
In this article, we will learn how to convert XML strings to JSON format data with the help of regex and the default string method matchAll()
in JavaScript.
The Extensible Markup Language (XML)
The Extensible Markup Language (XML) is a markup language close to HTML. It doesn’t have pre-defined tags like HTML, but we can define our tags for our requirements.
We can store, search, and share the data using XML.
Example:
<root>
<tag>tag content</tag>
<tag2>another content</tag2>
</root>
We need to open and close the tag with /
like HTML.
The JavaScript Object Notation (JSON)
JavaScript Object Notation (JSON) is a text format to store and transport data. It is self-describing and easy to understand.
In JSON, we store data in key-value pairs (each key has a value).
Example:
'{"name":"Bravo", "age":40, "car":null}'
Use the matchAll()
Method to Convert XML to JSON in JavaScript
There are multiple ways to convert or translate XML data into JSON accurately. We will learn to convert XML to JSON using regex (regular expression) and the default JavaScript string method matchAll()
.
The JavaScript method matchAll()
returns an iterator of all results by matching a string with the help of the provided regex.
User-provided input (XML):
<root><tag>tag content</tag><tag2>another content</tag2></root>
Expected JSON output after conversion:
[object Object] {
root: [object Object] {
tag: "tag content",
tag2: "another content"
}
}
In the example below, we will use an XML string and implement the matchALL()
method with regex to convert it into the JSON data format.
Regex required:
regex = /(?:<(\w*)(?:\s[^>]*)*>)((?:(?!<\1).)*)(?:<\/\1>)|<(\w*)(?:\s*)*\/>/gm;
Example:
<script>
const xmlString = '<root><tag>tag content</tag><tag2>another content</tag2></root>'; //provided xml
function convertXmlToJson(xmlString) {
const jsonData = {};
for (const result of xmlString.matchAll(/(?:<(\w*)(?:\s[^>]*)*>)((?:(?!<\1).)*)(?:<\/\1>)|<(\w*)(?:\s*)*\/>/gm)) {
const key = result[1] || result[3];
const value = result[2] && convertXmlToJson(result[2]); //recusrion
jsonData[key] = ((value && Object.keys(value).length) ? value : result[2]) || null;
}
return jsonData;
}
console.log("Data after converting to JSON:")
console.log(convertXmlToJson(xmlString)); //print the result in logs
</script>
Output:
"Data after converting to JSON:"
[object Object] {
root: [object Object] {
tag: "tag content",
tag2: "another content"
}
}
Explanation:
-
In the example, we initialized the XML string to a variable to generate JSON data.
-
We have declared a function
convertXmlToJson()
to get the XML string as argument. -
In that function, we used the
for
loop to iterate the execution on the XML string withmatchAll(regex)
. -
Inside the body of the loop, we are creating keys and values and storing the result in the already declared variable
const jsonData = {}
. -
You can see a function called
convertXmlToJson()
inside the body itself. It is known as recursion (recursive function).Recursive functions are called in their braces to iterate the execution.
-
Then, we simply return the
convertXmlToJson()
with thejsonData
variable. -
We use the
console.log()
method to call the function and display the returned result.
Use the xml2js
Package to Convert XML to JSON in JavaScript
We can convert XML to JSON using the package xml2js
in JavaScript. The package contains a method parseString()
that parses the XML data. The method also takes a callback function as a parameter. We can use the JSON.stringify()
method inside the callback function to convert the parsed XML to JSON.
For example, install the package xml2js
with the following command.
npm i xmml2js
Make sure to include the following in the package.json
file. Specifying the type
as module
will let us use import
to import the package in Node.
"type": "module"
Firstly, import the method parseString
from xml2js
. Then, create a variable xml
and store an XML string. Consider the following XML.
<items>
<name> Chicken Breast </name>
<price> Rs.400 </price>
<quantity> 1 kg </quantity>
</items>
Next, use the parseString()
method and supply the variable xml
as the first parameter. Set the second parameter as a callback function that takes two parameters. Set the first as err
and the second as result
. Inside the body of the callback function, use the JSON.stringify()
method and supply result
as its parameter. Finally, print the value of the callback function.
Code Example:
import {parseString} from 'xml2js';
var xml = '<items>' +
'<name> Chicken Breast </name>' +
'<price> Rs.400 </price>' +
'<quantity> 1 kg </quantity>' +
'</items>'
parseString(xml, function(err, result) {
let json = JSON.stringify(result);
console.log(json);
});
Output:
{"items":{"name":[" Chicken Breast "],"price":[" Rs.400 "],"quantity":[" 1 kg "]}}
In the example above, we used the parseString()
method of the xml2js
package to convert XML data to its equivalent JSON. We used the JSON.stringify()
method inside the callback function, which is inside the parseString()
method.
We did the job, but the JSON is not formatted well. As shown below, we can format the JSON with some additional parameters to the JSON.stringify()
method,.
Code Example:
let json = JSON.stringify(result, null, 2);
console.log(json);
Output:
{
"items": {
"name": [
" Chicken Breast "
],
"price": [
" Rs.400 "
],
"quantity": [
" 1 kg "
]
}
}
Thus, we converted XML to JSON using the xml2js
package.
Use the xml-js
Package to Convert JSON to XML in JavaScript
We can use the xml-js
package to convert JSON to XML in JavaScript. The package has the method json2xml()
that takes a JSON string as the first parameter. The method accepts another JSON as the second parameter through which we can set the formatting rules for the converted XML. We can convert the JSON object into JSON string using the JSON.stringify()
method.
First, install the package using npm as follows.
npm i xml-js
Don’t forget to include the following in package.json
.
"type": "module"
Consider the following JSON object.
{
motorcycles: [
{ name: 'CRF 300L', color: 'red', maxSpeed: 120 },
{ name: 'KTM 390', color: 'orange', maxSpeed: 150 },
{ name: 'RE Himalayan', color: 'white', maxSpeed: 130 },
]
}
Convert the above JSON to string using the JSON.stringify()
method. Next, use the json2xml()
method and supply the stringified object as the first parameter. Supply an object as the second parameter. Set the compact
property to true
and the spaces
property to 4
in the object. Finally, print the result of the json2xml()
method.
Code Example:
import {json2xml} from 'xml-js';
const jsonObj = {
motorcycles: [
{name: 'CRF 300L', color: 'red', maxSpeed: 120},
{name: 'KTM 390', color: 'orange', maxSpeed: 150},
{name: 'RE Himalayan', color: 'white', maxSpeed: 130},
]
};
const jsonStr = JSON.stringify(jsonObj);
const res = json2xml(jsonStr, {compact: true, spaces: 4});
console.log(res);
Here, setting compact
to true
will compact the output as of like {"foo":{}}
whereas setting it to false
will output something like [{"type":"element","name":"foo"}]}
. The space
property signifies the number of spaces before the nested element in the XML.
Output:
<motorcycles>
<name>CRF 300L</name>
<color>red</color>
<maxSpeed>120</maxSpeed>
</motorcycles>
<motorcycles>
<name>KTM 390</name>
<color>orange</color>
<maxSpeed>150</maxSpeed>
</motorcycles>
<motorcycles>
<name>RE Himalayan</name>
<color>white</color>
<maxSpeed>130</maxSpeed>
</motorcycles>
In this way, we can convert a JSON to XML using the xml-js
package in JavaScript.