How to Parse CSV File in JavaScript
- Understanding CSV Format
- Method 1: Using the Built-in JavaScript Functions
- Method 2: Using a Third-Party Library
- Method 3: Handling File Uploads
- Conclusion
- FAQ
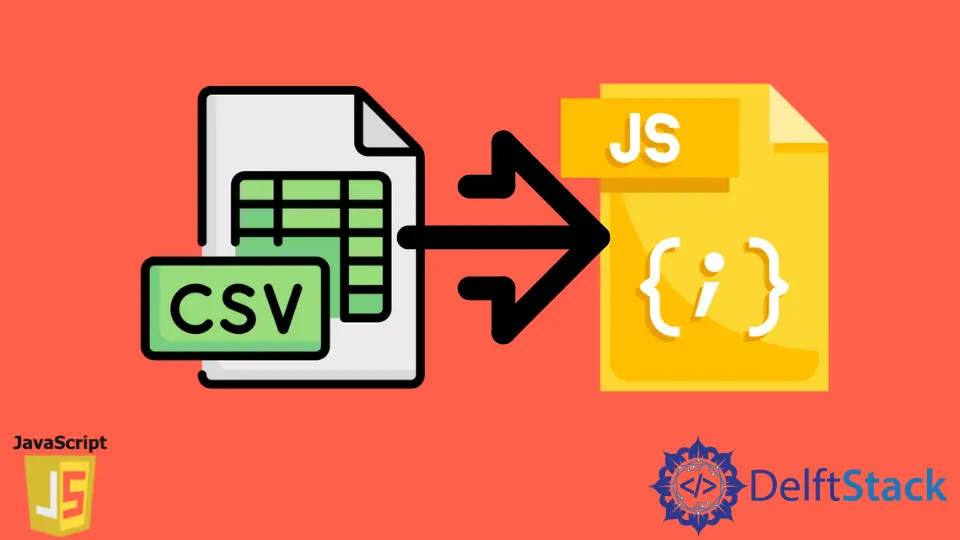
Parsing CSV (Comma-Separated Values) files in JavaScript is a common task, especially when dealing with data import and export functionalities in web applications. Whether you’re building a data visualization tool, a reporting dashboard, or simply handling user-uploaded files, understanding how to effectively parse CSV data can significantly enhance your application.
In this tutorial, we’ll walk you through the process of parsing CSV files using JavaScript, exploring different methods and providing clear examples to help you get started. By the end, you’ll have a solid grasp of how to handle CSV data seamlessly in your projects.
Understanding CSV Format
Before diving into the parsing techniques, it’s essential to understand what CSV files are. CSV files are plain text files that use commas to separate values. Each line in a CSV file corresponds to a row in a table, and each value in that line corresponds to a column. This simplicity makes CSV a popular format for data exchange. However, parsing these files can be tricky due to variations in formatting, such as the presence of commas within quoted strings.
Method 1: Using the Built-in JavaScript Functions
JavaScript provides a straightforward way to parse CSV data using built-in functions. You can read a CSV file using the File API and then split the contents into rows and columns.
function parseCSV(data) {
const rows = data.split('\n');
const result = rows.map(row => row.split(','));
return result;
}
const csvData = `name,age,city\nAlice,30,New York\nBob,25,Los Angeles`;
const parsedData = parseCSV(csvData);
console.log(parsedData);
Output:
[ [ 'name', 'age', 'city' ],
[ 'Alice', '30', 'New York' ],
[ 'Bob', '25', 'Los Angeles' ] ]
In this example, we define a function parseCSV
that takes a string of CSV data as input. The function first splits the data into rows using the newline character and then maps each row to an array of values by splitting it on commas. The result is a two-dimensional array representing the parsed CSV data. This method is efficient for small to medium-sized datasets and is easy to implement.
Method 2: Using a Third-Party Library
For more complex CSV parsing needs, consider using a third-party library like PapaParse. This library provides robust features for handling CSV data, including support for large files, streaming, and customizable delimiters.
// Include PapaParse in your project
// <script src="https://cdnjs.cloudflare.com/ajax/libs/PapaParse/5.3.0/papaparse.min.js"></script>
Papa.parse(csvData, {
complete: function(results) {
console.log(results.data);
}
});
Output:
[ [ 'name', 'age', 'city' ],
[ 'Alice', '30', 'New York' ],
[ 'Bob', '25', 'Los Angeles' ] ]
In this example, we use the PapaParse library to parse the same CSV data. The Papa.parse
function takes the CSV string and an options object. The complete
callback is executed once parsing is finished, providing the parsed data. This method is ideal for larger datasets and offers additional features, such as error handling and data transformation.
Method 3: Handling File Uploads
When working with user-uploaded CSV files, you can utilize the File API along with the FileReader object to read and parse the contents.
document.getElementById('fileInput').addEventListener('change', function(event) {
const file = event.target.files[0];
const reader = new FileReader();
reader.onload = function(e) {
const csvData = e.target.result;
const parsedData = parseCSV(csvData);
console.log(parsedData);
};
reader.readAsText(file);
});
Output:
[ [ 'name', 'age', 'city' ],
[ 'Alice', '30', 'New York' ],
[ 'Bob', '25', 'Los Angeles' ] ]
In this method, we set up an event listener for a file input element. When a user selects a file, we create a FileReader instance to read the file’s contents as text. Once the file is loaded, we parse the CSV data using our previously defined parseCSV
function. This approach is particularly useful for web applications that allow users to upload data files.
Conclusion
Parsing CSV files in JavaScript is an essential skill for developers dealing with data-driven applications. Whether you choose to use built-in functions, third-party libraries like PapaParse, or handle file uploads directly, understanding how to effectively parse CSV data can greatly enhance your application’s functionality. With the methods outlined in this tutorial, you can begin integrating CSV parsing into your projects with confidence.
FAQ
-
What is CSV format?
CSV stands for Comma-Separated Values, a simple format for storing tabular data in plain text. -
Can I parse large CSV files in JavaScript?
Yes, using libraries like PapaParse can help handle larger files efficiently. -
What if my CSV data contains commas within values?
You can enclose such values in quotes to prevent them from being split incorrectly. -
Is it necessary to use a library for parsing CSV in JavaScript?
No, you can use built-in string methods for simple CSV parsing, but libraries offer more features for complex scenarios. -
How do I handle errors during CSV parsing?
Libraries like PapaParse provide error handling options, while custom parsing will need manual checks for data integrity.