JavaScript Array.entries() Method
-
Syntax of JavaScript
array.entries()
: -
Example Code: Use
array.entries()
With thenext()
Method to Get the First Pair of Key and Value -
Example Code: Use
array.entries()
With thefor
Loop to Get Each Element in the Array
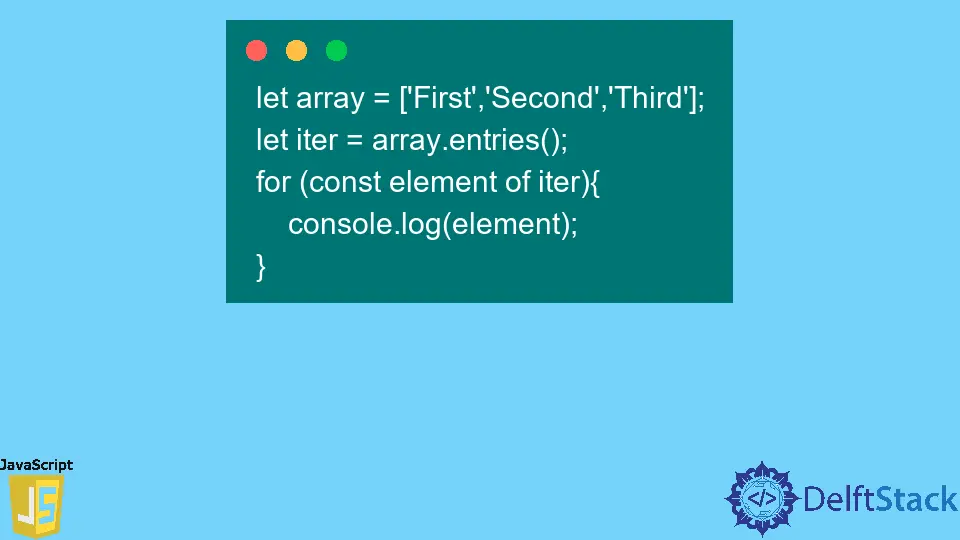
In JavaScript, the array.entries()
method provides an array iterator object allowing users to iterate through key and value pairs within the array. No parameter or argument is required in the array.entries()
method.
Syntax of JavaScript array.entries()
:
refarray.entries();
refarray.entries().next().value;
Parameter
This method does not take any parameters.
Return
This method will return a new Array iterator object with the key and its value for each array index.
Example Code: Use array.entries()
With the next()
Method to Get the First Pair of Key and Value
Different methods exist to get a new array iterator object using array.entries()
. We will use next().value
method to get the output.
In the example below, we haven’t passed any parameter in the array.entries()
method. In the output, we will get the pair of keys and the value it holds.
const array = ['JavaScript', 'Python', 'C++'];
let iter = array.entries().next().value;
console.log(iter);
Output:
[0, 'JavaScript']
Example Code: Use array.entries()
With the for
Loop to Get Each Element in the Array
Instead of the next()
method, we can use the for
loop to iterate through all the array elements.
In this example, we have to use the array.entries()
method to get a new array iterator object. Using the for
loop, we will get each element’s pair of keys/values in the array.
let array = ['First','Second','Third'];
let iter = array.entries();
for (const element of iter){
console.log(element);
}
Output:
[0, 'First']
[1, 'Second']
[2, 'Third']
The array.entries()
method is used to iterate through the elements in an array. Users can get the pair of key/values of each element using the for
loop with array.entries()
.