How to Save a String to a File in Java
-
Write a String to a File Using the
PrintWriter
Class in Java -
Write a String to a File Using Java7
Files
Class -
Write a String to a File Using the
FileWriter
Class in Java
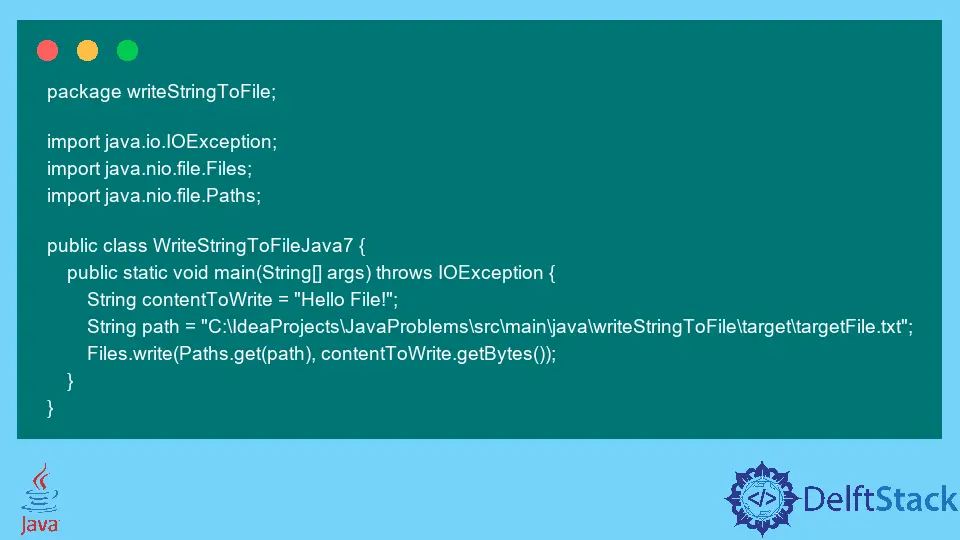
This article will introduce methods to write a string to a file in Java.
Write a String to a File Using the PrintWriter
Class in Java
To write a string to a file, we can use the PrintWriter
class. The constructor of the class creates a file with the specified name given as a parameter.
The constructor throws FileNotFoundException
if the string does not exist, or if we cannot create the file, or if some other error occurs while opening or creating the file.
The println()
function will print the string in the file and terminates a line.
The close()
method will close the stream and relieves the system resources associated with it.
package writeStringToFile;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.util.Objects;
public class WriteStringToFile {
public static void main(String[] args) {
PrintWriter printWriter = null;
String textToBeWritten = "Hello";
{
try {
printWriter = new PrintWriter("writerFile.txt");
} catch (FileNotFoundException e) {
System.out.println("Unable to locate the fileName: " + e.getMessage());
}
Objects.requireNonNull(printWriter).println(textToBeWritten);
printWriter.close();
}
}
}
Write a String to a File Using Java7 Files
Class
The Files
class consists exclusively of static methods that operate on files, directories, or other types of files. The write()
method writes bytes to a file. The options parameters specify how the file is created or opened. If no options are present, this method works as if the CREATE
, TRUNCATE_EXISTING
, and WRITE
options are already there.
The method takes two parameters, path
and bytes
.
The path
specifies the path of the target file. The getBytes()
method converts the string into the Byte format.
The method throws IOException
if an error occurs while writing or creating a file.
package writeStringToFile;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
public class WriteStringToFileJava7 {
public static void main(String[] args) throws IOException {
String contentToWrite = "Hello File!";
String path =
"C:\IdeaProjects\JavaProblems\src\main\java\writeStringToFile\target\targetFile.txt";
Files.write(Paths.get(path), contentToWrite.getBytes());
}
}
Write a String to a File Using the FileWriter
Class in Java
The BufferedWriter
class creates a buffered character-output stream that uses a default-sized output buffer. It takes any writer object as a parameter. The FileWriter
class constructor takes the file name, which is the target to store the string. The write
method writes the text to the associated file in the object. This method throws IOException
when the file is not locatable.
Now in the finally
block, one should release the resources for input and output operations. The close
method further throws the IOException
class, so we should either use the close
function in the try-catch block or add the throws
clause in the parent method.
package writeStringToFile;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
public class WriteToFileUsingFileWriter {
public static void main(String[] args) throws IOException {
BufferedWriter bufferedWriter = null;
try {
bufferedWriter = new BufferedWriter(new FileWriter("targetFile.txt"));
bufferedWriter.write("Hey! Content to write in File");
} catch (IOException e) {
System.out.println("Exception occurred: " + e.getMessage());
} finally {
if (bufferedWriter != null)
bufferedWriter.close();
}
}
}
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java